While doing folder based operation in PowerShell script file, it’s very common practice to check if directory exists and create directory if not exists.
If folder doesn’t exist then it may throw exceptions in the script while trying to access folder files, delete folders, create new files in folders, or any kind of folder based operation.
In PowerShell, there are different ways to create directory if not exists and check directory exists or not before doing any directory-based operation to avoid exceptions.
In this article, I will explain to you how to create directory if not exists with examples using PowerShell.
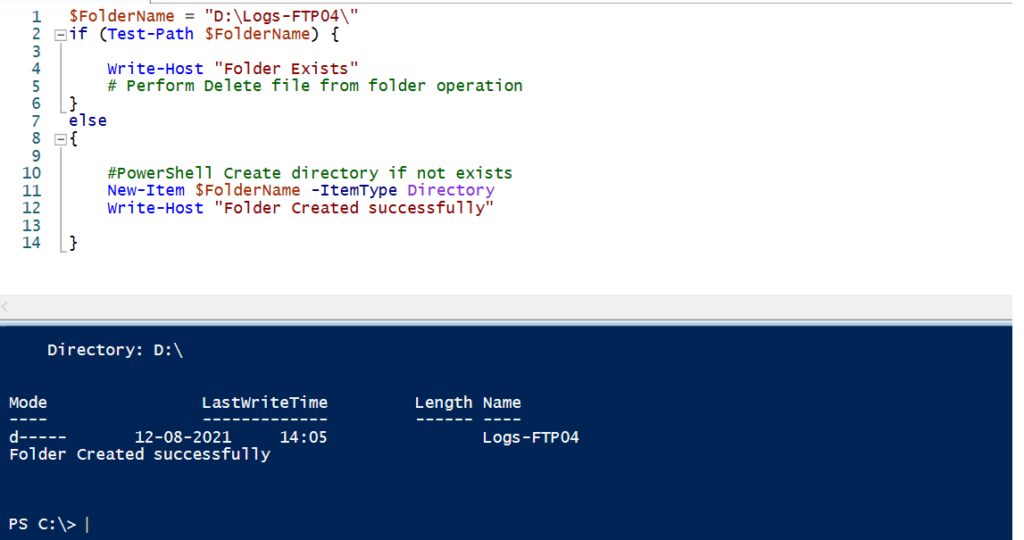
There are four different ways to check if folder exists and create directory as below
- Test-Path
- Get-Item
- Get-ChildItem
- [System.IO.File]::Exists(directory)
Let’s understand each of the methods to check if a folder exists or not to create a folder.
PowerShell Create Directory If Not Exists using Test-Path
PowerShell Test-Path
cmdlet check if directory exists or not.
If a directory exists then it will return $True. If a path or directory is missing or doesn’t exist, it will return $False.
Using PowerShell New-Item cmdlet, it will create directory if not exists using Test-Path.
Test-Path cmdlet Syntax
Test-Path -Path <FolderPath>
In the above syntax,
FolderPath – folder location
Test-Path Example
Let’s consider an example to understand how to use PowerShell Test-Path cmdlet to check and create directory if not exists.
We want to create the Log-FTP03 directory in D: drive.
Run the below code to understand the Test-Path cmdlet
$FolderName = "D:\Logs-FTP03\" if (Test-Path $FolderName) { Write-Host "Folder Exists" # Perform Delete file from folder operation } else { #PowerShell Create directory if not exists New-Item $FolderName -ItemType Directory Write-Host "Folder Created successfully" }
In the above PowerShell script,
$FolderName variable contains directory path
Using Test-Path cmdlet, it first checks if folder exists on location.
If the folder exists then “Folder Exists” message on the console and perform any operation written inside if statement like delete the file from folder or etc…
If the folder doesn’t exists then print a message on the console as “Folder Doesn’t Exists”
Using the New-Item cmdlet, it create directory with the path specified and ItemType
parameter specified as Directory.
PowerShell Create Directory if Not Exists using Get-Item
PowerShell Get-Item
cmdlet used to get items at a specified location.
You can use the wildcard character (*) to get all the contents of the item.
We will see how to use the Get-Item cmdlet in PowerShell to create directory if not exists.
Get-Item Syntax
Get-Item [-Path] <String[]> [-Filter <String>] [-Include <String[]>] [-Exclude <String[]>] [-Force] [-Credential <PSCredential>] [-Stream <String[]>] [<CommonParameters>]
In the above Get-Item syntax, you can use different parameters to get specific items.
Let’s understand, using Get-Item cmdlet to check directory exists or not in PowerShell.
Get-Item Example
Let’s consider an example to create directory with the name Log-FTP03 in D drive.
Use PowerShell Get-Item cmdlet to check if folder exists or not and create directory if not exists.
$FolderName = "D:\Logs-FTP03\" $FileName = "D:\Logs-FTP03\ftp-103.txt" if(Get-Item -Path $FolderName -ErrorAction Ignore) { Write-Host "Folder Exists" #Create new file if(Get-Item -Path $FileName -ErrorAction Ignore){ Write-Host "File already exists" } else { New-Item -Verbose $FileName -ItemType File } } else { Write-Host "Folder Doesn't Exists" #PowerShell Create directory if not exists New-Item $FolderName -ItemType Directory }
In the above PowerShell script,
$FileName variable contains new filename path.
$FolderName variable contains new folder name path
Using the Get-Item cmdlet, it first checks if folder exists on location.
If a folder exists then it checks if file exists or not to create new file using New-Item a cmdlet.
It will create directory if a folder does not exist with New-Item cmdlet having ItemType parameter as Directory.
Cool Tip: How to get file creation date in PowerShell!
Get-ChildItem to Create Directory If Not Exists
PowerShell Get-ChildItem cmdlet is used to get items or child items from one or more specified locations.
We will see with examples, how to use the Get-ChildItem cmdlet to create folder if not exists.
Get-ChildItem Syntax
Get-ChildItem [[-Path] <string[]>] [[-Filter] <string>] [-Include <string[]>] [-Exclude <string[]>] [-Recurse] [-Depth <uint32>] [-Force] [-Name] [-Attributes <FlagsExpression[FileAttributes]>] [-FollowSymlink] [-Directory] [-File] [-Hidden] [-ReadOnly] [-System] [<CommonParameters>]
In the above Get-ChildItem syntax, you can use different parameters to get items from one or more specific locations specified by the Path parameter.
Let’s understand, using Get-ChildItem cmdlet to check folder exists in PowerShell.
Get-ChildItem Example
Let’s consider an example to create new directory Logs-FTP01 on D drive.
Using the Get-ChildItem cmdlet, it will check if folder exists or not, run the below script
$FolderName = "D:\Logs-FTP01\" if(Get-ChildItem -Path $FolderName -ErrorAction Ignore) { Write-Host "Folder Exists" #Perform Folder based operation, Count files in folder (Get-ChildItem -Path $FolderName -File | Measure-Object).Count } else { Write-Host "Folder Doesn't Exists" #PowerShell Create directory if not exists New-Item $FolderName -ItemType Directory }
In the above PowerShell script,
$FolderName variable contains a new directory name
Using the Get-ChildItem cmdlet, it first checks if folder exists at the location.
If the folder exists then it will count files in folder.
If the folder doesn’t exist then print a message on the console as “Folder Doesn’t Exists”.
It will create directory if not exists using New-Item cmdlet with folder name as path specified.
–ErrorAction ignore parameter ignore the exception raised while checking folder exists operation.
Cool Tip: How to find large size files in PowerShell!
Using [System.IO.Directory]::Exists() Method
Using the .Net class System.IO.Directory and Exists() method, we can check if directory exists or not before doing any operation.
Let’s understand using [System.IO.Directory]::Exists() method with example.
For example, if you want to create a new directory at the specified location.
Before you create new directory, you first check if the folder exists or not to avoid errors.
[System.IO.Directory]:: Exists() method takes folder name as input and returns True or False depending on folder exists or not.
$FolderName = "D:\Logs-FTP03\" if([System.IO.Directory]::Exists($FolderName)) { Write-Host "Folder Exists" Get-ChildItem -Path $FolderName | Where-Object {$_.CreationTime -gt (Get-Date).Date} } else { Write-Host "Folder Doesn't Exists" #PowerShell Create directory if not exists New-Item $FolderName -ItemType Directory }
In the above PowerShell script,
$FolderName – contains foldername path
Using [System.IO.Directory]::Exists() method to check if folder exists. It returns true if the file exists else returns false.
If the folder exists then Get-ChildItem will get list of files created today from the directory.
It will create directory if the folder doesn’t exist using the New-Item cmdlet as folder name path specified.
Cool Tip: How to list files in the directory in PowerShell!
Conclusion
In the above article, I have explained four different methods to create directory if directory not exists using PowerShell way.
These methods are Test-Path, Get-Item, Get-ChildItem, and [System.IO.Directory]:: Exists().
It’s good practice to check if a directory exists before you perform any folder-based operation to avoid errors.
Using directory exists check, you can handle errors and conditions to do folder operation.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.