Function, in general, contains code to perform certain tasks and return a value. Functions are mostly used to reuse the functionality.
In PowerShell function contains one-liner or script to perform a task, we can handle functionality in function by passing multiple parameters to function in PowerShell.
In this article, we will discuss how to pass multiple parameters to a function in PowerShell with examples.
PowerShell β Pass Multiple Parameters to Function
In the PowerShell function, we can pass multiple parameters sets with different data types. These parameters may be mandatory or optional.
We will see for example to create folder and file at a specified location using the PowerShell function.
Problem Statement: Create a function to create directory if not exists.
The function should accept multiple parameters as mandatory.
In the function, it should check if a folder exists or not and create directory using the Foldername parameter specified.
The function should check if a file exists and create a new file if not using provided filename parameter.
Function Name β Create-Directory
Multiple Parameters:
FolderName β string data type, contains folder name path
FileName β string data type, mandatory parameter, contains file name path
TempFile β string data type, optional parameter, contains file name path
Solution:
Refer below PowerShell script for the above problem statement to pass multiple parameters to function in PowerShell.
# function to create directory and create file Function Create-Directory() { # Create multiple parameters # Mandatory parameter, set true # Optional parameter, set false # string data type and parameter name Param ( [Parameter(Mandatory = $true)] [string] $FolderName, [Parameter(Mandatory = $true)] [string] $FileName, [Parameter(Mandatory = $false)] [string] $TempFile ) try { # Check if folder exists if(Get-Item -Path $FolderName -ErrorAction Ignore) { # Check optional parameter value as by default optional parameter has null value if($TempFile) { # Check if file exists if(Get-Item -Path $TempFile -ErrorAction Ignore){ Write-Host $TempFile "file already exists!" } else { #Create temp file New-Item -Verbose $TempFile -ItemType File Write-Host -f Green $TempFile "file created successfully!" } } else { Write-Host "File name path Parameter is null/optional" } } else { #PowerShell Create directory if not exists New-Item -Verbose $FolderName -ItemType Directory Write-Host -f Green $FolderName "folder created successfully!" New-Item -Verbose $FileName -ItemType File Write-Host -f Green $FileName "file created successfully!" } } catch { $ErrorMsg = $_.Exception.Message + " error raised while creating folder!" Write-Host $ErrorMsg -BackgroundColor Red } } #Parameters - Pass multiple parameters to the function $FolderName="D:\Logs-FTP01"; $FileName= "D:\Logs-FTP01\NewFile.txt"; $TempFile= "D:\Logs-FTP01\Temp.txt"; Create-Directory $FolderName $FileName $TempFile
In the above PowerShell script, we have
$FolderName β contains folder path (mandatory parameter)
$FileName β contains file path (mandatory parameter)
$TempFile β contains temp file path (optional parameter)
We have created function Create-Directory as per PowerShell function guideline ( pascal case name with a verb and noun)
Using the Param keyword, we have created three parameters.
$Foldername
and $Filename
parameters are mandatory as we have set mandatory set to $true
.
$TempFile
parameter is having mandatory set to $false
. In PowerShell, by default, all parameters are optional.
Inside a function, we have to check if a folder exists or not using Get-Item a cmdlet.
If a folder doesnβt exist then create directory and create file.
We have used βErrorAction parameter to Ignore any error message if directory doesnβt exist.
If a folder exists, create a temporary file name inside the folder name path.
We need to check for $TempFile value as optional parameters have default null value.
We can call the functions using Function name and three parameters created above as below
#Parameters - Pass multiple parameters to the function
$FolderName="D:\Logs-FTP01";
$FileName= "D:\Logs-FTP01\NewFile.txt";
$TempFile= "D:\Logs-FTP01\Temp.txt";
Create-Directory $FolderName $FileName $TempFile
Using the above method, we can create a function in PowerShell and pass multiple parameters sets to function in PowerShell.
The output of the above script as below
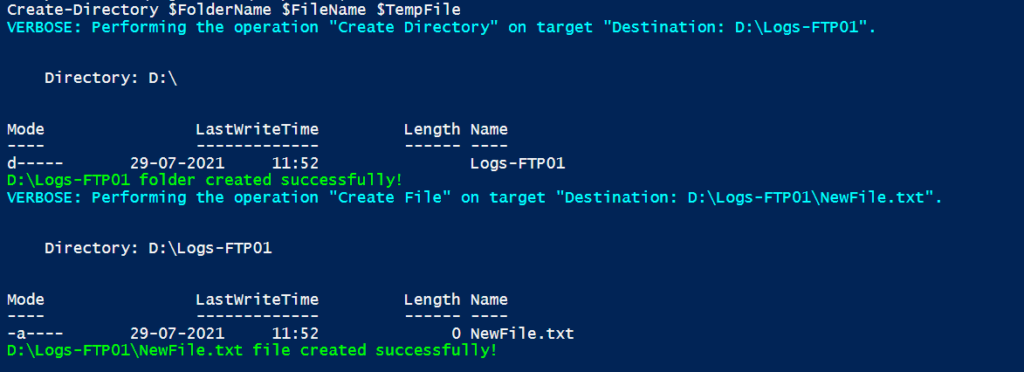
Cool Tip: How to get parameters list for cmdlet in PowerShell!
Conclusion
I hope the above-detailed article on how to pass multiple parameters to function using PowerShell is helpful and educational to you.
By default parameters in the PowerShell function are optional. To make it mandatory parameters, use Mandatory and set it $true.
Optional parameters have default null values.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.