If you have prior software development experience, for exception handling we generally use PowerShell Try-Catch-Finally block. PowerShell also supports try-catch-finally block to handle or respond to terminating errors in the PowerShell script.
In PowerShell 2.0, common parameters related to error handling available are: -ErrorAction and -ErrorVariable.
The ErrorAction parameter specifies how PowerShell handles the error during the execution of a script.
The ErrorVariable parameter specifies a variable that will be used to store the error message.
In this blog post, we will discuss -ErrorAction and -ErrorVariable parameters in PowerShell with examples.
PowerShell ErrorAction Parameter
The PowerShell ErrorAction parameter allows you to specify how to respond or handle terminating errors or actions to take if a command fails.
ErrorAction parameter has below options available to handle the execution of command if an error raised
- Continue – The script will continue to execute even if an error occurs.
- Ignore – The script will ignore the error and continue executing the script.
- Inquire – The script will stop executing and prompt the user for further instructions if an error occurs.
- SilentlyContinue – The script will continue to execute if an error occurs, but the error message will not be displayed.
- Stop – The script will stop executing if an error occurs.
- Suspend – The script will suspend the script and wait for the user to press a key before continuing.
Let’s understand each of the ErrorAction PowerShell options with examples
-ErrorAction Continue
ErrorAction PowerShell parameter basically overrides the $ErrorActionPreference in Windows PowerShell.
$ErrorActionPreference variable responds to which action to take in case of error is raised.
using PowerShell ErrorAction parameter, its default uses continue preference. It means that in the event of an error raised, its output will be written to the host and the script will be able to continue.
Let’s consider an example to stop the process RabbitMQ and handle any error produced using the ErrorAction PowerShell parameter.
PS C:\> Stop-Process -Name RabbitMQ -ErrorAction Continue; Write-Host "Execute second command";
In the above example, the Stop-Process
cmdlet takes the RabbitMQ process name and tries to stop it. As it cannot find a process with a given name, it throws an exception.
Error raised is output to host and using –ErrorAction continue option, it continues with execution for the second command.
It converts terminating errors to non-terminating errors to continue with execution and write output to the host as given below.
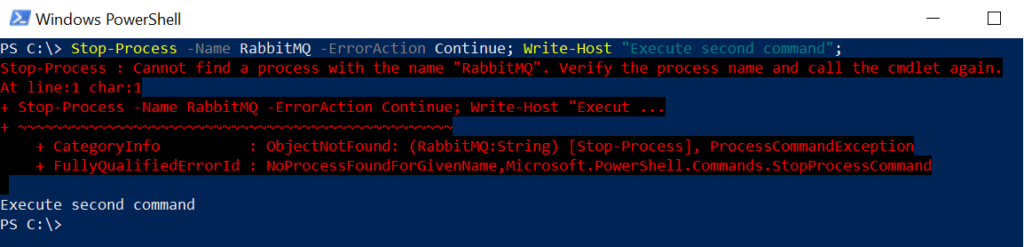
Do you know: How to use the cat command in Windows!
PowerShell -ErrorAction Ignore
The PowerShell ErrorAction Ignore option does not produce any error message write any error output on the host and continue with execution.
Using -ErrorAction Ignore
option no errors will be updated in PowerShell $Error variable (automatic variable).
Let’s consider the same example to stop the RabbitMQ process. We will use the ErrorAction Ignore option to ignore any error message and continue with the execution of the second command.
PS C:\> Stop-Process -Name RabbitMQ -ErrorAction Ignore; Write-Host "Execute second command";
The output of the above command is as below.
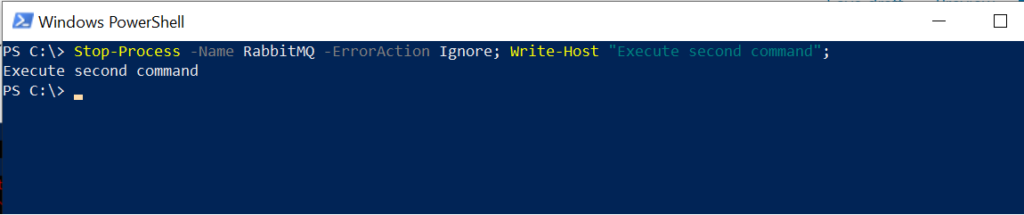
PowerShell Tip: How to add a new line to a string or variable?
-ErrorAction Inquire
The PowerShell ErrorAction Inquire option produces an error and prompts the user to confirm actions to take:
a. Yes b. Yes to All c. Halt Command d. Suspend
Based on the user’s action, it decides to continue with execution or halt or suspend it.
Let’s consider the above example to stop the RabbitMQ process using Stop-Process. We will use -the ErrorAction Inquire option to let the user confirm action in the case of an error produced
PS C:\>> Stop-Process -Name RabbitMQ -ErrorAction Inquire; Write-Host "Execute second command";
Output of the above command with user selection as Yes
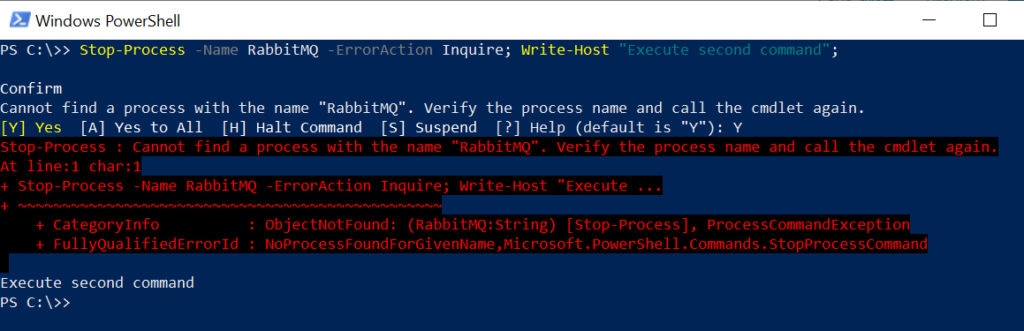
If the user selects Halt Command, it will write an error message on the Host and halt further execution. If the user selects the Suspend option, it will suspend the current execution.
Cool Tip: Replace text in a string using PowerShell!
PowerShell -ErrorAction SilentlyContinue
–ErrroAction SilentlyContinue in PowerShell silently continues with the execution of code if the part of the code does not work or has non-terminating errors.
PowerShell ErrorAction SilentlyContinue
option silence non-terminating errors and continue execution. The error will updated in the PowerShell Error variable (automatic variable).
Let’s consider an example of to stop process named “RabbitMQ” using the Stop-Process
. We will use -the ErrorAction SilentlyContinue option to silence non-terminating errors and execution of the second command.
PS C:\>>> Stop-Process -Name RabbitMQ -ErrorAction SilentlyContinue; Write-Host "Execute second command";
The output of the above command is as below.

Cool Tip: Get-ChildItem cmdlet – Search for files in PowerShell!
-ErrorAction Stop
The PowerShell ErrorAction Stop option displays an error message on the host and stops further execution of code. It terminates the code.
Let’s consider a similar example given above to find the process name “RabbitMQ“. We will use the -ErrorAction Stop option to check execution.
PS C:\>>> Stop-Process -Name RabbitMQ -ErrorAction Stop; Write-Host "Execute second command";
The output of the above command is as below.
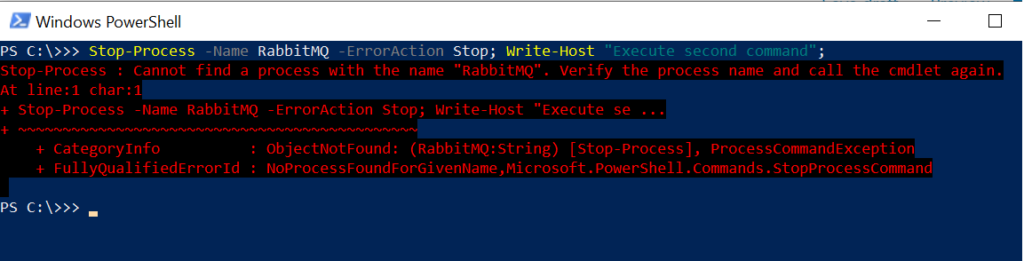
-ErrorAction Suspend
PowerShell -ErrorAction Suspend option available in PowerShell workflows. If an error is raised in the command, workflows will get suspended.
Cool Tip: The best way to use the PowerShell Get-ChildItem cmdlet!
PowerShell ErrorVariable Parameter
When you run a PowerShell command, in the event of an error raised, an error will added to the PowerShell $Error automatic variable.
Using the PowerShell ErrorVariable parameter, you can specify your own variable name to store the error message.
PowerShell ErrorVariable will overwrite the error message value to the variable name. If you want to append an error in a variable name, use + in front of the variable name. It will append an error message.
Let’s consider an example to illustrate -ErrroVariable in PowerShell. We will use the same example given above to stop the process named RabbitMQ. As the command is not able to find the process, it will raise an error.
Using -ErrorVariable PowerShell, we will store an error message in processerror variable name as given below
PS C:\>>> Stop-Process -Name RabbitMQ -ErrorVariable processError;
In the above command, it will add error message on PowerShell Error variable as well as on to processvariable variable.
If we print processError variable name, it will write error output on host as given below
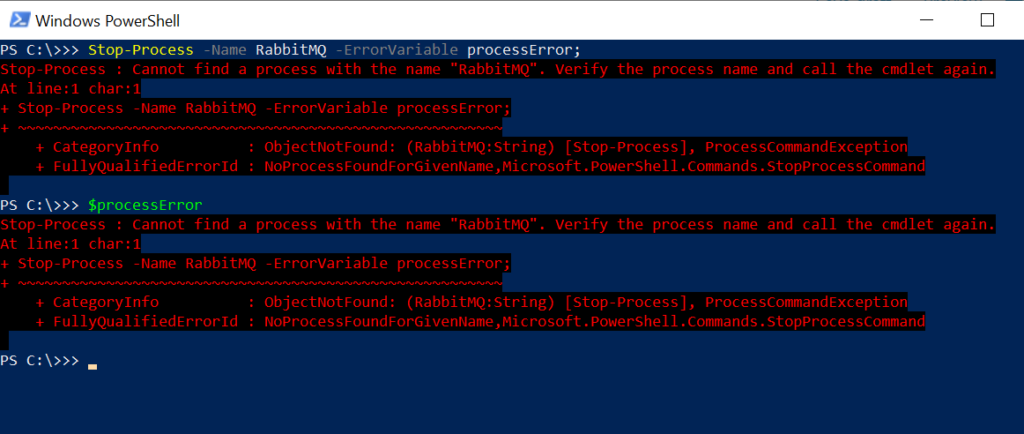
Cool Tip: Get-FileHash cmdlet – Get MD5 checksum in PowerShell!
You can make the use of -ErrorAction and -ErrorVariable together to silent non-terminating errors and add error message in variable to take further action
Using the same example given above with -ErrorAction SilentlyContinue option and -ErrorVariable to store error message in processError
variable.
PS C:\>>> Stop-Process -Name RabbitMQ -ErrorAction SilentlyContinue -ErrorVariable processError; PS C:\>>> $processError
In the above code, SilentlyContinue option in PowerShell silent non-terminating errors and -ErrorVariable parameter add error message to processError variable as given below
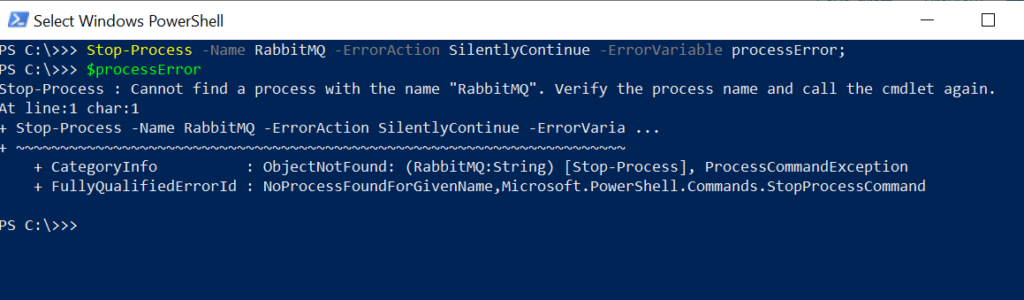
Cool Tip: Read more about PowerShell $PSScriptRoot Automatic Variable!
Conclusion
I hope you have liked the above-detailed article about the PowerShell ErrorAction parameter and ErrorVariable PowerShell parameters.
Using different options available with -ErrorAction, we can handle error messages in code.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.