In one of my recent tasks, I had to deal with files and folder-based operations on a local system. File or folder-based operation is a very cumbersome process using a manual way if you have a lot many files or directories to look for.
Using PowerShell, itβs very easy to list files in a directory or list folders based on filter criteria.
PowerShell PSIsContainer to list files in a directory which has PSIsContainer property set to $false
in their file system object. Folder or Directory has PSIsContainer property set to $true
in their container.
Using PowerShell PSIsContainer, itβs very easy to get files in the directory or select folders only.
PowerShell Get-ChildItem cmdlet returns files or folders in the root directory of the file system.
In this article, I will explain using PowerShell PSIsContainer with different examples to list files in the directory, get all files in a directory and subdirectories, and list all .txt files in a folder.
PowerShell List Files in Directory
To get a list of files in a directory, use the Get-ChildItem command with a filter condition based on a file that has the PowerShell PSIsContainer property set to $false
.
Use the below command to list all files in the directory and subdirectories.
PS C:\> Get-ChildItem -Path D:\PowerShell\Excel\ -Recurse | Where-Object {$_.PSIsContainer -eq $false}
The above PowerShell script, using the PowerShell Get-ChildItem
cmdlet, returns files and folders. The Get-ChildItem
command uses the Path
parameter to specify the directory path from where we need to list files. The Recurse
parameter specifies the list of files from the directory and subdirectories recursively.
It passes its output to the Where-Object command where it checks for PowerShell PSIsContainer property is equal to false ($_.PSIsContainer -eq $false) to filter files only.
It returns list files in directory and subdirectories, it prints files with their Mode, LastWriteTime, File size, and File Name
PowerShell Tip: How to find files with lastwritetime in PowerShell!
The output of the above command to list all files in the directory and subdirectories is given below.
PS C:\> Get-ChildItem -Path D:\PowerShell\Excel\ -Recurse | Where-Object {$_.PSIsContainer -eq $false}
Directory: D:\PowerShell\Excel
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 05-10-2020 21:14 559 DownloadZipFile-Unzip.ps1
-a---- 08-10-2020 09:40 105 import-csv.ps1
-a---- 04-10-2020 17:06 199 IsModuleInstalled.ps1
-a---- 24-10-2020 20:08 598 ping-computers.ps1
-a---- 10-10-2020 19:37 139 quser.ps1
-a---- 04-10-2020 17:05 911 rename-adgroup.ps1
-a---- 04-10-2020 13:22 861 search-excel.ps1
-a---- 06-10-2020 19:06 25 throw.ps1
-a---- 06-10-2020 18:09 182 Unzip-file.ps1
PS C:\>
Cool Tip: Get-ChildItem cmdlet β Search for files in PowerShell!
List Files in a Directory using the -Exclude parameter
The β-Excludeβ parameter excludes the directories and files with some specific extension. Wildcard characters can be used with -Exclude parameter, such as β*.exeβ.
Get-ChildItem -Path D:\PS\sqlite\ -Recurse -Exclude *.exe | Where-Object {$_.PSIsContainer -eq $false}
In the above PowerShell script, the Get-ChildItem
command uses the Path
parameter that specifies the location of the directory from where we want to get all the files.
The Recurse parameter specifies that the command should recursively look into the directory and subdirectories to list all the files. The -Exclude parameter specifies the condition to exclude the files having the extension .exe and get all other files in a directory.
The output of the first command passes to the Where-Object
command which checks if the file has the PSIsContainer
property set to false and gets all files in the folder.
The output of the above PowerShell script list files in a directory and subdirectory that excludes the .exe file is given below.
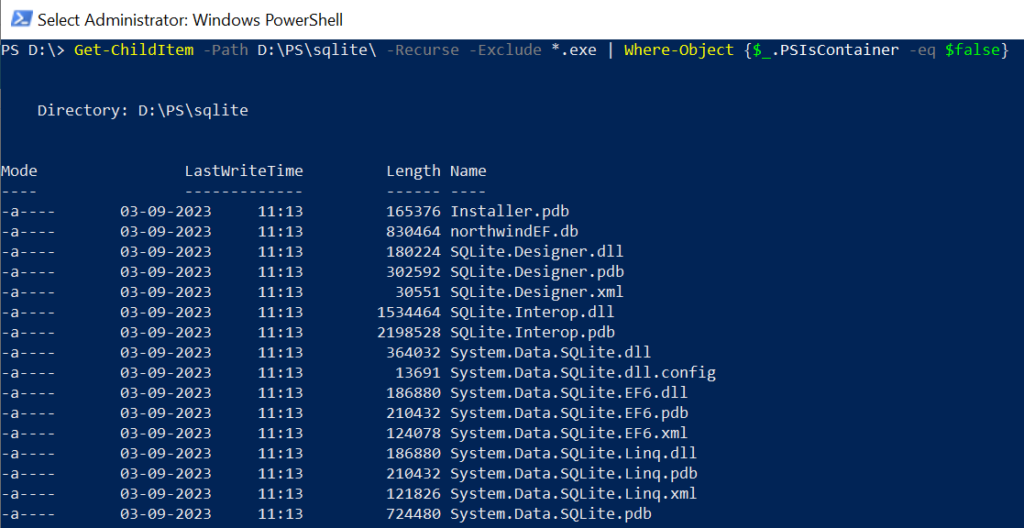
List Files in a Directory using the -Include parameter
The β-Includeβ parameter includes the directories and files with some specific extension. Wildcard characters can be used with -Include parameter, such as β*.txtβ.
Get-ChildItem -Path D:\PS\LogTest\ -Recurse -Include *.txt | Where-Object {$_.PSIsContainer -eq $false}
In the above PowerShell script, the Get-ChildItem
command uses the Path
parameter that specifies the location of the directory from where we want to get all the files.
The Recurse parameter specifies that the command should recursively look into the directory and subdirectories to list all the files. The -Include
parameter specifies the condition to exclude the files having the .txt extension and get all files in a directory.
The output of the first command passes to the Where-Object command which checks if the file has the PSIsContainer property set to false and gets all .txt files in the folder.
The output of the above PowerShell script list files in a directory and subdirectory that includes the .txt file is given below.
PS D:\> Get-ChildItem -Path D:\PS\LogTest\ -Recurse -Include *.txt | Where-Object {$_.PSIsContainer -eq $false}
Directory: D:\PS\LogTest\FTP-01
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 22-08-2021 21:55 0 Newline-FileTest - Copy.txt
-a---- 22-08-2021 21:55 0 Newline-FileTest.txt
Directory: D:\PS\LogTest\FTP-02\File-Split
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 08-06-2022 09:46 12367006 LogFile.txt
Directory: D:\PS\LogTest\FTP-02
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 25-06-2022 12:49 599 Environement-Variable.txt
PowerShell PSIsContainer to List Folders and Subfolders
PowerShell Get-ChildItem cmdlet gets files and folders only.
To get a list of folders and subfolders in the filesystem, use a filter based on a folder that has PSIsContainer
property set to $true
.
PS C:\> Get-ChildItem -Path D:\PowerShell -Recurse | Where-Object {$_.PSIsContainer}
In the above PowerShell script, Get-ChildItem
returns files, and folders recursively and passes its output to the Where-Object command.
In the second command, it checks for the folder which has the PowerShell PSIsContainer property set to $true and gets a list of folders and subfolders in the file system.
Cool Tip: To get memory usage in PowerShell!
PowerShell PSIsContainer to Get Folders with No Files
To get folders and subfolders having no files in them, use PowerShell PSIsContainer uses the property of all file system object to select folders only which has the property set to true.
Use GetFiles().Count
property to get file count.
PS C:\> Get-ChildItem -Path D:\PowerShell -Recurse | Where-Object {$_.PSIsContainer -eq $true} | Where-Object {$_.GetFiles().Count -eq 0}
In the above PowerShell script, Get-ChildItem
cmdlets return files and folders recursively and pass their output to another command.
The second command check PowerShell PSIsContainer property is true to filter only the directory and pass its output to the next command
The third command, get files count for each folder and check if it is equal to 0 and returns folders having no files.
Cool Tip: How to fix the PowerShell script is not digitally signed!
Conclusion
Use the PowerShell PSIsContainer property of the file system object to select files if the property is set to $false in their PSIsContainer property and select folder if the property is set to $true in their PSIsContainer property.
Using PowerShell Get-ChildItem
cmdlet and PSIsContainer
to list files in the directory or list all files in the directory and subdirectories.
Cool Tip: How to list files sorted by date in PowerShell!
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.