The Get-Date cmdlet in PowerShell gets the current date and time of the system. Get-Date uses the computer’s culture settings to format the output date and can format date and time in different .Net and Unix formats.
Get-Date can be used to generate a date or time character string to pipe input to other cmdlets.
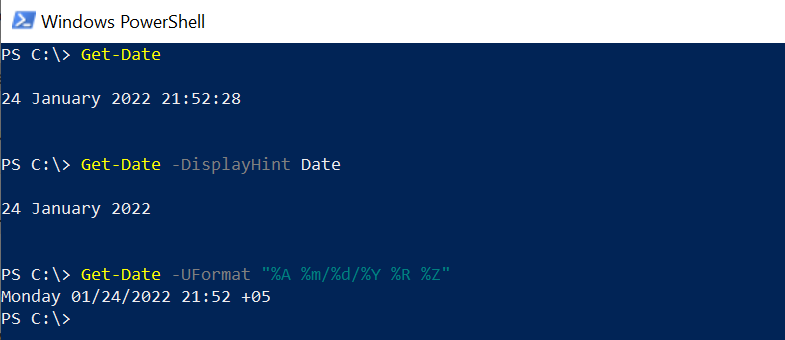
The Get-Date gets the DateTime object which represents the current date of the system. It returns the DateTime object if you specify the date.
In this article, we will discuss using the Get-Date cmdlet to get the current date in PowerShell, and format specifier to get the current date and time.
PowerShell Get-Date
The Get-Date cmdlet in PowerShell gets the current date and time.
Syntax
Get-Date [[-Date] <DateTime>] [-Year <Int32>] [-Month <Int32>] [-Day <Int32>] [-Hour <Int32>] [-Minute <Int32>] [-Second <Int32>] [-Millisecond <Int32>] [-DisplayHint <DisplayHintType>] [-AsUTC] -UFormat <String> [<CommonParameters>]
Parameters
AsUTC: It converts the date value to the equivalent time in UTC.
Date: It specifies a date and time. Time is optional and returns 00:00:00 if not specified.
Day: It specifies the day of the month.
DisplayHint: It determines which elements of the date and time are displayed.
Format: It displays the date and time in the Microsoft .NET framework indicated by the format specifier.
Hour: It specifies the hour that is displayed.
Minute: It specifies the minute that is displayed.
Second: It specifies the second that is displayed.
Millisecond: It specifies the milliseconds in date.
Month: It specifies the month that is displayed.
Year: It specifies the year that is displayed.
UFormat: It displays the date and time in UNIX format.
Let’s understand using PowerShell Get-Date with examples.
Let’s practice!
Get the current date and time
PowerShell Get-Date cmdlet displayed the current date and time.
Get-Date
The above command outputs the current date and time in long date and long time formats.
24 January 2022 21:49:52
Get-Date Get elements of the current date and time
Get elements of the current date and time using the Get-Date DisplayHint parameter.
Get-Date -DisplayHint Date
It outputs the current date of your system.
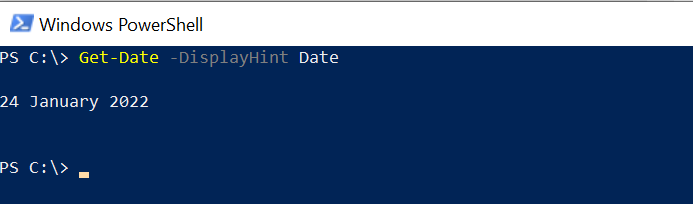
You can get the time element of the current date and time using the PowerShell Get-Date DisplayHint parameter.
Get-Date -DisplayHint Time
It outputs the time element of your local system’s current date and time.
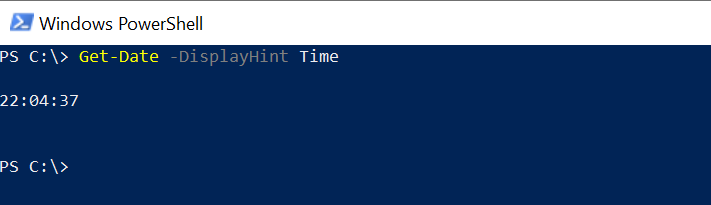
Get date and time with .NET format specifier
The Get-Date cmdlet in PowerShell uses different .NET format specifiers to customize the output format.
It uses a Format parameter to specify several format specifiers. The output of a command is a String object.
Get-Date -Format "MM/dd/yyyy HH:mm:ss"
In the above get date .net format specifier example, we have specified the customized date-time format MM/dd/YYY HH:mm:ss for the output date.
The output of the above command is:
PS C:\> Get-Date -Format "MM/dd/yyyy HH:mm:ss" 01-24-2022 22:13:21
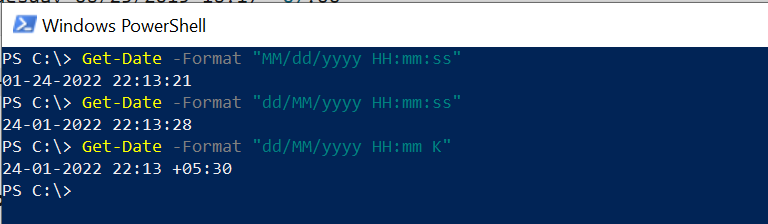
To get the current date and time in dd/MM/yyyy HH:mm:ss format, use
Get-Date -Format "dd/MM/yyyy HH:mm:ss"
The output of the above command is:
PS C:\> Get-Date -Format "dd/MM/yyyy HH:mm:ss" 24-01-2022 22:13:28
To get the current date and time in dd/MM/yyyy HH:mm:ss format with TimeZone offset from the current zone from UTC.
Get-Date -Format "dd/MM/yyyy HH:mm K"
The output of the above command is:
PS C:\> Get-Date -Format "dd/MM/yyyy HH:mm K" 24-01-2022 22:13 +05:30
.NET format specifiers to be used with the Get-Date command
Specifier | Definition |
---|---|
dddd | Day of the week – full name |
MM | Month number |
dd | Day of the month – 2 digits |
yyyy | Year in 4-digit format |
HH:mm | Time in 24-hour format – no seconds |
K | Time zone offset from Universal Time Coordinate (UTC) |
Cool Tip: How to get aduser creation date in PowerShell!
Get date and time with a UFormat Specifier
You can use several UFormat specifiers to customize the output format.
Get-Date uses the Format parameter to specify the format specifier. The output is a String object.
Get-Date -UFormat "%A %m/%d/%Y %R %Z"
The output of the above command is:
PS C:\> Get-Date -UFormat "%A %m/%d/%Y %R %Z" Monday 01/24/2022 22:28 +05
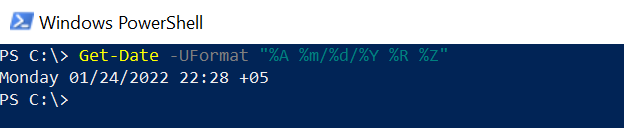
UFormat Specifier to be used with Get-Command are
Specifier | Definition |
---|---|
%A | Day of the week – full name |
%m | Month number |
%d | Day of the month – 2 digits |
%Y | Year in 4-digit format |
%R | Time in 24-hour format – no seconds |
%Z | Time zone offset from Universal Time Coordinate (UTC) |
Get Date’s day of the year in PowerShell
You can get the date’s day or numeric day using the DayofYear property.
(Get-Date -Year 2021 -Month 11 -Day 07).DayOfYear
In the above example, it gets the numeric day of the year using the Gregorian calendar.
In the above command, get-Date uses three parameters Year, Month, and Day with their values.
It is wrapped inside parentheses and the result is evaluated by the DayOfYear property.
PS C:\> (Get-Date -Year 2021 -Month 11 -Day 07).DayOfYear 311
PS C:\> (Get-Date -Year 2022 -Month 01 -Day 24).DayOfYear 24
PS C:\>
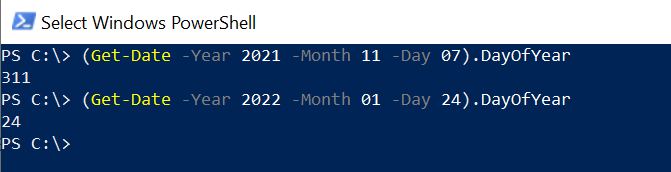
Get-Date – Convert the current date time to UTC time
You can convert the current date time to UTC time using the UFormat parameter.
UFormat format %Z specifier represents the UTC offset.
Get-Date -UFormat "%A %B/%d/%Y %T %Z" $Cur_DateTime = Get-Date $Cur_DateTime.ToUniversalTime()
In the above example, Get-Date uses the UFormat parameter with format specifiers “%A %B/%d/%Y %T %Z” to display the current system date and time.
UFormat specifier %Z represents the UTC offset of +5
$Cur_DateTime variable stores the current date and time of the system.
$Cur_DateTime uses ToUniversalTime() method to convert the current date and time to UTC offset time.
The output of the above command is:
PS C:\> Get-Date -UFormat "%A %B/%d/%Y %T %Z" Monday January/24/2022 22:43:15 +05
PS C:\> $Cur_DateTime = Get-Date PS C:\> $Cur_DateTime.ToUniversalTime()
24 January 2022 17:13:20
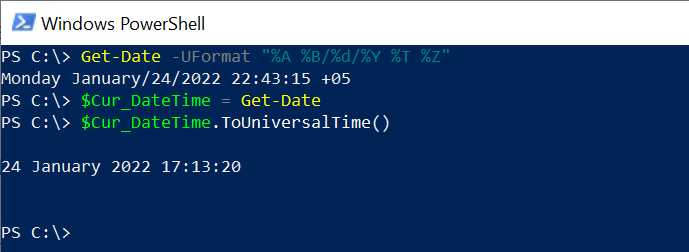
Get Yesterday Date using Get-Date in PowerShell
You can get yesterday’s date using the Get-Date AddDays() method in PowerShell.
(Get-Date).AddDays(-1)
In the above example, Get-Date is wrapped in parentheses and uses AddDays() method.
AddDays() method accepts the input parameter as a number, in this case, -1, and gets yesterday’s date.
PS C:\> (Get-Date).AddDays(-1)
23 January 2022 22:51:06
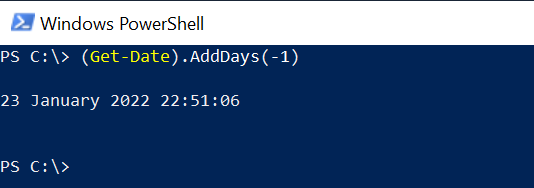
Check if the date is set for daylight savings time
Using the IsDaylightSavingTime() method, You can check if the date is adjusted for daylight savings time.
$Cur_DateTime = Get-Date $Cur_DateTime.IsDaylightSavingTime()
In the above example, $Cur_DateTime stores the current date and time of the local system.
$Cur_DateTime variable uses IsDaylightSavingTime() method to check if the date is set for daylight savings time and return a boolean value.
The output of the above command is:
PS C:\> $Cur_DateTime = Get-Date PS C:\> $Cur_DateTime.IsDaylightSavingTime() False
PS C:\>
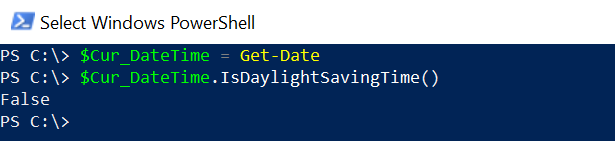
Add hours in the current date time in PowerShell
You can use AddHours() method to add hours to the current date and time.
The AddHours() method accepts a numeric value, ex… a number of hours.
(Get-Date).AddHours(5)
In the above example, Get-Date is wrapped inside parentheses and uses AddHours() method to add hours to the current date and time.
AddHours(5) adds the 5 hours in the current time.
The output of the above command is:
PS C:\> (Get-Date).AddHours(5)
25 January 2022 04:05:09
Cool Tip: How to concatenate strings in PowerShell!
PowerShell Get-Date Formats and UFormat Specifier
PowerShell Get-Date cmdlet returns the DateTime object and provides get-date formats and UFormat specifiers.
Format specifier | Meaning | Example |
---|---|---|
%A | Day of the week | Monday |
%a | Day of the week – abbreviated name | Mon |
%B | Month name – full | January |
%b | Month name – abbreviated | Jan |
%C | Century | 20 for 2022 |
%c | Date and time – abbreviated | Thu Jun 21 06:42:38 2023 |
%D | Date in mm/dd/yy format | 01/21/23 |
%d | Day of the month – 2 digits | 03 |
There are many get-date formats are available. You can find more about PowerShell get-date formats and Uformat specifiers on Microsoft PowerShell documentation.
Conclusion
I hope the above article on the PowerShell Get-Date cmdlet is useful to you.
You can use several .NET format or Unix format specifiers to customize the output’s format.
The Get-Date cmdlet in PowerShell returns the DateTime object. If you use Format or UFormat parameters, it will return the String object.
You can use the Get-Member cmdlet to get members of the PowerShell get-date cmdlet.
You can convert a DateTime object to a String object using the ToString() method.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.
Recommended Content
How to Convert String to DateTime in PowerShell
PowerShell Epoch Time and DateTime Conversion