To Compare dates in PowerShell, use the PowerShell operator lt
or gt
. It compares the date that is less or greater than the other date and returns the value of either True or False.
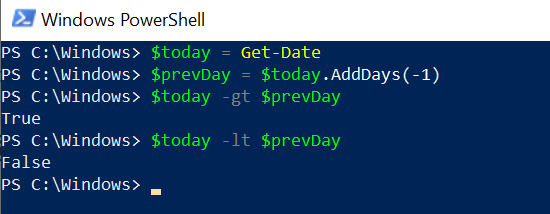
In PowerShell, Get-Date returns the DateTime object, hence comparing dates becomes much easier with the PowerShell operator lt or gt.
You can compare date strings in PowerShell provided they are in the correct DateTime format.
In this article, we will discuss how to compare dates in PowerShell and compare date strings in PowerShell.
PowerShell Compare Dates
Use the PowerShell operator like lt
( lesser than) or gt
( greater than) to compare two dates in PowerShell.
Let’s use an example to compare two dates in PowerShell.
# Get Current Date $date1 = Get-Date # Get Previous Date $date2 = (Get-Date).AddDays(-1) # Compare two dates, if $date1 is less than $date2 $date1 -lt $date2 # Compare two dates, if $date1 is greater than $date2 $date1 -gt $date2
In the above PowerShell script, Get-Date returns the current DateTime object and stores it in the $date1 variable, and the $date2 variable stores the previous day’s date.
In PowerShell for date comparison, it uses the -lt operator to check if $date1 is lesser than $date2 and it returns a boolean value as False
-gt operator checks if $date1 is greater than $date2 and returns a boolean value as True. It means $date1 is greater than $date2.
The output of the above PowerShell script to compare two dates using the PowerShell operator lt and gt are as given below:
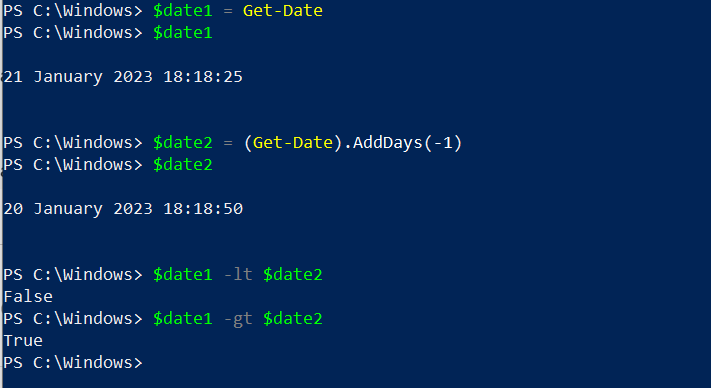
PowerShell Compare Date Strings
In PowerShell to compare date strings, strings that need to be compared against the date should have the correct date format else it will throw an exception while comparing two date strings.
# Get the current date of the user login $loginDate = Get-Date # User account expiry date $accountexpiryDate ="02-25-2023" # Compare date strings $loginDate -lt $accountexpiryDate
In the above PowerShell script, the $loginDate variable stores the current DateTime object. $accountexpiryDate variable stores the date in string format.
While comparing the dates, use the PowerShell operators like lt or gt to check if a date is less or greater than a later one, it returns the boolean value either True or False.
In the above script, the date in string format follows the system format, which means on my machine, the DateTime format is “mm-dd-yyyy“, hence date time comparison with string is possible.
The output of the above PowerShell to compare two dates is given below
PS C:\Windows> $loginDate = Get-Date PS C:\Windows> $loginDate
21 January 2023 18:45:59
PS C:\Windows> $accountexpiryDate ="02-25-2023" PS C:\Windows> $accountexpiryDate 02-25-2023
PS C:\Windows> $loginDate -lt $accountexpiryDate True
PS C:\Windows>
If we modify the $accountexpiryDate to “25-02-2023” ( dd-mm-yyyy), it would result in the exception “String was not recognized as a valid DateTime” as given below
PS C:\Windows> $accountexpiryDate ="25-02-2023"
PS C:\Windows> $loginDate -lt $accountexpiryDate
Could not compare "01/21/2023 18:45:59" to "25-02-2023". Error: "Cannot convert value "25-02-2023" to type
"System.DateTime". Error: "String was not recognized as a valid DateTime.""
At line:1 char:1
+ $loginDate -lt $accountexpiryDate
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : InvalidOperation: (:) [], RuntimeException
+ FullyQualifiedErrorId : ComparisonFailure
PS C:\Windows>
Conclusion
I hope the above article on how to compare two dates in PowerShell is helpful to you.
PowerShell operator lt and gt are used to compare dates and returns a boolean value.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.
Recommended Content
How to Convert String to DateTime in PowerShell