Often while working on DateTime-based operations, it’s common problem that we face to convert string to DateTime in PowerShell and DateTime to string in PowerShell.
In this article, I will explain to you different ways to convert string to datetime in PowerShell.
You can use the ParseExact method of the DateTime object or cast string to DateTime to convert string to DateTime format.
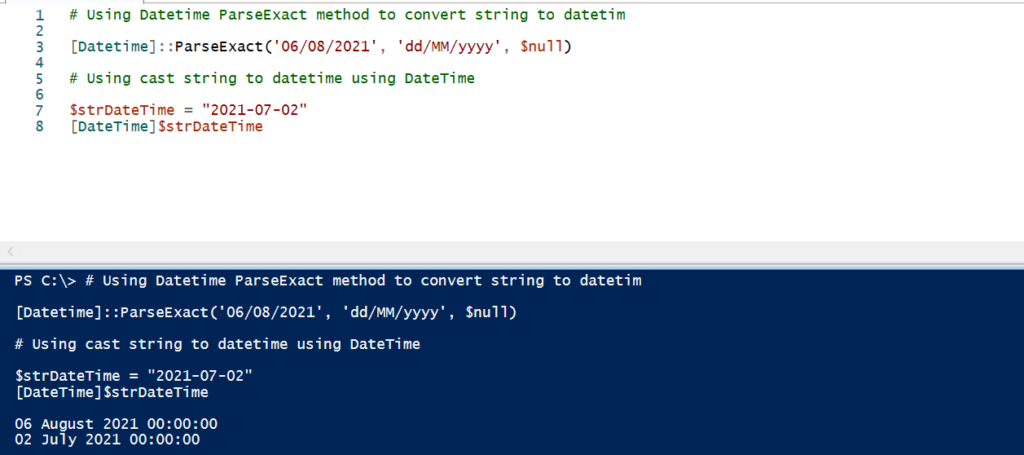
Convert String to DateTime using ParseExact
DateTime
class has the ParseExact method which converts the string representation of DateTime to DateTime using a specified date format.
Let’s consider a string variable having a string representation of DateTime. Using ParseExact()
the method will convert string to datetime format in PowerShell.
# Using Datetime ParseExact method to convert string to datetime $strDateTime = '06/08/2021' [Datetime]::ParseExact($strDateTime, 'dd/MM/yyyy', $null)
In the above PowerShell script, $strDateTime
the variable contains a DateTime format.
Using ParseExact
method of DateTime, take a string as input, DateTime format, and culture-specific format information, and convert string to DateTime.
The output of the above script in PowerShell to convert string to DateTime format as below
PS C:\> # Using Datetime ParseExact method to convert string to datetime
$strDateTime = '06/08/2021'
[Datetime]::ParseExact($strDateTime, 'dd/MM/yyyy', $null)
06 August 2021 00:00:00
PS C:\>
As in the above output, ParseExact converts string DateTime format to dd/MM/yyyy format DateTime.
Cool Tip: How to create a multiline string in PowerShell!
Convert String to Datetime using Cast
You can cast to convert string to DateTime format in PowerShell. cast string converts to DateTime format.
Let’s consider an example below to convert string to DateTime using cast
# Using cast string to datetime using DateTime $strDateTime = "2021-07-02" [DateTime]$strDateTime
The above PowerShell script, $strDateTime
stores string representation of the DateTime format.
Using [DateTime]string
, you can cast the string to DateTime format.
Cool Tip: How to use Get-Date in PowerShell to convert date to string!
The output of the above convert string to DateTime format using cast as below
PS C:\> # Using Datetime ParseExact method to convert string to datetime
$strDateTime = '06/08/2021'
[Datetime]::ParseExact($strDateTime, 'dd/MM/yyyy', $null)
06 August 2021 00:00:00
PS C:\>
You can store the converted string to DateTime format date in variable and use the DateTime operation as below
# Using cast string to datetime using DateTime $strDateTime = "2021-07-02" $convertDate = [DateTime]$strDateTime # Print variable datatype $convertDate.GetType() # Convert datetime to dd-mm-yyyy datetime format '{0:dd-MM-yyyy}' -f $convertDate # Convert datetime to mm-dd-yyyy datetime format '{0:MM-dd-yyyy}' -f $convertDate # Convert datetime to yyyy-mmm-dd datetime format '{0:yyyy-MMM-dd}' -f $convertDate
In the above PowerShell script, first, it converts a string to DateTime format and stores the DateTime value in $convertDate
a variable.
$convertDate variable has DateTime datatype, use GetType()
method to get the data type of variable.
Cool Tip: How to execute a script with noprofile parameter in PowerShell!
Once you have the DateTime variable, you can do any kind of DateTime operation like print DateTime in different DateTime formats like dd-mm-yyyy or mm-dd-yyyy or any other valid date time format.
The output of the above PowerShell script as below
IsPublic IsSerial Name BaseType
-------- -------- ---- --------
True True DateTime System.ValueType
02-07-2021
07-02-2021
2021-Jul-02
Cool Tip: How to create multiline comments in PowerShell!
Conclusion
I hope the above article on converting string to DateTime format using PowerShell is helpful to you while performing DateTime operations.
Use DateTime ParseExact()
method or cast to Datetime format to convert the string to DateTime.
PowerShell Tip: Use PowerShell $null to check if a variable is empty!
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.
Recommended Content
PowerShell Epoch Time and DateTime Conversion