Use the Split() and ToCharArray() methods of string objects to convert string to array in PowerShell. The Split() method returns a string array and ToCharArray() method returns the character array.
PowerShell String is an object of type System.String
and provides many methods for string manipulation like splitting the string, replacing the string, etc…
In this article, we will discuss how to use PowerShell to convert a string to an array using the Split() method and ToCharArray() method.
PowerShell Split String to Array
You can easily split the string into an array using the Split() method of string. The Split () method takes the separator as an input argument for breaking the string into multiple substrings.
$testString = "Hello,Welcome,to,ShellGeek,Website" # Split the string to Array $teststring.Split(',')
In the above PowerShell script, the $testString variable contains the string. To convert string to array, we have used the PowerShell String Split() method that takes comma (,) as a separator.
It returns the string array that contains the substring as given below.
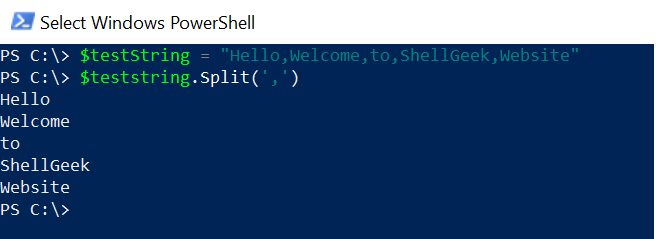
To check the returns data type of the result array, refer to the following code which stores the result array in the $strArr variable and uses the GetType() method to print the data type of the variable.
$testString = "Hello,Welcome,to,ShellGeek,Website" # Split the string to Array $strArr = $teststring.Split(',') # Print the result string array $strArr #Output Hello Welcome to ShellGeek Website # Get the data type of the variable $strArr.GetType() #output IsPublic IsSerial Name BaseType -------- -------- ---- -------- True True String[] System.Array
In the above PowerShell script, GetType() method over the $strArr variable prints the String[] array type.
Cool Tip: How to split string or text file on newline in PowerShell!
PowerShell Converts String to Array using ToCharArray()
Using the ToCharArray() method of the String object, you can convert the string to an array of characters.
$testString = "Hello,Welcome,to,ShellGeek,Website" # Convert string to array $charArr = $teststring.ToCharArray() # Print the character array $charArr # Get the data type of the variable $charArr.GetType()
In the above PowerShell script, the $testString variable contains the string value. We have used ToCharaArray() string method to convert a string to an array. It returns a character array.
To check the return type of the result array, we have stored the result in $charArr and used the GetType() method. It returns the result array data type as char[].
The output of the above PowerShell script that converts a string into an array is:
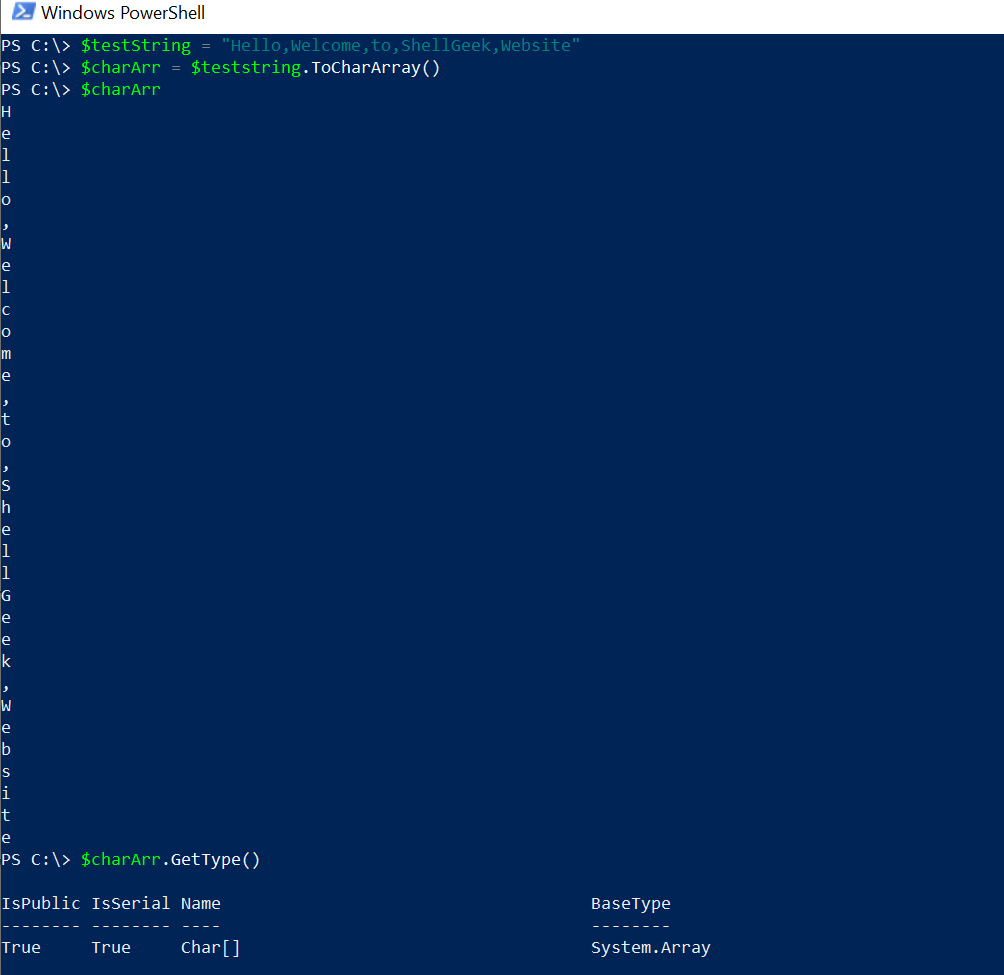
Cool Tip: How to convert string to byte array in PowerShell!
PowerShell Split String into Array and use Foreach
You can split the string into an array using the PowerShell string Split() method and store the results in a variable. The result is a string array that contains multiple substrings.
To access the element of the string array, you can use the foreach loop to iterate over and access each element of an array.
$testString = "Hello,Welcome,to,Shell Geek" # Split string by comma $strArr = $testString.Split(',') # Use the Splitted string result in foreach foreach ($str in $strArr) { Write-Host $str }
In the above PowerShell script, the $strArr variable stores the string array. To access the element of the array, we have used a foreach loop to iterate over the string array and print it using the Write-Host cmdlet.
The output of the above PowerShell script to split string to array and use foreach is:
Hello
Welcome
to
Shell Geek
Cool Tip: How to use GetEnumerator in PowerShell to read array data!
Conclusion
I hope the above article on how to convert the string to array in PowerShell is helpful to you.
PowerShell string provides many methods for string manipulation. Use the Get-Member() method to get all available members for the string.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.