Use the GetBytes()
method of the UTF8
encoding format in PowerShell to convert string to byte array. This method converts a string to bytes and returns a byte array.
[System.Text.Encoding] class provides methods to convert strings to and from using different encoding formats.
In this article, we will discuss how to use GetBytes() method in PowerShell to convert a string to byte array.
Convert String to Byte Array in PowerShell using GetBytes() Method
Use GetBytes()
method of UTF8 encoding format in PowerShell to convert the string to bytes array
# Declare string variable $str = "ShellGeek" # Get the data type of the variable $str.GetType() # converting string to bytes stream $bytesArr = [System.Text.Encoding]::UTF8.GetBytes($str) # Print the bytes array $bytesArr # Get the data type of the variable $bytesArr.GetType()
In the above PowerShell script, the $str
variable is a string type containing the value "ShellGeek"
. Using the GetBytes() method, it converts the string to bytes and assigns the result to the variable $bytesArr
that contains the bytes array.
GetType() method in PowerShell gets the data type for the variable.
The output of the above script in PowerShell converts a string to bytes using the GetBytes() method [System.Text.Encoding]
class is:
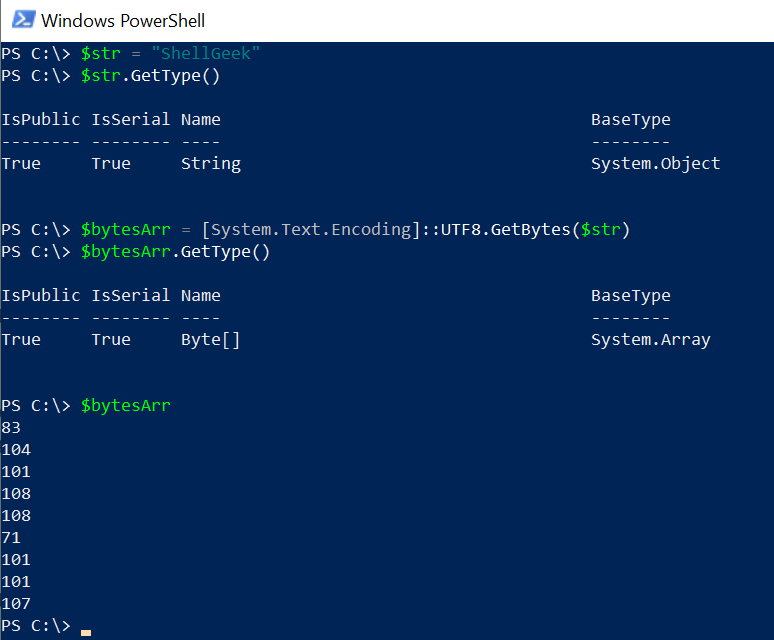
Cool Tip: How to convert string to integer in PowerShell!
PowerShell Convert String to Byte Stream using Unicode encoding
Use the Unicode encoding type supported by System.Text.Encoding
class to convert a string to a byte stream in PowerShell.
$str = "ShellGeek" $bytesArr = [System.Text.Encoding]::Unicode.GetBytes($str) $bytesArr
In the above PowerShell script, the $str contains the string value “ShellGeek”. The GetBytes() method of System.Text.Encoding
class uses the Unicode encoding type to convert a string to a byte stream.
The output of the above PowerShell script is:
PS C:\> $str = "ShellGeek"
PS C:\> $str.GetType()
IsPublic IsSerial Name BaseType
-------- -------- ---- --------
True True String System.Object
PS C:\> $bytesArr = [System.Text.Encoding]::Unicode.GetBytes($str)
PS C:\> $bytesArr
83
0
104
0
101
0
108
0
108
0
71
0
101
0
101
0
107
0
PS C:\>
A byte array is a collection of bytes, where each byte represents 8 bits of data.
In PowerShell to convert string to bit, convert a string to a byte array using the GetBytes() method of the System.Text.Encoding class and then iterate over each byte in byte array to convert to a binary string using the ToString() method of [System.Convert] class with a base of 2.
$str = "ShellGeek" # Convert a string to byte array $bytesArr = [System.Text.Encoding]::Unicode.GetBytes($str) $bits = "" foreach($byte in $bytesArr){ $bits += [System.Convert]::ToString($byte,2).PadLeft(8,'0') } Write-Host $bits
The output of the above PowerShell script converts a string to bits as follows:
PS C:\> $str = "ShellGeek"
PS C:\> $bytesArr = [System.Text.Encoding]::UTF8.GetBytes($str)
PS C:\> $bits = ""
PS C:\> foreach($byte in $bytes){$bits += [System.Convert]::ToString($byte,2).PadLeft(8,'0')}
PS C:\> $bits 0100100001100101011011000110110001101111001000000101011101101111011100100110110001100100
PS C:\>
Conclusion
I hope the above article on how to convert string to byte array in PowerShell using the GetBytes() method of UTF8 encoding format is helpful to you.
PowerShell strings are sequences of characters while the bytes are sequences of binary data. System.Text.Encoding
class provides methods to convert string to bytes and convert byte array to string using the GetString() method.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.