In PowerShell, converting string to integer is necessary when mathematical or conditional operations need to be performed on a value stored as a string.
Use the int
casting operator in PowerShell or TryParse()
method to convert string to int in PowerShell.
In this article, we will discuss how to convert string to int using a PowerShell script.
Convert String to Int in PowerShell using int Casting Operator
Use int
casting operator in PowerShell to convert the string to an integer, it will try to parse the string and gets the integer value.
# Declare string variable $str = "100" # Get the data type of the variable $str.GetType() # converting string to int $num1 = [int]$str # Print the number $num1 # Get the data type of the variable $num1.GetType()
In the above PowerShell script, the $str
variable is a string type containing the value "100"
. Using the [int] casting operator, it converts the string to an integer and assigns the result to the $num1 variable that contains the value 100
.
GetType() method in PowerShell gets the data type for the variable.
The output of the above PowerShell script to convert string to an int using the int casting operator is:
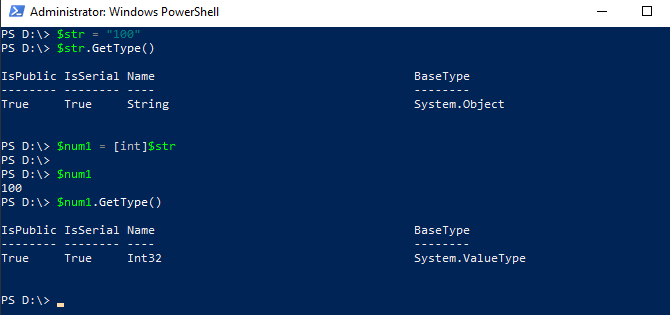
Cool Tip: How to convert integer to string in PowerShell!
Use TryParse Method to Convert String to Int in PowerShell
Use the TryParse() method from the System.Int32 class to convert a string to an integer in PowerShell. This method takes two arguments: a string to be converted and a variable to store the converted value.
The TryParse method returns the value True if the parsing is successful and stores the result in a variable. If the parsing is not successful, it returns False.
$str = "100" # Declare variable $num2 = 0 # Use TryParse method to convert string to int $result = [System.Int32]::TryParse($str,[ref]$num2) $num2
In the above PowerShell script, the $str
variable is a string type containing the value "100"
. $num2 variable declared with value 0 to be used in the TryParse method.
TryParse() method from the System.Int32 class takes $str
and $num2
arguments and parses them.
The output of the above PowerShell script for converting a string to an integer is:
PS D:\> $str = "100"
PS D:\> $num2 = 0
PS D:\> $result = [System.Int32]::TryParse($str,[ref]$num2) PS D:\> $num2
100
Conclusion
I hope the above article on how to convert string to int in PowerShell using the int casting operator and the TryParse method is helpful to you.
In PowerShell, it has the Parse() method that also converts the string to int but if the PowerShell string is not a valid integer, this method can throw an exception. Hence to handle exceptions use the TryParse() method.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.