In PowerShell to perform automation tasks, it’s quite often to convert data from different data types like converting an integer to a string.
To convert the integer to a string, use the ToString() method which is available in all .Net data types. Another way to convert the int to string is using the [string] type casting operator.
In this article, we will discuss how to convert integer to string using ToString() method and the type cast operator [string] in PowerShell.
How to Convert an Int to a String in PowerShell
Use the type cast operator [string] to convert the integer to string type value.
# Declare the variable with numeric value $num1 = 50 # Get the data type of the variable $num1.GetType() # Use the type cast operator [string] $str = [string]$num1 # Print the result $str # Get the data type for the variable $str.GetType()
In the above PowerShell script, the $num1
variable contains the integer value. Using the type cast operator in PowerShell [string]
, it converts the int to a string type.
Use the GetType() method in PowerShell to get the data type of the variable.
The output of the above PowerShell script to convert the integer to a string value is:
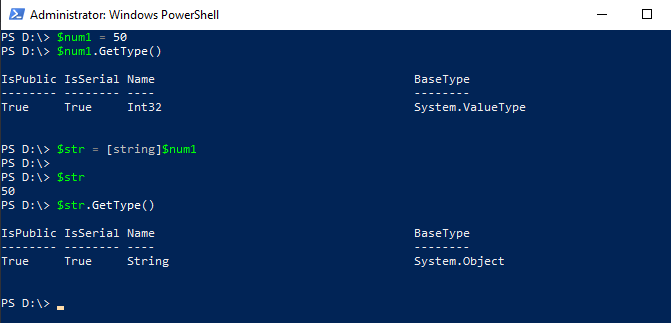
Cool Tip: How to convert string to integer in PowerShell!
Use ToString() to Convert integer to String in PowerShell
ToString() method is available in all .net data types. Using this method, convert the integer value to a string.
# Declare integer value variable $num1 = 50 # Use ToString() to convert int to string $str = $num1.ToString() $str
In the above PowerShell script, the $num1
variable contains the integer value 50
. Using the ToString() method with the $num1 variable, it converts the integer to a string and stores the result in the $str
variable.
The variable $str is the PowerShell string type.
Conclusion
I hope the above article on how to convert integer to string using PowerShell ToString() and type cast operator is helpful to you.
In PowerShell, you can convert variables to strings using ToString or -f operator.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.