In PowerShell, you can convert the variable to a string using the ToString()
method or the -f format
operator.
PowerShell allows you to work with different data types such as strings, integers, floats, arrays, etc., and convert variables to strings, especially when displaying the output to the user.
In this article, we will discuss converting PowerShell variables to strings and different ways to work effectively with different data types in your scripts.
PowerShell Convert Integer Variables to String
In PowerShell, use the ToString() method or the -f format operator to convert the int variable to a string.
Using the ToString() method:
$temp = 123 $stringNumber = $temp.ToString()
In the above PowerShell script, we have defined a variable $temp
that contains the integer data type value. To convert an integer variable to a string data type, we use ToString() method and store the converted value in the variable $stringNumber
.
The output of the above PowerShell script to convert an integer to a string is:
PS C:\> $temp = 123
PS C:\> $stringNumber = $temp.ToString() PS C:\> $stringNumber
123
PS C:\>
Using the -f format operator
$temp = 123 $stringNumber = "{0}" -f $temp
The above PowerShell uses the -f format operator to convert the int variable to string type.
Convert Float Variable to String in PowerShell
In PowerShell, use the ToString() method or the -f format operator to convert the floating-point number to a string.
Using the ToString() method:
$temp = 123.12 $stringNumber = $temp.ToString()
In the above PowerShell script, we have defined a variable $temp
that contains the integer data type value. To convert a floating-point variable to a string data type, we use ToString() method and store the converted value in the variable $stringNumber
.
The output of the above PowerShell script to convert a float variable to a string is:
PS C:\> $temp = 123.12
PS C:\> $stringNumber = $temp.ToString() PS C:\> $stringNumber
123.12
PS C:\>
Using the -f format operator
$temp = 123.12 $stringNumber = "{0}" -f $temp
The above PowerShell uses the -f format
operator to convert the float variable to string type.
Convert Boolean Variable to String in PowerShell
Use the ToString() method to convert a boolean variable to a string.
$boolValue = $true $stringBoolean = $boolValue.ToString()
In the above PowerShell script, the $boolValue
variable stores a boolean value True. Using the ToString() method, it converts a boolean variable to a string.
Using Variables in Strings
PowerShell supports string interpolation that allows you to embed variables directly in a string by enclosing them in curly braces ({}) and prefixing it with a dollar sign ($).
$username = "Tom" $age = 27 $stringMsg = "Hello, my name is $($username), my age is $($age)"
In the above PowerShell script, $username
and $age
variables of data types string and integer respectively. We have used string interpolation to embed these variables into the string.
The output of the above PowerShell script is:
PS C:\> $username = "Tom"
PS C:\> $age = 27
PS C:\> $stringMsg = "Hello, my name is $($username), my age is $($age)"
PS C:\> $stringMsg
Hello, my name is Tom, my age is 27
PS C:\>
Cool Tip: How to check if a variable contains a string in PowerShell!
Convert PowerShell Objects to Strings
You can convert PowerShell objects into String using the ToString() method or Out-String() method.
Using the ToString() method for converting PowerShell objects to Strings.
$processInfo = Get-Process -Id 7372 $processString = $processInfo .ToString()
Using the Out-String method for converting PowerShell objects to Strings. The Out-String method provides a more detailed output.
$processInfo = Get-Process -Id 7372 $processString = $process | Out-String
The output of the above PowerShell script is:
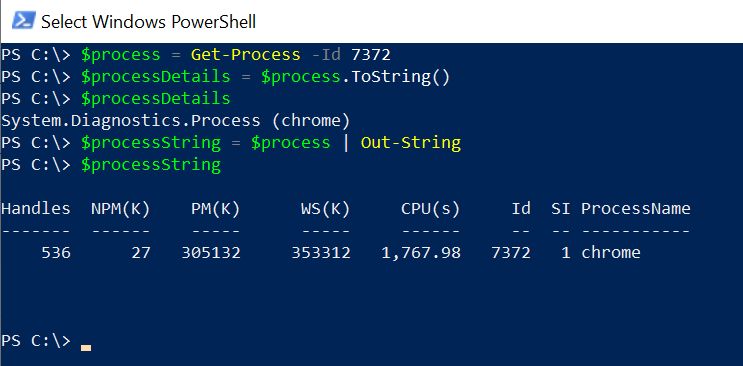
Cool Tip: How to check if a string variable is null or empty in PowerShell!
Conclusion
I hope the above article on how to convert variables to strings in PowerShell ToString() method or -f format operator is helpful to you.
While working with floating-point numbers, use the -f format operator and while converting objects, use the Out-String for more detailed output.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.