GetEnumerator in PowerShell is used to iterate through the Hashtable or array to read data in the collection.
GetEnumerator doesn’t modify the collection data. Use foreach or foreach-object in PowerShell to iterate over the data read from the GetEnumerator.
In this article, we will discuss how to use GetEnumerator in PowerShell to loop through the HashTable or array in PowerShell
Use GetEnumerator in PowerShell to Read Data in HashTable
Hashtable stores the items in key-value pairs. Use GetEnumerator in PowerShell to loop through the HashTable items and pass items to the foreach or foreach-object to read key-value pairs.
$employees = @{1 = "Tom"; 2 = "Gary" ; 3 = "Thomas"} # Display keys and values of HashTable using GetEnumerator $employees.GetEnumerator() # Iterate through the Hashtable Items using GetEnumerator $employees.GetEnumerator() | ForEach-Object { Write-Output "Key = $($_.key) , Value = $($_.Value)" }
In the above PowerShell script, the $employees
variable contains the HashTable having key-value pairs.
GetEnumerator in PowerShell iterates through the HashTable and displays the keys and values of the HashTable.
To read all items of HashTable, use GetEnumerator and pipe the HashTable output objects to ForEach-Object. ForEach-Object loop through the objects and prints the keys and values of the HashTable.
The output of the above PowerShell script to iterate through HashTable is:
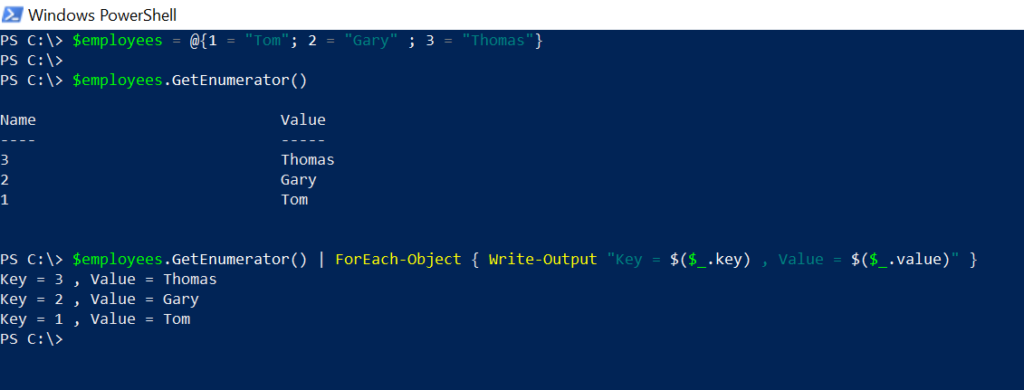
GetEnumerator in PowerShell to Read Array
A PowerShell array is a data structure that stores collections of multiple items. To read the data in the collection, use GetEnumerator in PowerShell. It iterates through the array.
$emp = @("Tom","Gary","Thomas") # Use the GetEnumerator to iterate throgh an array, display name $emp.GetEnumerator() # Display name of the employee $emp.GetEnumerator() | ForEach-Object { Write-Output "Name = $_" }
In the above PowerShell script, the $emp
is an array that stores string data type elements.
Use the GetEnumerator
in PowerShell to iterate over an array and pipes the output of the array objects to ForEach-Object
. It displays the employee’s name.
The output of the above PowerShell script to loop through an array using GetEnumerator in PowerShell is:
PS C:\> $emp = @("Tom","Gary","Thomas") PS C:\>
PS C:\> $emp
Tom
Gary
Thomas
PS C:\> $emp.GetEnumerator() Tom
Gary
Thomas
PS C:\> $emp.GetEnumerator() | ForEach-Object { Write-Output "Name = $_" } Name = Tom
Name = Gary
Name = Thomas
PS C:\>
Conclusion
I hope the above article helped you to understand the GetEnumerator in PowerShell to iterate through HashTable or Array.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.