Use the ToString()
method of [System.BitConverer]
class in PowerShell to convert byte array to hex string. This method takes a byte array as an input parameter and get the hexadecimal representation of the byte array.
A hex string is a representation of binary data in hexadecimal format. It is a base-16 numbering system that uses 0-9 digits and A-F letters to represent values from 0 to 15.
[System.BitConverter] class provides methods to convert numeric types values to and from byte arrays as well as to convert byte arrays to other data types.
In this article, we will discuss how to use ToString() method in PowerShell to convert byte array to hex string.
Convert Byte Array to Hex String in PowerShell using ToString() Method
Use ToString()
method of [System.BitConverter]
class in PowerShell to convert byte array to hex string.
Let’s understand with the help of an example to create a byte array and use it to convert a byte array to a hex string.
# Declare string variable $str = "ShellGeek" # converting string to bytes array $bytesArr = [System.Text.Encoding]::UTF8.GetBytes($str) # Print the bytes array $bytesArr # Convert byte array to hex string $hex = [System.BitConverter]::ToString($bytesArr) # Print the hex string $hex
In the above PowerShell script, the $str
variable is a string type containing the value “ShellGeek”. Using the GetBytes() method, it converts the string to a byte array and assigns the result to the variable $bytesArr
that contains the byte array.
To convert a byte array to a hex string, it uses the ToString() method of the System.BitConverter class that takes a byte array as an input parameter and returns a hex string
The output of the above script in PowerShell converts the byte array to a hex string.
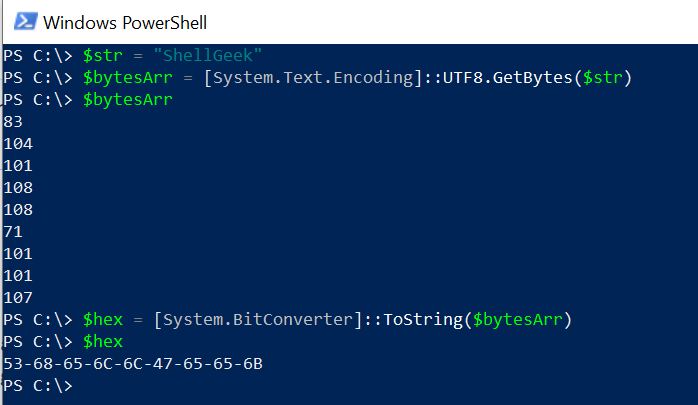
Cool Tip: How to convert string to integer in PowerShell!
Convert Byte Array to Hex String in PowerShell
Use [System.BitConverter]
class that provides the method ToString() to convert byte array to hex string.
In the following script, the $bytesArr
variable contains the binary representation of the string “ShellGeek”.
# Create a byte array $bytesArr = [byte[]]@(83,104,101,108,108,71,101,101,107) # Convert byte array to hex string $hex = [System.BitConverter]::ToString($bytes) # Print the string $hex
In the above PowerShell script, System.BitConverter
class has GetString() method in PowerShell to convert a byte array to a hex string. This method takes an input parameter as a byte array and returns a string.
The output of the script converts the byte array to a hex string.
# Create a byte array
PS C:\> $bytesArr = [byte[]]@(83,104,101,108,108,71,101,101,107)
# Convert byte array to hex string
PS C:\> $hex = [System.BitConverter]::ToString($bytes)
# Print the hex string
PS C:\> $hex
PS C:\> ShellGeek
Hex string can be converted to a byte array using the FromHexString() method of [System.Convert] class in PowerShell version 7.1 onwards.
# byte array $bytesArr = [byte[]]@(83,104,101,108,108,71,101,101,107) # Convert byte array to hex string $hex = [System.BitConverter]::ToString($bytes) # remove - between hex string $hexString = $hex.Replace('-','') # convert hex string to byte array using FromHexString method $bytes = [System.Convert]::FromHexString($hexString) # Print the bytes Write-Host $bytes
In the above PowerShell script, create a byte array and convert it to a hex string using the ToString() method of the System.BitConveter class.
In PowerShell version 7.1 onwards, System.Convert class provides the FromHexString() method that converts hex string to byte array.
In an older version of PowerShell, use the following script to convert hex string to byte array.
# byte array $bytesArr = [byte[]]@(83,104,101,108,108,71,101,101,107) # Convert byte array to hex string $hex = [System.BitConverter]::ToString($bytes) # remove - between hex string $hexString = $hex.Replace('-','') # Convert hex string to byte array [byte[]]@(for ($i = 0; $i -lt $hexString.Length; $i += 2) { [convert]::ToByte($hexString.Substring($i, 2), 16) })
Cool Tip: How to convert guid to string in PowerShell!
Conclusion
I hope the above article on how to convert byte array to hex string in PowerShell using the GetString() method of UTF8 encoding format is helpful to you.
Converting a byte array to a hex string can be useful for tasks such as generating cryptographic keys or working with binary data.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.