Use the PowerShell String replace()
method or replace
operator to replace the string in the array. e.g. $fileArr | ForEach-Object{$_.Replace('csv','xlsx')}
replace the string in the array.
In this article, we will discuss how to use PowerShell replace() method or replace operator to replace a string in an array with another string.
PowerShell replace() to Replace String in Array
PowerShell String built-in replace() method takes two arguments; a string to search and a string to replace with the found text.
# Create the array $fileArr = @('D:\PS\Data_V1.csv','D:\PS\Data_V2.csv','D:\PS\Data_V3.csv') # Replace string in the array $fileArr | ForEach-Object{$_.Replace('csv','xlsx')}
In the above PowerShell script, the $fileArr is an array that stores the files having a .csv extension.
To replace a string in the array, pass the content of array $fileArr to ForEach-Object to iterate over each element and replace the string in the array.
PowerShell replace()
function takes 2 arguments:
- the substring to search for in the given string, in the above example, CSV
- the replacement string for the found text, in the above example, xlsx,
The output of the above script to replace a string in the array with another string is:
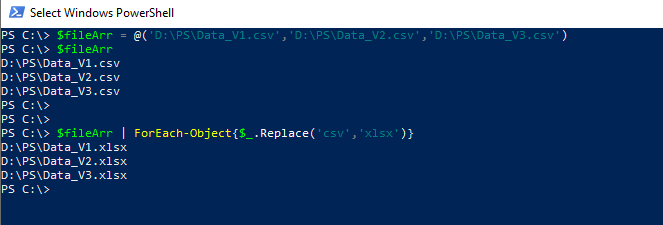
Cool Tip: How to replace the first occurrence of a string in PowerShell!
PowerShell replace Operator to Replace String in Array
Using the PowerShell replace operator, we can easily replace strings in the array.
The replace operator in PowerShell takes two arguments; string to find for in a string and replacement string for the found text.
# Create the array $fileArr = @('D:\PS\Data_V1.csv','D:\PS\Data_V2.csv','D:\PS\Data_V3.csv') # Use replace operator to replace file extention $fileArr -replace 'csv$','xlsx'
The above PowerShell script uses the replace operator to replace string in the array with another string.
replace operator takes 2 arguments:
- the substring to search for in a string, csv$ represents the end of the string.
- the replacement string, xlsx
In the first example, replace
operator replaces file extension .csv with another string in the array.
The output of the above Powershell script to replace the string in the array is:
PS C:\> $fileArr = @('D:\PS\Data_V1.csv','D:\PS\Data_V2.csv','D:\PS\Data_V3.csv')
PS C:\> $fileArr | ForEach-Object{$_.Replace('csv','xlsx')}
D:\PS\Data_V1.xlsx
D:\PS\Data_V2.xlsx
D:\PS\Data_V3.xlsx
PS C:\> $fileArr -replace 'csv$','xlsx'
D:\PS\Data_V1.xlsx
D:\PS\Data_V2.xlsx
D:\PS\Data_V3.xlsx
PS C:\>
Cool Tip: How to replace variables in a file using PowerShell!
Conclusion
I hope the above article on how to replace a string in the array using PowerShell replace() method is helpful to you.
Cool Tip: How to use hashtable in PowerShell!
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.