Hashtable in PowerShell is a data structure that stores the key-value pairs in a hash table. Keys and values in the PowerShell hashtable can have strings, integers, or any object type data.
Hashtable is similar to an array but data is stored as key-value pairs and it allows to access the value using the keys. The key is a unique identifier and the value is data that is associated with the key.
In this article, we will discuss the Hash table in PowerShell and how to use PowerShell HashTables.
How to Create Hashtable in PowerShell
Hashtable in PowerShell can be created using different ways.
Use @{} notation to create a hash table
PowerShell hashtable can be declared using the @{} notation.
Syntax
$stage1Server = @{}
Let’s create a hashtable in PowerShell that stores the server configuration for a given project.
$stage1Server = @{ "Server" = "IT-101" "Port" = "80" "Username" = "admin" "DBServer" = "DB-CORP1" "DBUser" = "dbadmin" "DbTimeOut" = 120 }
The output of the above PowerShell to create hashtable in PowerShell is:
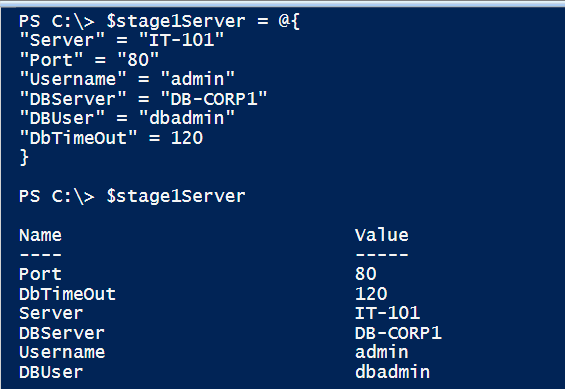
Use the New-Object cmdlet to create a hashtable
Using the PowerShell New-Object cmdlet, you can create a hashtable. The New-Object cmdlet creates a new instance of System.Collections.Hashtable class to create a hashtable.
$ServerConfig = New-Object -TypeName System.Collections.Hashtable
Using the [ordered] attribute to create a hashtable
You can use the [ordered] attribute to create a hashtable in PowerShell, keys in the ordered dictionaries always appear in the order.
[ordered] attribute create an instance of the System.Collections.OrderedDictionary
class.
$serverConfig = [ordered]@{ "Server" = "DB-102" "User" = "admin" "Password" = "secret" }
Hashtable Properties and Methods
Hashtable in PowerShell has several properties and methods to perform the operation.
PowerShell has a Get-Member cmdlet that gets the properties and method for the object.
# Declare hashtable $serverConfig = @{} # Get the properties and methods for hashtable object $serverConfig | Get-Member
The output of the above PowerShell script to get properties and methods available for hash table object is:
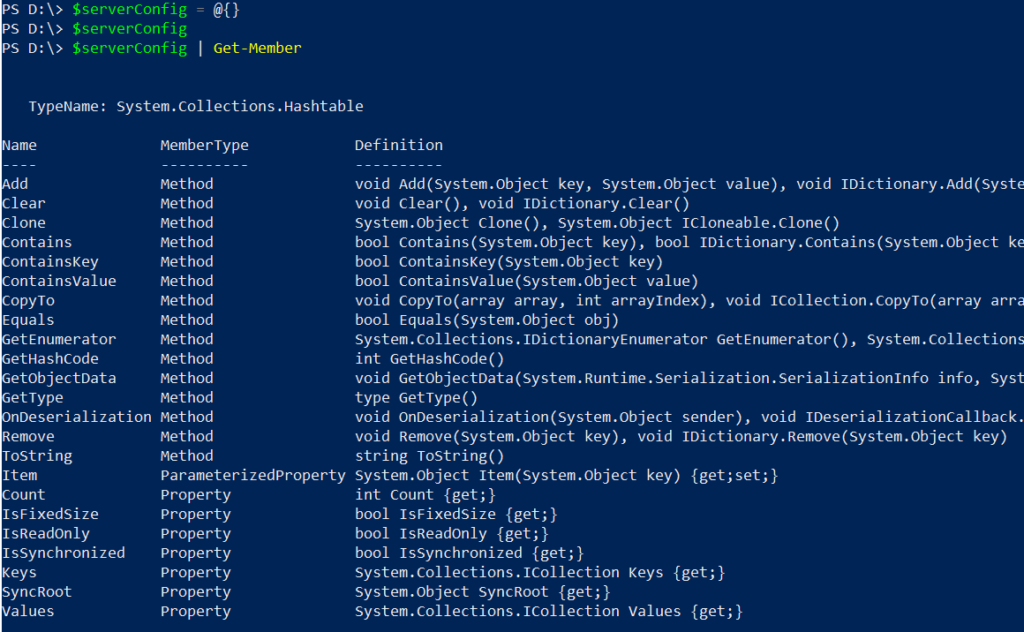
Adding Items to a Hashtable in PowerShell
PowerShell hashtable object has Add method that is used to add key-value pair as an element to the hashtable.
# Create hashtable $serverConfig = @{} # Add value to hashtable $serverConfig.Add('Server','DB-102') $serverConfig.Add('Subnet','255.255.255.0') # Display the Hashtable $serverConfig
In the above PowerShell script, we have created hashtable $serverConfig. Using the hashtable object Add() method added key-value pair.
$serverConfig hashtable stores the key-value pair of data.
The output of the above $serverConfig hashtable is:
# Create hashtable
$serverConfig = @{}
# Add value to hashtable
$serverConfig.Add('Server','DB-102')
$serverConfig.Add('Subnet','255.255.255.0')
# Display the Hashtable
$serverConfig
Name Value
---- -----
Server DB-102
Subnet 255.255.255.0
$serverConfig hashtable contains key-value pair data of different data types like string and alphanumeric.
PowerShell provides another way to add all key-value pairs to Hashtable instead of adding them one by one.
# Add the key-value pairs to hashtable all at once $stage1Server = @{ "Server" = "IT-101" "Port" = "80" "Username" = "admin" "DBServer" = "DB-CORP1" "DBUser" = "dbadmin" "DbTimeOut" = 120 }
In the above PowerShell script, the $stage1Server hashtable is created using @{} notation, and all the key-value pairs are added at once. An equal sign (=) is used to separate the key from its value.
The output of the above hashtable is:
PS D:\> $stage1Server
Name Value
---- -----
Port 80
DbTimeOut 120
Server IT-101
DBServer DB-CORP1
Username admin
DBUser dbadmin
PowerShell Tip: Keys are unique in the hashtable, if you try to add a similar key again with the same or different value it will throw an exception “Item has already been added. Key in dictionary:”
Access Items from a Hashtable
PowerShell provides several ways to access items in a hashtable.
Use the square bracket [] notation to retrieve the items from the hashtable. You will have to provide a specific key to access the value or data associated with that key.
Let’s consider the above-created $stage1Server hashtable and use the [] (square bracket) to access the item.
$stage1Server["Server"]
In the above PowerShell script, the hashtable $stage1Server object uses the [] to access the value associated with a specific key ‘Server‘.
The output of the above script returns the Server value from the hash table in PowerShell.
PS D:\> $stage1Server["Server"] IT-101
Another way to get elements in a hashtable is to use Item parameterized property which takes the specific key as input and retrieves the value associated with a key.
PS D:\> $stage1Server.Item("UserName") admin PS D:\>
You can access an item in a hashtable using the key. In the following PowerShell script, to get the port number, use the hashtable object with its key.
PS D:\> $stage1Server.Port 80 PS D:\>
Loop through Hash table in PowerShell
PowerShell provides several ways to loop through a hashtable to access the hashtable elements.
Using the Foreach to loop through a hashtable
Powershell has a foreach statement to iterate in a collection of items. You can use the foreach to traverse through the hashtable collection.
Let’s use the $stage1Server hashtable to access the elements using the foreach.
foreach($key in $stage1Server.Keys) { Write-Host "$($key) is $($stage1Server[$key])" }
The above PowerShell script uses a foreach statement to iterate hashtable keys in a hashtable $stage1Server. It uses the square bracket notation [] to access the value associated with a key.
The output of the above PowerShell script for looping through a hashtable is:
PS C:\> foreach($key in $stage1Server.Keys) {
Write-Host "$($key) is $($stage1Server[$key])"
}
Port is 80
DbTimeOut is 120
Server is IT-101
DBServer is DB-CORP1
Username is admin
DBUser is dbadmin
PS C:\>
Using the ForEach-Object to iterate hashtable
Using the Foreach-object in PowerShell, you can loop through the hashtable.
Let’s consider the $stage1Server hashtable to understand using of Foreach-object
for iteration.
$stage1Server.keys|ForEach-Object { Write-Host "$($_) is $($stage1Server[$_])" }
In the above PowerShell script, $stage1Server.Keys pipe to the ForEach-Object
command for iteration. In the script block, it uses the Write-Host
command to display the value associated with a key item in a hashtable.
The output of the above script is:
Port is 80
DbTimeOut is 120
Server is IT-101
DBServer is DB-CORP1
Username is admin
DBUser is dbadmin
Using the GetEnumerator to loop through a hashtable
The PowerShell hash table object has GetEnumerator() method that can be used to enumerate the collections.
In the following script, the $stage1Server hashtable uses GetEnumerator()
method and pipes the output to the foreach-object
for iteration of the enumerator object. In the script block, it uses the key and value to retrieve the items in a hashtable.
$stage1Server.GetEnumerator()|ForEach-Object { Write-Host "$($_.Key) : $($_.Value)" }
The output of the above PowerShell script is:
Port : 80
DbTimeOut : 120
Server : IT-101
DBServer : DB-CORP1
Username : admin
DBUser : dbadmin
Modify the Hashtable in PowerShell
You can modify the items in a hashtable using different ways.
The simple way to modify the value of the specific key in a hashtable, use square bracket notation []
to specify the key and assign the value. It will overwrite an existing value associated with a key in a hashtable.
$stage1Server["Server"] = "IT-101A"
Output:
PS D:\> $stage1Server["Server"] = "IT-101A" PS D:\> $stage1Server
Name Value
---- -----
Port 80
DbTimeOut 120
Server IT-101A
DBServer DB-CORP1
Username admin
DBUser dbadmin
Another way to change or update the value in a hashtable is using the Item parameterized property.
Item parameterized property of a hashtable object has {get; set;}
to retrieve and set the value.
$stage1Server.Item("Server") = "IT-101-South"
Output:
PS D:\> $stage1Server.Item("Server") = "IT-101-South" PS D:\> $stage1Server
Name Value
---- -----
Port 80
DbTimeOut 120
Server IT-101-South
DBServer DB-CORP1
Username admin
DBUser dbadmin
Remove Items in a Hashtable
PowerShell hashtable object has Remove() method that takes the key as an input parameter to remove the key-value pair from the hashtable.
$stage1Server = @{ "Server" = "IT-101" "Port" = "80" "Username" = "admin" "DBServer" = "DB-CORP1" "DBUser" = "dbadmin" "DbTimeOut" = 120 } # Remove element from the hashtable $stage1Server.Remove("DBUser")
The output of the above PowerShell script deletes the key-value pair from the hashtable.
PS D:\> $stage1Server.Remove("DBUser") PS D:\> $stage1Server
Name Value
---- -----
Port 80
DbTimeOut 120
Server IT-101-South
DBServer DB-CORP1
Username admin
Conclusion
Hashtable in PowerShell is a very powerful data structure that can be used to add, modify, delete and retrieve items from the hashtable easily.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.