In PowerShell, use the Get-ChildItem cmdlet to get certificate details, list all certificates in the personal store or remote computer, get installed certificates, and display certification details like Thumbprint, Subject, NotAfter, etc…
Certificates are stored in Certificate Store. Use the Certmgr.msc command in Windows to access the certificate Store, or open the Control Panel and search for manage computer certificates.
Using PowerShell to get the windows certificate details is very much easy and we can view all certificate details and export them to a CSV file.
In this post, we will discuss how to get certificate details from the root store, and list certificates in the personal store. The certificate provider exposes the certificate namespace as Cert:
drive in PowerShell.
Get Certificate details stored in the Root directory on a local machine
Get-ChildItem Cert:\LocalMachine\Root\* | ft -AutoSize
In the above example, PowerShell Get-ChildItem
cmdlet uses the path Cert:\LocalMachine\Root to get certificate information from the Root directory on a local machine account.
The above PowerShell command list all certificates from the Root directory and displays certificate details below
PSParentPath: Microsoft.PowerShell.Security\Certificate::LocalMachine\Root Thumbprint Subject ---------- ------- E4C3B69139C26ED0620ADF4F727C9D19BF66391 CN=corp-in-252 EDD4EEAE6000A7F40C3202C171E30148030C072 CN=Microsoft Root Certificate Authority, DC=microsoft, DC=com EE36A4562FB2E05DBB3S32323ADF445084ED656 CN=Timestamping CA, OU=Certification, O=, L=D... E43489159A5200D93D022CCAF37E7FE20A8B419 CN=Microsoft Root Authority, OU=Microsoft Corporation, OU=Copyrig... 784E459FF99D8FD97AFS46DCDCBCB90E0B7FCD5 CN=localhost 22B46C76E1305104F230517E6E504D43AB10B5 CN=Symantec Enterprise Mobile Root for Microsoft, O=Symantec Corp... AF43288AD2722103B6AB1428485EA3014C0BCFE CN=Microsoft Root Certificate Authority 2011, O=Microsoft Corpora...
PowerShell Tip: How to find a certificate by a thumbprint in PowerShell!
List Certificates in Personal Store
Certificates stored in the personal store can be easily retrieved using the Get-ChildItem cmdlet.
#Get installed certificates from localmachine $certs = Get-ChildItem Cert:\LocalMachine\My | ft -AutoSize #Display results in console $certs
In the above PowerShell script, the Get-ChildItem cmdlet uses the Cert:\LocalMachine\My path to get all certificate lists. It lists certificates in the Personal store along with certification details like Thumbprint, and Subject.
The output of the above PowerShell script shows all certificates from the personal stores and certificates Thumbprint and Subject properties as below
Thumbprint Subject ---------- ------- D4C3B69139C26AD0610FDF4F727C9D19BF66391 CN=corp-in-252 984E459FF9987FD97AFC46DCDCBCB90E0B7FCD5 CN=localhost 30B2EF587D70C873A46C34586599B94BF8F42B4 CN=localhost 21E1CF06BA981753337D4773CF9CB1D29100684 CN=localhost 10D6649DC0672CC1866F26B0E6BA4E7BFFCA4E3 CN=corp-in-252 0B8DF4B103544C05FB9633DB5553727803BEE2D CN=corp-in-252.shellgeek.org
PowerShell Tip: How to get the certificate expiration date in PowerShell!
List Certificates on Remote Computer using PowerShell
Use the Invoke-Command cmdlet in PowerShell with the remote computer name to run the script block that runs the Get-ChildItem cmdlet to get certificates on the remote server.
Run the following command to list certificates on the remote computer.
Invoke-Command -ComputerName corp-in-254 -Scriptblock {Get-ChildItem Cert:\LocalMachine\My\ }
In the above PowerShell script, Invoke-Command uses the ComputerName property to specify the remote server name. The Get-ChildItem cmdlet uses a remote computer LocalMachine store path to get certificates and their details.
The output of the above PowerShell script to get all certificates from the remote computer and view their details as below:
PS C:\> Invoke-Command -ComputerName corp-in-254 -Scriptblock {Get-ChildItem Cert:\LocalMachine\My\ }
PSParentPath: Microsoft.PowerShell.Security\Certificate::LocalMachine\My
Thumbprint Subject PSComputerName
---------- ------- --------------
8D0718A1037DB06BD4557B6BFB733E73D7962AD3 CN=be32a42c-7d1f-6732-a173-7e4fac624a... corp-in-254
PowerShell Tip: How to export the certificate to PEM format in PowerShell!
Get Certificate FriendlyName in PowerShell
In PowerShell to get the certificate friendly name, use the FriendlyName property of the certificate.
# Get all certificates $mycertificates = Get-ChildItem -Path Cert:\LocalMachine\My\ # Find Friendly Name of the certificate $mycertificates | Select ThumbPrint,FriendlyName
In the above PowerShell script, the Get-ChildItem cmdlet retrieves all certificates from LocalMachine\My
store and stores them in the $mycertificates
variable.
Use the FriendlyName property of a certificate to return the string type value.
The output of the above script to find the friendly name of the certificate in PowerShell is:
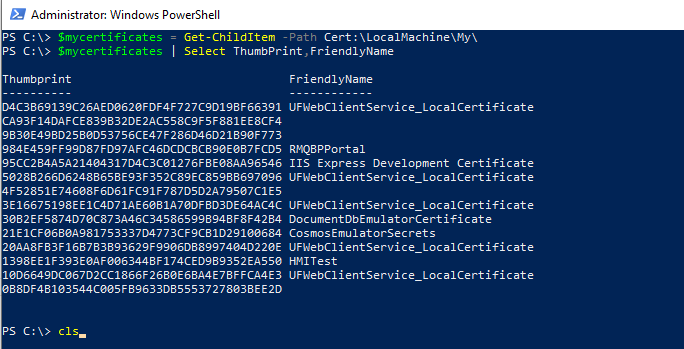
Get Certificate Subject Name in PowerShell
In PowerShell to retrieve the certificate subject name, use the SubjectName property of the certificate.
# Get the certificate by thumbprint $uacert = Get-ChildItem -Path 'Cert:\LocalMachine\UA Applications\841048409e5e81af553a6fa599f0d7ea8c2f5c08' # Obtain SubjectName of the certificate $uacert.SubjectName.Name
In the above PowerShell script, the Get-ChildItem cmdlet retrieves the certificate details by the thumbprint and stores it in the $uacert
variable.
Use the SubjectName property of a certificate to return the X500 DistinguishedName in string type.
The output of the above script to find the subject name of a certificate in PowerShell is:
PS C:\> $uacert = Get-ChildItem -Path 'Cert:\LocalMachine\UA Applications\841048409e5e81af553a6fa599f0d7ea8c2f5c08'
PS C:\> $uacert.SubjectName.Name
CN=UA Configuration Tool, DC=CORP-EU-102
PS C:\>
Get Certificate Issuer Name in PowerShell
In PowerShell to retrieve the certificate issuer name, use the IssuerName property of the certificate.
# Get the certificate by thumbprint $uacert = Get-ChildItem -Path 'Cert:\LocalMachine\UA Applications\841048409e5e81af553a6fa599f0d7ea8c2f5c08' # Obtain IssuerName of the certificate $uacert.IssuerName.Name
In the above PowerShell script, the Get-ChildItem cmdlet retrieves the certificate details by the thumbprint and stores it in the $uacert
variable.
Use the IssuerName property of a certificate to return the X500 DistinguishedName in string type.
The output of the above script to find the issuer name of a certificate in PowerShell is:
PS C:\> $uacert = Get-ChildItem -Path 'Cert:\LocalMachine\UA Applications\841048409e5e81af553a6fa599f0d7ea8c2f5c08'
PS C:\> $uacert.IssuerName.Name
CN=UA Configuration Tool, DC=CORP-EU-102
PS C:\>
Get Certificate Info into a CSV by using PowerShell
In PowerShell, use the Get-ChildItem cmdlet to get all certificates and their details or information. Use the Export-CSV cmdlet to export information to the CSV file.
Run the following PowerShell script to get all certificate info into a CSV.
# Get all certificates from the store $mycertificates = Get-ChildItem -Path Cert:\LocalMachine\My\ | Select Thumbprint,NotAfter,SerialNumber # Export certificates information to the CSV file $mycertificates | Export-Csv -Path D:\export_certificates.csv -NoTypeInformation
In the above PowerShell script, the Get-ChildItem uses the Path
parameter to specify LocalMachine\My
certificate store location and retrieves all the certificate and their details and stores them into the $mycertificates
variable.
The $mycertificates variable that stores certificate info passes the output to the Export-CSV cmdlet. The Export-CSV command uses the Path
parameter to specify the destination path to save certificate info into CSV.
Conclusion
I hope, you may find the above article interesting about how to get windows certificate details using Powershell on the local machine or remote computer.
In PowerShell, use the Get-ChildItem Cert:\ drive to get certificate information. As in the above article, you can easily get certificate details, and get certificates on the remote computer.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.