Use the PowerShell String replace() method or replace operator to replace the variable in a file.Use the Get-Content to read the content of the file and pipe it to replace() or replace operator.
The replace() method returns a string where variables in a file are replaced by another variable.
In this article, we will discuss how to use PowerShell replace() method or replace operator to replace the variable in a file.
Use replace() to Replace Variable in File using PowerShell
PowerShell String built-in replace() method takes two arguments; a string to search and a string to replace with the found text.
To read the content of the file, use the PowerShell Get-Content cmdlet and pass the file path to it.
Let’s consider, that a file is having the below text in it and we want to replace the variable in the file with another text in the file.
File Content
$AssemblyVersion=1.0.0.1
$AssemblyTitle = "Calc"
$findStr = '$AssemblyVersion' $replaceStr = '$AssemblyFileVersion' # Read the content of the file and replace string in file (Get-Content -Path D:\PS\calc.txt) | foreach{$_.replace($findStr,$replaceStr)}
The above PowerShell script uses the replace()
method to replace variable $AssemblyVersion by another string $AssemblyFileVersion
$findStr and $replaceStr variable in PowerShell stores the string which needs to be found in the file and replaced with.
PowerShell replace()
takes 2 arguments:
- the substring to search for in the given string, in the above example, $findStr
- the replacement string for the found text, in the above example, $replaceStr
In the above example, the Get-Content cmdlet reads the content of the file and passes it through the pipeline to replace function to replace the text in a file.
The PowerShell replace() method returns a new string where the variable in a file is replaced by the other string variable.
The output of the above script to replace a string in a file display variable name $AssemblyVersion has been replaced to $AssemblyFileVersion
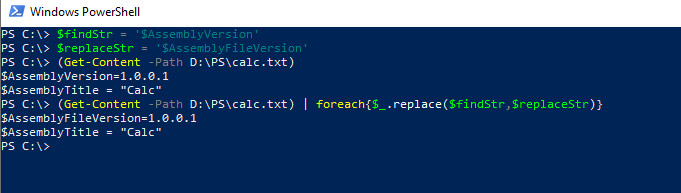
Cool Tip: How to replace space with commas in PowerShell!
Use replace Operator to Replace Variable in File using PowerShell
Using the PowerShell replace operator, you can replace the variable in the file.
Refer to the above example, where the file content is as given below
$AssemblyVersion=1.0.0.1
$AssemblyTitle = "Calc"
We want to replace the $AssemblyVersion variable in the file with another string.
replace operator uses the regex (regular expression) for patterns matching and $ is a special character in regex. Hence to replace the variable having a $ symbol with it, we need to use an escape character before the variable.
The replace operator in PowerShell takes two arguments; string to find for in a string and replacement string for the found text.
# Use the escape character before the special character $ $f = '\$AssemblyVersion' # String to be replaced $r = '$AssemblyFileVersion' # Read the content of the file (Get-Content -Path D:\PS\calc.txt) # Read the content of the file and pipe it replace operator to replace variable in file (Get-Content -Path D:\PS\calc.txt) | foreach{$_ -replace $f,$r}
The above PowerShell script uses the replace operator to replace the variable in a file.
replace operator takes 2 arguments:
- the substring to search for in a string
- the replacement string
In the above example, the Get-Content cmdlet read the content of the file and passes it through the the pipeline to replace operator to replace the variable in the file.
The output of the above Powershell script to replace string in the file is:
PS C:\> $f = '\$AssemblyVersion'
PS C:\> $r = '$AssemblyFileVersion'
PS C:\> (Get-Content -Path D:\PS\calc.txt)
$AssemblyVersion=1.0.0.1
$AssemblyTitle = "Calc"
PS C:\> (Get-Content -Path D:\PS\calc.txt) | foreach{$_ -replace $f,$r}
$AssemblyFileVersion=1.0.0.1
$AssemblyTitle = "Calc"
PS C:\>
Cool Tip: How to do case insensitive replacement in a string using PowerShell!
Conclusion
I hope the above article on how to replace variables in a file using PowerShell replace() method is helpful to you.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.