Use the PowerShell String replace()
method or replace
operator to do the case insensitive replacement in a string. e.g. $str -replace 'hello', 'bye'
do case insensitive replacement in a string.
PowerShell replace() method or replace operator returns a string where the case-insensitive string is replaced by another string.
In this article, we will discuss how to use PowerShell replace() method or replace operator to do case insensitive string replacement.
Use replace() for Case Insensitive Replacement in String
PowerShell String built-in replace() method takes two arguments; a string to search and a string to replace with the found text.
In PowerShell, Replace() method is case-sensitive.
To do case insensitive replacement in a string using replace(), there are a couple of options
- Convert the string to ToUpper() or ToLower() and use replace()
- Use PowerShell 7 version, it uses the System.String class to create an instance and use Replace() method.
# Use the PowerShell version 7 $str = "hello Hello to programming world" # Use the Replace() to do case insensitive replacement $str.Replace('hello','Bye','OrdinalIgnoreCase')
The above PowerShell script uses the replace()
method does the case insensitive replacement in a string.
PowerShell replace()
takes 3 arguments:
- the substring to search for in the given string.
- the replacement string for the found text.
- Use StringComparision that ignores the case example, OrdinalIgnoreCase
The PowerShell replace() method returns a new string where it does all case-insensitive replacements in a string.
The output of the above script to use replace() for case insensitive replacement in a string is:
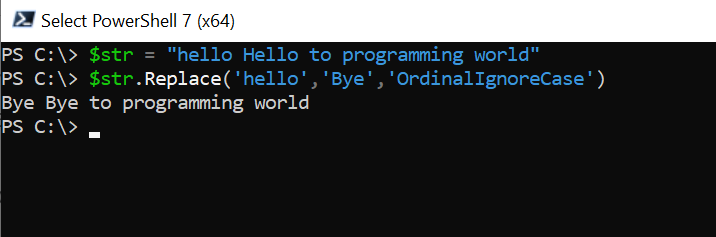
Cool Tip: How to replace the first occurrence of a string in PowerShell!
Use replace Operator to Replace Case Insensitive in String
Using the PowerShell replace operator, you can easily replace case insensitive in a string.
replace operator is case insensitive by default.
The replace operator in PowerShell takes two arguments; string to find for in a string and replacement string for the found text.
$str = "hello Hello to programming world" # Use replace operator for case insensitive replacement in string $str -replace 'hello','Bye'
The above PowerShell script uses the replace operator to do the case insensitive replacement in a string.
replace operator takes 2 arguments:
- the substring to search for in a string.
- the replacement string.
In the above example, replace
operator replaces the ‘hello’ in a string with ‘Bye‘ for all the occurrences regardless of string case ‘hello’
The output of the above Powershell script after case insensitive replacement in a string is:
PS C:\> $str = "hello Hello to programming world"
PS C:\> $str -replace 'hello','Bye'
Bye Bye to programming world
PS C:\>
Cool Tip: How to replace pipe characters in a string using PowerShell!
Conclusion
I hope the above article on how to do case insensitive replacement in a string using PowerShell replace() method and replace operator is helpful to you.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.