PowerShell remove method deletes specified number of characters from the current string based on the beginning at a specified position and returns a new string.
In this article, we will discuss the PowerShell string remove method with various examples.
PowerShell String Remove
PowerShell remove method has two overloads methods.
Overloads
Remove(int startIndex, int count) – Remove a specified number of characters in the current string beginning at a specified position.
StartIndex: The zero-based position to begin deleting characters from the string
count: Specifies the number of characters to delete
It returns the string.
Remove(int startIndex)
startIndex: Specifies the zero-based position to begin deleting characters from the string.
It returns the string after deleting the characters.
Remove First Character from the String in PowerShell
To remove the first character from the string in PowerShell, use the remove() method.
The remove method takes two arguments to remove the first character from the string.
- startIndex: Specify the start index to begin deleting the character. In our case, it should be 0 as we want to delete the first character and the index is zero-based in PowerShell.
- count: Specify the number of characters to remove.
$str = "ShellGeek" # Specify the start index and count to remove first character $str.Remove(0,1)
In the above PowerShell script, the $str variable stores the string data.
To remove the first character from the string, call the PowerShell string Remove() method and pass input arguments as startIndex and count.
The remove () method starts deleting the character at position 0 and count 1 to delete the first character and return the string except for the first character.
The output of the above script in PowerShell to remove the first character in a string delete the first character S from the string ShellGeek.
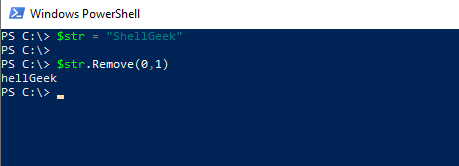
Cool Tip: How to replace a string in PowerShell!
Remove Last Character from the String in PowerShell
Using the remove() method in PowerShell, you can remove the last character from the string.
We can delete the last character from the string using both remove() methods available.
Let’s say, we have a string “ShellGeeks” and we want to remove the last character s from the string.
Refer to the following code where we have used remove() methods to remove the last character.
$str = "ShellGeek" # Get the length of the string $len = $str.Length # To get last character from the string, remove 1 from the lenght $len = $len - 1 # Use the remove() method that accepts startindex and count to remove last character $str.Remove($len,1)
In the above PowerShell script, the $str stores the string “ShellGeeks”.
To remove the last character from the string, follow the below steps:
- Get the length of the string and stores it in variable $len
- To get the last character, subtract 1 from the $len
- Use remove() method that accepts start index as $len and count = 1
The output of the above PowerShell to remove the last character from the string deletes last character s
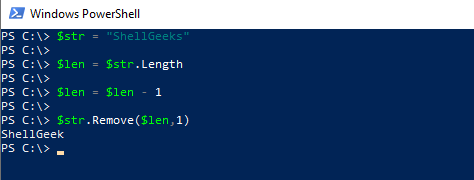
We can remove the last character from the string using the other overload method of remove() that accepts the startIndex
only.
$str = "ShellGeeks" $len = $str.Length $len = $len - 1 $str.Remove($len)
In the above PowerShell script, the remove() method takes a single argument as the start index for the beginning of the deleting character from the string.
The output of the script to delete the last character in a string is:
PS C:\> $str = "ShellGeeks"
PS C:\>
PS C:\> $len = $str.Length
PS C:\>
PS C:\> $len = $len - 1
PS C:\>
PS C:\> $str.Remove($len)
ShellGeek
PS C:\>
Conclusion
I hope the above article on how to use PowerShell String remove method to delete the characters in a string is helpful to you.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.