The `ToBase64String()` method of the class `[System.Convert]` is used to convert binary data into text-based data using Base64 encoding in PowerShell.
Base64 encoding represents binary data in ASCII string format by translating data into Radix 64 representation. Base64 encoding is a group of binary-to-text encoding schemes.
Base 64 encoding is used when we want to transfer data over media or network in ASCII string format so that data remain intact on transport.
In this article, we will discuss how to use PowerShell base64 encoding for images, commands, zip files, and utf8.
Let’s understand base64 encoding in PowerShell with examples.
PowerShell Base64 Encode File Content
Use PowerShell script to take a file as input, and read the contents of the file using the Get-Content cmdlet.
PowerShell base64 encodes the file contents and saves the file to a location with a .64 file format.
$FileName = "D:\Logs-FTP01\EncodeLogic.txt" # Get content of the file $FileContent = Get-Content $FileName #Get the bytes of the file content with encode $fileContentInBytes = [System.Text.Encoding]::UTF8.GetBytes($FileContent) # Base64 Encode file $fileContentEncoded = [System.Convert]::ToBase64String($fileContentInBytes) # Save Base64 Enconde file with extension .b64 $fileContentEncoded | Set-content ($fileName + ".b64") Write-Host $FileName + ".b64" + "File Encoded Successfully!"
In the above PowerShell script to base64 encode file,
$FileName – contains the file path
$FileContent – contains content of the file using the Get-Content cmdlet
$fileContentInBytes – contains bytes about file content, get it using UTF8.GetBytes() method
$fileContentEncoded – contains base64 encode file content using ToBase64String() method which accepts $fileContentsInBytes parameter and convert file to base64.
Using the Set-Content cmdlet, it out base64 encoded file content to file at the specified location.
Cool Tip: How to find large-size files in PowerShell!
PowerShell Base64 Encode String
Let’s understand with an example of a base64 encoded string in PowerShell.
For example, if you want to base64 encode a string, use the below script
$StringMsg = "PowerShell Base64 Encode Example" # Gets the bytes of String $StringBytes = [System.Text.Encoding]::Unicode.GetBytes($StringMsg) # Encode string content to Base64 string $EncodedString =[Convert]::ToBase64String($StringBytes) Write-Host "Encode String: " $EncodedString
In the above PowerShell script to convert string to base64,
$StringMsg – variable contains string content
$StringBytes – variable contains Bytes for a given string using Unicode.GetBytes()
Using the ToBase64String() method, it converts bytes to Base64 encoded string and prints it on the console.
You can use FromBase64String() method to convert from base64 encoded string.
$DecodedString = [System.Text.Encoding]::Unicode.GetString([System.Convert]::FromBase64String($EncodedString)) Write-Output $DecodedString
In the above PowerShell script to decode the base64 string,
It uses [System. Convert]:: FromBase64String method which accepts base64 encodes string as an input parameter.
Using [System.Text.Encode]::Unicode.GetString() method converts from base64 string in PowerShell.
The output of the above command is:
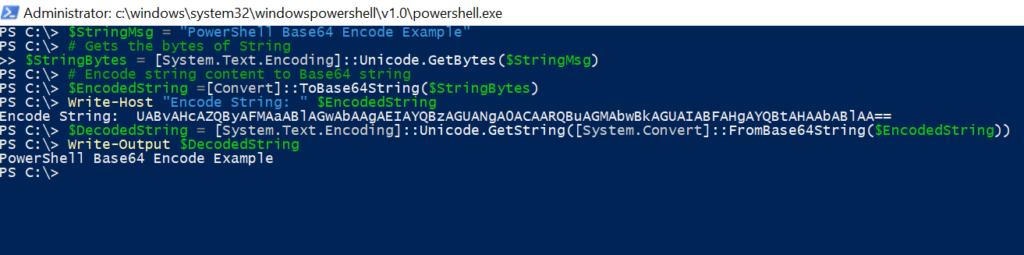
PowerShell Base64 Encode Image
You can easily convert the image to base64 encoded string as below
[convert]::ToBase64String((Get-Content D:\Logs-FTP01\powershell-mkdir.png -Encoding byte))
In the above script, the PowerShell Base64 Encoding converts the image to the Base64 string.
PowerShell Get-Content cmdlet read the image file contents and converts the image to base64 string.
Cool Tip: How to use the net user command in the command prompt!
PowerShell Base64 Encode zip file
If you want to base64 encode a zip file, use below PowerShell script
$zipfile = "D:\Logs-FTP01\EncodeLogic.zip" $zipfilebytes = Get-Content $zipfile -Encoding byte $zipfileBytesBase64 = [System.Convert]::ToBase64String($zipfilebytes) $zipfileBytesBase64 | Out-File 'D:\Logs-FTP01\EncodeLogic.txt'
In the above PowerShell script,
$zipFile – contains zip file path
$zipfilebytes – contains the bytes information, Get-Content cmdlet read zip file content
$zipfileBytesBase64 – variable contains Base64 encoded string for the zip file.
Base64 encodes zip file contents out to a .txt file as the path specified.
Cool Tip: How to list files in a directory in PowerShell!
Conclusion
In the above article, I have explained about PowerShell base64 encode for image, string, file content or base64 encode zip file.
Using [System.Convert].ToBase64String in PowerShell, you can convert the file to base64, and convert the string to base64 encoded string.
You can read more about using PowerShell mkdir to create a new directory or New-Item cmdlet to create a directory if not exists and pass multiple parameters to function to create a directory.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.