Use the GetString()
method of the UTF8
encoding format in PowerShell to convert byte array to string. This method returns a string.
[System.Text.Encoding] class provides methods to convert strings to and from using different encoding formats.
In this article, we will discuss how to use GetString() method in PowerShell to convert byte array to string.
Convert Byte Array to String in PowerShell using GetString() Method
Use GetString()
method of [System.Text.Encoding]
class in PowerShell to convert byte array to string.
Let’s understand with the help of an example to get a byte array and use it to convert it to a string.
# Declare string variable $str = "ShellGeek" # converting string to bytes array $bytesArr = [System.Text.Encoding]::UTF8.GetBytes($str) # Print the bytes array $bytesArr # Convert byte to string $str2 = [System.Text.Encoding]::UTF8.GetString($bytesArr) # Print the string $str2
In the above PowerShell script, the $str
variable is a string type containing the value “ShellGeek”. Using the GetBytes() method, it converts the string to a byte array and assigns the result to the variable $bytesArr
that contains the byte array.
To convert a byte array to a string, it uses GetString() method that takes a byte array as an input parameter and returns a string
The output of the above script in PowerShell converts the byte array to a string using the GetString() method [System.Text.Encoding]
class is:
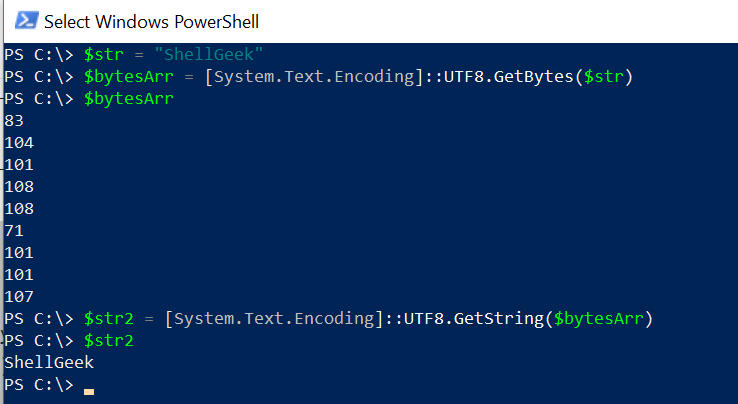
Cool Tip: How to convert string to integer in PowerShell!
Convert Byte Array to String in PowerShell
Use [System.Text.Encoding]
class that provides the method GetString() to decode binary data into a string using a specified encoding. It provides a set of methods for encoding and decoding text in various formats.
# Create a byte array $bytesArr = [byte[]]@(0x48, 0x65, 0x6C, 0x6C, 0x6F, 0x20, 0x57, 0x6F, 0x72, 0x6C, 0x64) # Convert byte array to string $str3 = [System.Text.Encoding]::UTF8.GetString($bytesArr) # Print the string $str3
In the above PowerShell script, the $bytesArr
variable contains the bytes array. System.Text.Encoding
class has GetString() method in PowerShell to convert a byte array to a string. This method takes an input parameter as a byte array and returns a string.
The output of the script decodes binary data into a string using the UTF8 encoding format as follows:
# Create a byte array
PS C:\> $bytesArr = [byte[]]@(0x48, 0x65, 0x6C, 0x6C, 0x6F, 0x20, 0x57, 0x6F, 0x72, 0x6C, 0x64)
# Convert byte array to string
PS C:\> $str3 = [System.Text.Encoding]::UTF8.GetString($bytesArr)
# Print the string
PS C:\> $str3
PS C:\> Hello World
Cool Tip: How to convert byte array to hex string in PowerShell!
Conclusion
I hope the above article on how to convert byte array to string in PowerShell using the GetString() method of UTF8 encoding format is helpful to you.
PowerShell strings are sequences of characters while the bytes are sequences of binary data. System.Text.Encoding
class provides methods to convert string to bytes and convert bytes to string using the ToString() method of UTF8 encoding format.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.