PowerShell is a versatile scripting language that provides extensive support for handling command line arguments, including named and position parameters, and special parameter types like switch, array, and $Args.
In this article, we will discuss how to work with PowerShell command line arguments.
Basics of PowerShell Command Syntax
PowerShell supports both named and positional parameters. Named parameters are identified by their specific name, and positional parameters are identified by their order.
The below examples illustrate defining both types in PowerShell script:
# Create scrip1.ps1 file having parameters of different data types param( [string]$Subject, [int]$Marks ) Write-Host "Tom scored $Marks in subject $Subject."
In the above example, the $Subject and $Marks are named parameters. Let’s use them when running the script:
D:\PS\script1.ps1 -Subject "Physics" -Marks 74
Pass Parameters to a PowerShell Script
Let’s understand with the help of an example to use named and positional parameters when running a script file.
Using named parameters
Named parameters make it easier to read and understand while using them within a script. To use named parameters in a PowerShell script, provide them explicitly:
D:\PS\script1.ps1 -Subject "Physics" -Marks 74
In the above PowerShell script, when running a script file, it requires two parameters like Subject and Marks. We have provided these parameters explicitly with their values in a script.
While using the named parameters in a script, the parameter order doesn’t important, providing the correct parameter name is important.
The output of the above PowerShell script is:
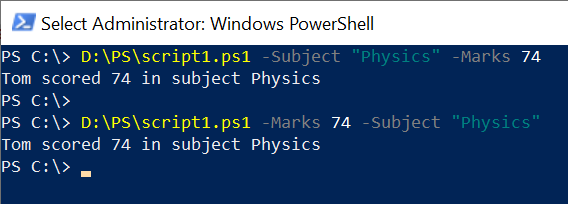
Using positional parameters
Positional parameters in the PowerShell script allow you to pass the values without specifying the parameter name, you must provide the values in the order that the parameters are defined in the script.
D:\PS\script1.ps1 "Physics" 74
In the above PowerShell script, we have provided the parameter values in the order that they are defined in the script file.
Switch Parameter Command Line Argument
Switch
parameters are used when you need a on/off
switch in your script. They don’t require a value but are either true or false.
# Create script1.ps1 file having switch parameter param( [string]$Subject, [int]$Marks, [switch]$Verbose ) Write-Host "Tom scored $Marks in subject $Subject" Write-Host "The True/False switch argument is $Verbose"
To use the switch parameter, use it when calling the script:
# Without switch parameter, it print the value as False D:\PS\script1.ps1 -Subject "Physics" -Marks 74 # Include switch parameter when calling a script D:\PS\script1.ps1 -Subject "Physics" -Marks 74 -Verbose
In the above PowerShell script, if the switch
parameter included in the command line argument, it will print True, else displays the output as False.
The output of the above PowerShell script is:
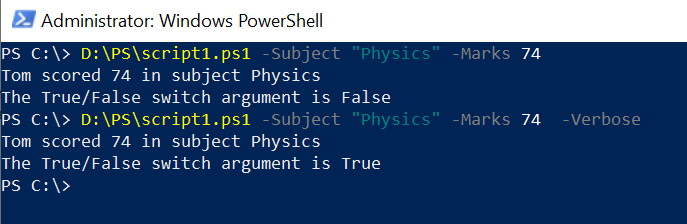
Array Parameter in Command Line Argument
Array parameters allow you to pass the array ( multiple values) to a single parameter in the command line argument.
# Create script1.ps1 file having Subjects parameter of type string[] param( [string[]]$Subjects ) Write-Host "Subjects passed as an array are: $Subjects"
To use an array parameter in a command line argument, pass multiple values to the $Subjects parameter using a comma-separated list.
D:\PS\script1.ps1 -Subjects "Maths","Physics","English"
The output of the above PowerShell script is:
PS C:\> D:\PS\script1.ps1 -Subjects "Maths","Physics","English" Subjects passed as array: Maths Physics English
PS C:\>
Validation Attributes in Command Line Argument
Validation attributes in the PowerShell script allow you to enforce specific restrictions on parameters.
# Create a script1.ps1 file having validation range defined for parameter. param( [ValidateRange(1, 100)][int]$Marks ) Write-Host "$Marks is between 1 to 100" -ErrorAction SilentlyContinue
In the above PowerShell script, the $Marks parameter must be between 1 to 100.
To pass the value of the parameter between the given range and outside of the range.
# Pass the Marks value in between 1 to 100 D:\PS\script1.ps1 -Marks 25 # Pass the Marks value greater than 100 D:\PS\script1.ps1 -Marks 101
In the above PowerShell script, the -Marks
parameter value between 1 to 100 will display the result as follows:
PS C:\> D:\PS\script1.ps1 -Marks 25 25 is between 1 to 100
-Marks parameter value greater than 100 will show an exception because the provided value is outside the validation range.
PS C:\> D:\PS\script1.ps1 -Marks 101 D:\PS\script1.ps1 : Cannot validate argument on parameter 'Marks'. The 101 argument is greater than the maximum
allowed range of 100. Supply an argument that is less than or equal to 100 and then try the command again.
At line:1 char:26
+ D:\PS\script1.ps1 -Marks 101
+ ~~~
+ CategoryInfo : InvalidData: (:) [script1.ps1], ParameterBindingValidationException
+ FullyQualifiedErrorId : ParameterArgumentValidationError,script1.ps1
Using $Args Automatic Variable in Command Line Argument
The $Args
is an automatic variable that contains an array of all the arguments passed to the script.
To use the $Args
variable to access and manipulate the script’s arguments use the following script:
foreach ($arg in $Args) { Write-Host $arg }
Running PowerShell Scripts with Parameters from CMD
To run a PowerShell script with parameters from cmd, use the following command:
powershell -ExecutionPolicy Bypass -File "D:\PS\script1.ps1" -Marks 74 -Subject "Physics" -Verbose
The output of the above command displays the result as follows:
C:\>powershell -ExecutionPolicy Bypass -File "D:\PS\script1.ps1" -Marks 74 -Subject "Physics" -Verbose
Tom scored 74 in subject Physics
The True/False switch argument is True
You can learn more about how to run the PowerShell script from cmd with different methods.
Conclusion
I hope the above article on how to work with PowerShell command line arguments is helpful to you. We have covered the topic command line arguments in-depth including named, positional parameters, switch, array parameters and $Args to access the argument passed to the script.