To read file line by line in the Windows PowerShell, use Get-Content to read the content of the text file and then process each line within a ‘For each’ loop. You can also use the .NET library class [System.IO.File] method ReadLine() to read a text file.
While working on the files, you need to read the file line by line and store the line in the variable for further processing. This can be easily achieved using the Windows PowerShell commands.
In this article, we will discuss how to read file line by line in Windows PowerShell using the Get-Content and foreach line in a text file to process it.
Read the File line by line using [System.IO.File]
.Net library includes the [System.IO.File] class which has the ReadLines()
method. This method takes a text file path as input and reads the file line by line.
foreach($line in [System.IO.File]::ReadLines("D:\LogTest\FTP-02\Environement-Variable.txt")) { Write-Output $line }
In the above PowerShell script, the ReadLine() function reads a text file and uses the foreach loop to read the file line by line and pass it to the further for processing.
The output of the above PowerShell script reads the entire file content line by line and prints it on the terminal as below:
What is an environment variable in Windows? How to print environment variables in PowerShell?
The Environment variables stores information about operating system environment information and programs.
This information details include the operating system path, location of the windows installation directory, and a number of p
rocesses used by the operating system.
PowerShell can access and manage or change environment variables.
The Environment variable in Windows operating system stores that is used by programs.
Using PowerShell, we can print environment variables on the console.
Cool Tip: How to rename the part of the file name using PowerShell!
Read File line by line using Get-Content
The Get-Content cmdlet in PowerShell is used to read the contents of the specified item.
Using the Get-Content command, we can specify the file path to read the file content. To process each line individually, we can use the foreach loop in PowerShell. This allows us to perform the necessary operations on each line from the file.
$regex = '' foreach($line in Get-Content -Path D:\LogTest\FTP-02\Environement-Variable.txt) { if($line -match $regex) { Write-Output $line } }
In the above PowerShell script, the $regex
variable contains a regular expression to get the specific lines from the file. In this example, we will use the regex value as ”.
The above script uses the Get-Content command to read a text file and process each line using the foreach loop in PowerShell. It reads the file line by line and store content in the $line
variable.
Use the if statement in PowerShell to check for the line with the regex expression and if the regular expression matches with the line then print the line.
The output of the above PowerShell to read file line by line using the Get-Content command is:
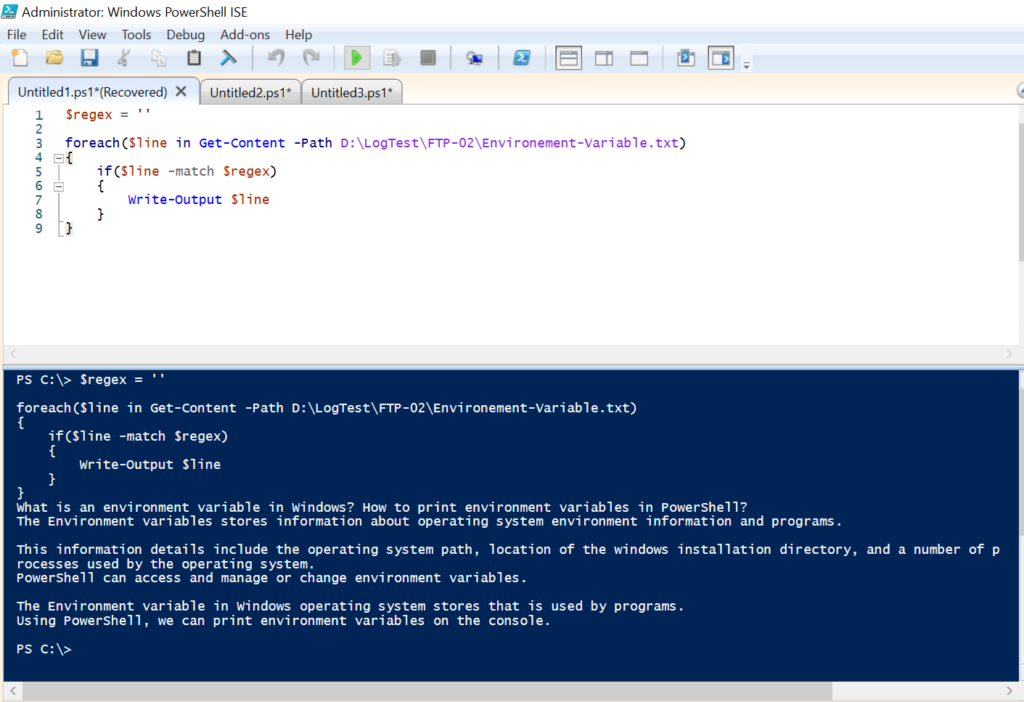
Cool Tip: How to get specific lines of the file using PowerShell!
If you want the specific lines to be read from the file, provide the custom regex value. For example, if you want to get lines from the file that starts with “The“, run the following command.
$regex = '^The' foreach($line in Get-Content -Path D:\LogTest\FTP-02\Environement-Variable.txt) { if($line -match $regex) { Write-Output $line } }
In the above PowerShell script, we have specified the regex value as “^The”.
Using the foreach loop in PowerShell, it read the file line by line and passes the line to the if statement to match against the regex expression.
If the regex expression matches the content of the file, in our case the line should start from “The”, it will evaluate to true and print the line.
The output of the above PowerShell script will read the file and print lines that match the specified regex.
The Environment variables stores information about operating system environment information and programs.
The Environment variable in Windows operating system stores that is used by programs.
Cool Tip: How to count lines in the file using PowerShell!
Use Switch to Read File Line by Line
The Switch statement in the PowerShell has Regex and File parameters. Using the File parameter, we can provide the file name for reading and Regex performs the regular expression matching of the value to the condition.
$regex = '^The' switch -regex -File Environement-Variable.txt{ $regex { "$_" } }
In the above PowerShell script, we have the Environment-Variable.txt file stored in the computer system. We have specified the custom regex value as “The”.
Using the switch statement in the PowerShell, it uses the File parameter to read the file content line by line and the regex parameter to match the value of the line to the condition that the line should start with “The”
If the regex conditions evaluate to trues, it will print the line as below.
The Environment variables stores information about operating system environment information and programs.
The Environment variable in Windows operating system stores that is used by programs.
Cool Tip: How to get the last line of the file using PowerShell!
Conclusion
I hope the above article on how to read file line by line in PowerShell is helpful to you.
The Get-Content cmdlet in the PowerShell reads the content at once, it may cause issues or freeze/ not respond if the file size is large. You can consider using any of the above three different ways to read file content.
You can use Import-CSV to import CSV files and read csv file line by line in PowerShell.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.