SSL ( Secure Sockets Layer) is a digital certificate that provides an encrypted connection between server and client and authenticates a website identity. To keep user-sensitive data secure, and provide trust to users, it is very important to check SSL certificate expiration and renew them if they are due.
In PowerShell, use [Net.HttpWebRequest]
to make the HTTP web request to the website and get all properties associated with it, and certificate details. It will help to find the SSL certificate expiration date.
In this article, we will discuss how to get the SSL certificate expiration date in PowerShell.
PowerShell Get SSL Certificate Expiration Date
.Net library provides [System.Net.ServicePoint] library to manage the collections of ServicePoint objects. ServicePointManager returns the ServicePoint object that contains the information about the internet resource URI.
Use ServicePointManager.ServerCertificateValidationCallBack property to get or sets the callback to validate the server certificate.
Use [Net.HttpWebRequest] library to get SSL certificate details about the website and extract the SSL certificate expiration date using the PowerShell script.
Run the following Windows PowerShell script to check the SSL certificate expiration date.
# Ignore SSL Warning [Net.ServicePointManager]::ServerCertificateValidationCallback = { $true } # Create Web Http request to URI $uri = "https://google.com/" $webRequest = [Net.HttpWebRequest]::Create($uri) # Get URL Information $webRequest.ServicePoint # Retrieve the Information for URI $webRequest.GetResponse() | Out-NULL # Get SSL Certificate and its details $webRequest.ServicePoint.Certificate # Get SSL Certificate Expiration Date $webRequest.ServicePoint.Certificate.GetExpirationDateString()
In the above PowerShell script, it uses [Net.HttpWebRequest] to create HTTP web requests to website URI and retrieve the URI properties like Address, ConnectionName, Certificate, etc… in the $webRequest variable.
$webRequest.GetResponse()
retrieves the response for the URI and fetches SSL certificate details, Server, Headers, and other relevant information.
$webRequest.ServicePoint.Certificate
gets the certificate details like issuer, Handle, and SSL certificate thumbprint. Use the GetExpirationDateString()
method to check the SSL expiration date for a website using PowerShell.
The output of the above PowerShell script to find the SSL certificate expiration date for the website is:
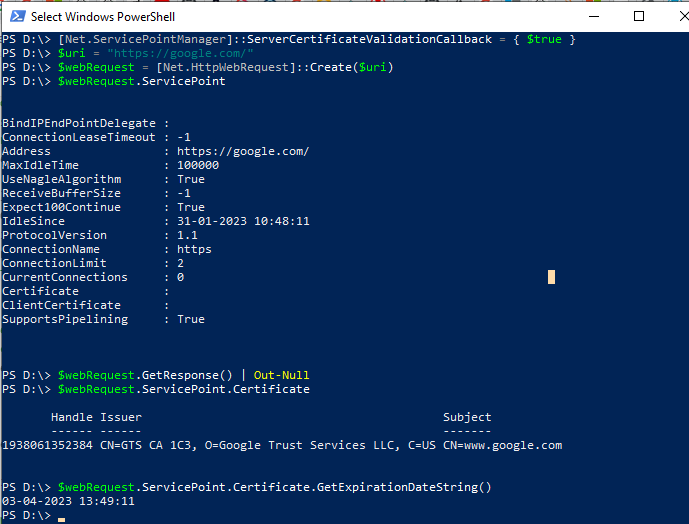
PS D:\> $webRequest.GetResponse()
IsMutuallyAuthenticated : False
Cookies : {}
Headers : {X-XSS-Protection, X-Frame-Options, Cache-Control, Content-Type...}
SupportsHeaders : True
ContentLength : -1
ContentEncoding :
ContentType : text/html; charset=ISO-8859-1
CharacterSet : ISO-8859-1
Server : gws
LastModified : 31-01-2023 10:52:14
StatusCode : OK
StatusDescription : OK
ProtocolVersion : 1.1
ResponseUri : https://www.google.com/
Method : GET
IsFromCache : False
PS D:\> $webRequest.ServicePoint.Certificate.GetExpirationDateString()
03-04-2023 13:49:11
PS D:\>
Cool Tip: How to get certificate details in PowerShell!
Conclusion
I hope the above article on how to check SSL certificate expiration date in PowerShell is helpful to you.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.