Use the [Net.HttpWebRequest] library to create a connection to the website URI and GetResponse() that contains SSL certificate information like Handle, Issuer, Subject, Expiration Date, etc…
In this article, we will discuss how to retrieve SSL certificate details from a website in PowerShell.
PowerShell Get SSL Certificate From URL
Use the [Net.HttpWebRequest] library that has Create() method. It accepts the website URL as an input parameter and creates a web request connection to the website.
Use GetResponse() method to get the SSL certificate details from the URL.
[Net.ServicePointManager]::ServerCertificateValidationCallback = { $true } is used to ignore SSL certificate errors or warning while making the webrequest to the URI.
Run the following PowerShell script to retrieve the SSL certificate from the website URL.
#Ignore SSL Warning [Net.ServicePointManager]::ServerCertificateValidationCallback = { $true } # Create Web Http request to URI $uri = "https://google.com/" $webRequest = [Net.HttpWebRequest]::Create($uri) # Get URL Information $webRequest.ServicePoint # Retrieve the Information for URI $webRequest.GetResponse() | Out-NULL # Get SSL Certificate information $webRequest.ServicePoint.Certificate
In the above PowerShell script, it uses [Net.HttpWebRequest] to create HTTP web requests to website URI and retrieve the URI properties like Address, ConnectionName, Certificate, etc… in the $webRequest variable.
$webRequest.GetResponse()
retrieves the response for the URI and fetches certificate details, Server, Headers, and other relevant information.
$webRequest.ServicePoint.Certificate
gets the certificate details like issuer, Handle, and SSL certificate thumbprint.
The output of the above PowerShell script to get the SSL certificate details from the URL is:
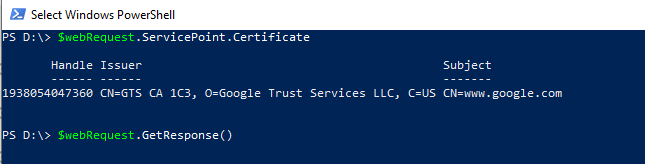
PowerShell Get SSL Certificate Issuer Name
The SSL certificate has the Issuer property or GetIssuerName() method to retrieve the SSL certificate issuer name.
Run the following PowerShell script to obtain the SSL certificate issuer name, refer to the above PowerShell script to make webrequest to the website and get the SSL certificate details.
#Ignore SSL Warning [Net.ServicePointManager]::ServerCertificateValidationCallback = { $true } # Create Web Http request to URI $uri = "https://google.com/" $webRequest = [Net.HttpWebRequest]::Create($uri) # Get URL Information $webRequest.ServicePoint # Retrieve the Information for URI $webRequest.GetResponse() | Out-NULL # Get SSL Certificate Issuer Name $webRequest.ServicePoint.Certificate | Select Issuer # Use GetIssuerName() to obtain SSL certificate issuer name $webRequest.ServicePoint.Certificate.GetIssuerName()
The output of the above PowerShell script retrieves the SSL certificate issuer name as given below:
PS D:\> $webRequest.ServicePoint.Certificate | Select Issuer
Issuer
------
CN=GTS CA 1C3, O=Google Trust Services LLC, C=US
PS D:\> $webRequest.ServicePoint.Certificate.GetIssuerName() C=US, O=Google Trust Services LLC, CN=GTS CA 1C3
Find SSL Certificate Subject Name
The SSL certificate has a Subject property and GetName() method which is used to get the SSL certificate subject name.
Refer to the above PowerShell script that makes webrequest to the website and gets SSL certificate information.
# Get SSL Certificate Subject Name $webRequest.ServicePoint.Certificate | Select Subject # Use GetName() to obtain SSL certificate Subject name $webRequest.ServicePoint.Certificate.GetName()
The above PowerShell script uses the Subject property to retrieve the subject name of the SSL certificate. An alternate way is to use GetName() method to obtain the SSL certificate subject name.
The output of the above script is:
PS D:\> $webRequest.ServicePoint.Certificate | Select Subject
Subject
-------
CN=www.google.com
PS D:\> $webRequest.ServicePoint.Certificate.GetName() CN=www.google.com
Find SSL Certificate Serial Number
Use the GetSerialNumberString()
method of the SSL certificate to get the SSL certificate serial number.
Refer to the above PowerShell script that makes webrequest to the URL and gets certificate details.
# Use GetName() to obtain SSL certificate SerialNumber $webRequest.ServicePoint.Certificate.GetSerialNumberString()
The above PowerShell script uses the GetSerialNumberString() method to obtain the SSL certificate serial number.
The output of the above script is:
PS D:\> $webRequest.ServicePoint.Certificate.GetSerialNumberString() 229AE03988E214FE0A531413A2A103BE
Cool Tip: How to check SSL certificate expiration date in PowerShell!
Conclusion
I hope the above article on how to get SSL certificate information in PowerShell is helpful to you.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.