Office 365 and Azure have a requirement to authenticate users with upn, as an administrator, you have to make sure user UPN same and matches with on-premise user upn. If it is not you have to change upn suffix for all the users in the active directory.
In this article, I will explain how to change UPN suffix for a single user or multiple users with PowerShell.
PowerShell Get-AdUser cmdlets get active directory users specified by filter and search condition.
Get UPN Suffix in Active Directory
Before we change UPN suffix, we first need to find UPN suffixes in an active directory forest.
Get UPN suffix using Get-ADForest
Use PowerShell Get-ADForest
cmdlet to get active directory forest information, querying for userprincipalname, it returns upn suffixes in active directory.
Get-ADForest | Format-List UPNSuffixes
In the above PowerShell script, it will returns the list of UPN suffixes in the active directory forest. The UPN suffixes are created in Active Directory Domains and Trusts.
The output of above command to get UPN suffixes in an active directory as below
PS C:\Windows\system32> Get-ADForest | Format-List UPNSuffixes
UPNSuffixes : {SHELLPRO.LOCAL}
also, get UPN suffix for all active directory users using Get-AdUser cmdlet as below
Get-ADUser -Filter * | Sort-Object Name | Format-Table Name, UserPrincipalName
above PowerShell script will get a list of all active directory users name and their userprincipalname (UPN) as below
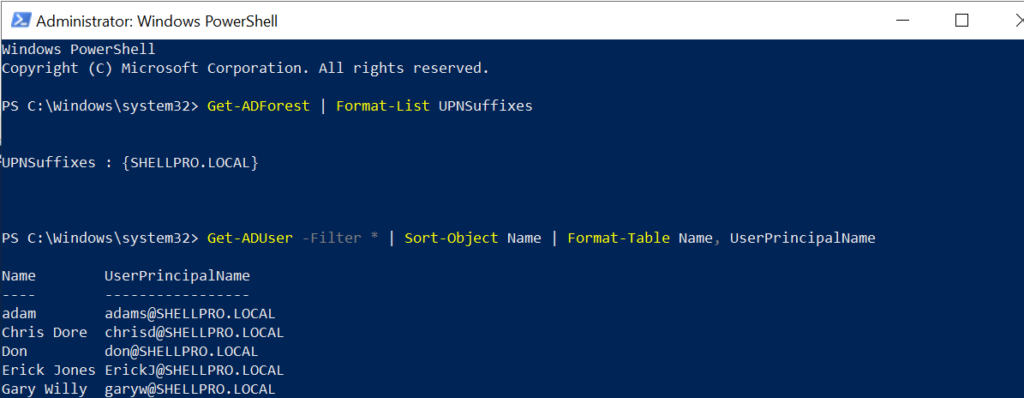
Change UPN Suffix in Active Directory
As in the above command, we get active directory users having upn suffix as ‘SHELLPRO.LOCAL’
To change UPN suffix in active directory with PowerShell, run below command
$OldUPNDetails = Get-ADUser -Filter {UserPrincipalName -like '*SHELLPRO.LOCAL'} -Properties UserPrincipalName $OldUPNDetails | foreach {$NewUpn = $_.UserPrincipalName.Replace("SHELLPRO.LOCAL","shellpro.com"); $_ | Set-ADUser -UserPrincipalName $NewUpn}
In the above PowerShell script,
$OldUPNDetails variable contains the list of active directory users retrieved using Get-ADUser filter where Userprincipalname
like SHELLPRO.LOCAL
In the second command, iterate over $OldUPNDetails
using foreach
to replace old upn with new upn and using Set-ADUser cmdlet to change upn suffix for users in active directory.
The output of above change upn suffix in active directory, after we again run Get-AdUser
command to get aduser name and userprincipalname as below:

Change UPN suffix for User in OU
If you want to change UPN suffix for users in a specified organizational unit (OU), you can easily do it using Get-AdUser SearchBase parameter to get specified OU users.
Once we get a list of active directory users from specific OU, let’s run a command to iterate over each aduser and change upn suffix with Set-ADUser
cmdlet.
For example, let’s consider an example to change UPN suffix for users in Sales OU, use below command to get all users in Sales OU as below
Get-ADUser -Filter * -SearchBase "OU=SALES,DC=SHELLPRO,DC=local" | Format-Table Name, UserPrincipalName
The output of above command as below
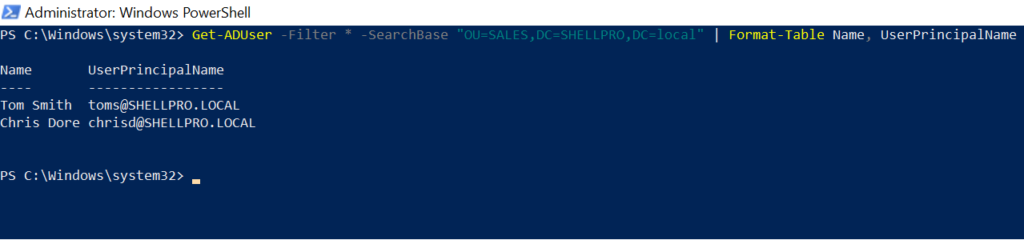
Now, as we have a list of all users with their old UPN suffix (SHELLPRO.LOCAL), run the below command to change UPN suffix for user
$OldUpnUsers | foreach {$NewUpn = $_.UserPrincipalName.Replace("SHELLPRO.LOCAL","shellpro.com"); $_ | Set-ADUser -UserPrincipalName $NewUpn}
In the above PowerShell script, it will iterate over $OldUpnUsers
using foreach
to replace old upn suffix (SHELLPRO.LOCAL) to new upn suffix (shellpro.com) and pass output to the second command.
The second command uses Set-ADUser
cmdlet to change upn suffix for users from specific OU.
If we use Get-AdUser
cmdlet to get users from specific Sales OU, results are as below

Cool Tip: How to create organizational unit (OU) in PowerShell!
Conclusion
In the above article, we have learned that how to get upn suffixes in active directory and change upn suffix for users in active directory forest or change upn suffix for users in OU with PowerShell.
You can read here about how to add userprincipalname suffix in active directory.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on ShellGeek home page.