Very often Admin has to update the Active Directory user’s properties manually. This process can be time-consuming. PowerShell Active Directory module provides Set-AdUser cmdlet to modify active directory user’s attributes.
PowerShell Set-AdUser cmdlet modifies active directory user attributes. It allows us to modify commonly used user properties using cmdlet parameters. Identity parameter to get specific active directory user to modify properties.
You can identify a user by GUID, Distinguished Name, SAMAccountName, or Security Identifier (SID). You can use the Get-AdUser cmdlet to retrieve user objects and pass objects through the pipe (|) operator to Set-AdUser cmdlet to modify user attributes.
Prerequisites
The Set-AdUser cmdlet is one of the Active Directory cmdlets. To use the set-aduser cmdlet, the system needs to have the following requirements:
- PowerShell
ActiveDirectory
Module to be installed - Users with administrator access or have enough access to read Active Directory information.
Tip: To know which modules are available in the system, run the below command in PowerShell ISE
Get-Module -ListAvailable
This command returns all the modules installed and available in the system. If the Active Directory module is not available then follow the Active Directory installation steps.
Syntax
Set-AdUser cmdlet modifies an active directory user properties
Set-ADUser [-WhatIf] [-Confirm] [-AccountExpirationDate <DateTime>] [-AccountNotDelegated <Boolean>] [-Add <Hashtable>] [-AllowReversiblePasswordEncryption <Boolean>] [-AuthenticationPolicy <ADAuthenticationPolicy>] [-AuthenticationPolicySilo <ADAuthenticationPolicySilo>] [-AuthType <ADAuthType>] [-CannotChangePassword <Boolean>] [-Certificates <Hashtable>] [-ChangePasswordAtLogon <Boolean>] [-City <String>] [-Clear <String[]>] [-Company <String>] [-CompoundIdentitySupported <Boolean>] [-Country <String>] [-Credential <PSCredential>] [-Department <String>] [-Description <String>] [-DisplayName <String>] [-Division <String>] [-EmailAddress <String>] [-EmployeeID <String>] [-EmployeeNumber <String>] [-Enabled <Boolean>] [-Fax <String>] [-GivenName <String>] [-HomeDirectory <String>] [-HomeDrive <String>] [-HomePage <String>] [-HomePhone <String>] [-Identity] <ADUser> [-Initials <String>] [-KerberosEncryptionType <ADKerberosEncryptionType>] [-LogonWorkstations <String>] [-Manager <ADUser>] [-MobilePhone <String>] [-Office <String>] [-OfficePhone <String>] [-Organization <String>] [-OtherName <String>] [-Partition <String>] [-PassThru] [-PasswordNeverExpires <Boolean>] [-PasswordNotRequired <Boolean>] [-POBox <String>] [-PostalCode <String>] [-PrincipalsAllowedToDelegateToAccount <ADPrincipal[]>] [-ProfilePath <String>] [-Remove <Hashtable>] [-Replace <Hashtable>] [-SamAccountName <String>] [-ScriptPath <String>] [-Server <String>] [-ServicePrincipalNames <Hashtable>] [-SmartcardLogonRequired <Boolean>] [-State <String>] [-StreetAddress <String>] [-Surname <String>] [-Title <String>] [-TrustedForDelegation <Boolean>] [-UserPrincipalName <String>] [<CommonParameters>]
Set-ADUser Examples
Let’s see below Set-AdUser examples to set aduser attributes, email address, set-aduser manager, and so on.
Use Set-Aduser to set aduser email address
To set an active directory user email address, use the PowerShell Set-AdUser cmdlet to update the EmailAddress attribute of the aduser.
Set-ADUser -Identity smith -EmailAddress '[email protected]'
In the above example, PowerShell Set-ADUser updates the user’s “smith” email address in the active directory account.
The Set-ADUser
command uses the -Identity
parameter to identify a user based on a distinguished name, in this case, “smith” and the EmailAddress
parameter to specify the email address which needs to be updated for the given user, in this case, “[email protected]“.
Cool Tip: How to get email address using Get-ADUser in PowerShell!
Set Active Directory User Job Title and Department Attributes
You can use the Set-AdUser cmdlet in PowerShell to update multiple attributes.
Set-ADUser -Identity "smith" -Department "Asia_Sales" -Title "Manager"
In the above example, the Set-ADUser command sets job, title, and department properties to the user object with the SAM account named “smith”. The Set-AdUser command uses the Department attribute to set the department name and the Title
attribute to set the title of the user.
Set Active Directory User HomePage Attribute
Set-ADUser smith -HomePage 'https://shellgeek.com/powershell/' -LogonWorkstations 'it-20-dekstop,it-20-laptop'
In the above example, the Set-ADUser command replaces the SAM account named “smith” user HomePage attribute to https://shellgeek.com/powershell/ and LogonWorkstations
property to it-20-desktop and it-20-laptop.
Get AD User and Set ADduser Manager property
Let’s consider an example to set aduser Smith manager attribute. The Set-AdUser
has a manager attribute that is used to set an active directory user manager.
Get-ADUser -Identity "Smith" | Set-ADUser -Manager "JohnKelly"
In the above example, it gets active directory user Smith using PowerShell Get-ADUser and passes through the user object to the PowerShell Set-ADUser
cmdlet to update the attribute Manager
in the case “JohnKelly“. You can get an ad user using userprincipalname or upn.
PowerShell Set-AdUser Attributes for Multiple Users
Get-ADUser -Filter 'Name -like "*"' -SearchBase 'OU=Sales,OU=UserAccounts,DC=shellgeek,DC=COM' -Properties DisplayName | % {Set-ADUser $_ -DisplayName ($_.Surname + ' ' + $_.GivenName)}
In the above example, the Get-ADUser cmdlet gets a list of all users that are located in OU=Sales,OU=UserAccounts,DC=shellgeek,DC=COM
organization unit.
PowerShell Set-ADUser cmdlet updates the DisplayName
attribute on user objects to the concatenation of the Surname
property and GivenName property.
PowerShell Set Ad Users Attributes from Csv
Let’s consider an example, to update AD user multiple attributes like ad user title and department from a CSV file, run the below command.
Import-Module ActiveDirectory $users = Import-csv -Path c:\powershell\ad_users.csv foreach ($user in $users) { Get-ADUser -Filter "employeeID -eq '$($user.employeeID)'" -Properties * -SearchBase "OU=Asia_Sales,dc=shellgeek,dc=com" |Set-ADUser -department $($user.department) -title $($user.title) }
In the above example, the ad_users.csv file contains the employeeID, title, and department. User Import-CSV to read a CSV file and store file data in $users
variable.
Iterate over $user to get a user from the active directory in a specific OU using Get-AdUser
cmdlet. Use retrieved employee user object and pass-through pipeline (|) to Set-AdUser
cmdlet to update department and title.
Cool Tip: Learn how to get ad user attributes from csv using the Get-ADUser cmdlet
Set-ADUser Update Multiple Attributes
PowerShell Set-ADUser replaces the specific values for an object property with current values. Use LDAP display name to modify object property. You can use Set-ADUser replace to update multiple attribute values.
Let’s consider a case to update the title and email address of an employee, using set-ADuser replace, We can do it as below.
Set-ADUser -Identity Smith -Replace @{title="manager";mail="[email protected]"}
This command updates the aduser named “Smith” title as manager and email address to [email protected].
Set-AdUser Company name
To set the aduser company name for active directory users in a specific OU, run the below command.
Get-ADUser -SearchBase "OU=SALES,DC=SHELLPRO,DC=LOCAL" -filter * | Set-ADUser -Replace @{company="SHELLGEEK"}
In the above PowerShell script, the Get-AdUser uses the SearchBase
parameter to get active directory users in the specified OU and the Filter *
parameter to retrieve all the user objects from the specified OU and pipes the output to the second command.
The second command uses the Set-ADUser to update the company name with the provided value as specified by the company attribute in this case “SHELLGEEK“.
Cool Tip: Learn how to get an ad user using userprincipalname or upn in PowerShell!
PowerShell Set-AdUser Replace Multiple Attributes
To replace multiple attributes for active directory users like update department, and company name, run the below command.
Get-ADUser -SearchBase "OU=SALES,DC=SHELLPRO,DC=LOCAL" -filter * -Properties Department,Company | Set-ADUser -Replace @{Department=101;Company="ShellGeek"}
In the above PowerShell script to replace multiple attributes for an ad user, the first command gets aduser from the specified OU and passes the output to the second command.
The second command uses the Set-ADUser
to replace multiple attributes like Department and Company name.
Run script to get aduser for specified OU to check the output of Set-ADUser replace multiple attributes command as below
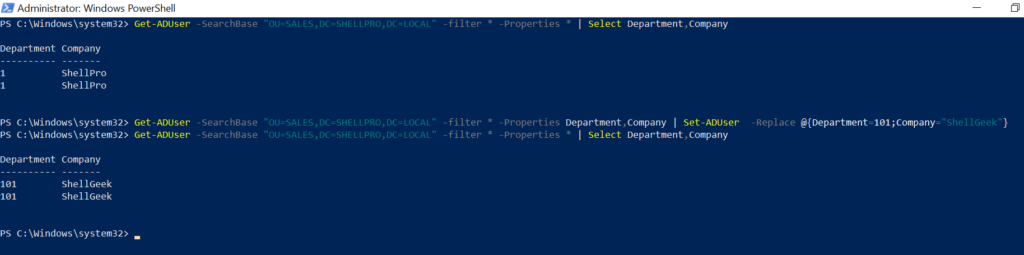
Set-AdUser Clear Attribute Value
Use the Set-AdUser
command with a clear attribute to the clear attribute value of the ad user account.
For example, consider an example to clear department value for a user in the active directory, run the below command
Get-ADUser -filter * -SearchBase "OU=SALES,DC=SHELLPRO,DC=LOCAL" | Set-AdUser -clear department
In the above PowerShell script, the Get-Aduser
cmdlet gets the user from the specified OU and passes the output to the second command.
The second command uses the Set-ADUser
cmdlet to clear attribute value specified by the clear attribute, in this case, department.
The output of the above command after we run the get ad user command is below.
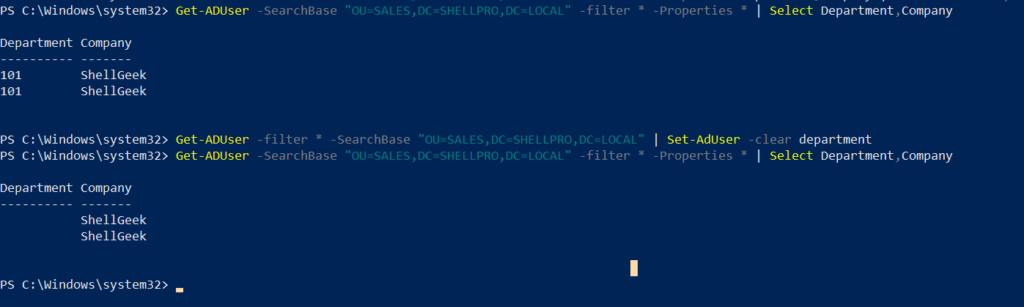
The above image displays the set-aduser clear attribute value of the department for users from specified OU.
Cool Tip: Learn how to query active directory users info!
Set-AdUser Disable Account
As an administrator, we need to keep a check on active user accounts and accounts that are no longer in use. When an employee leaves an organization, we need to disable the active directory user.
Let’s take an example to use a set-aduser disable account scenario for employee Don who leaves an organization.
First, we will use the Get-AdUser cmdlet to get the ad user account status enabled or disabled.
If Get-AdUser
Enabled
attribute has a True value, which means the account is active, False value means the account is disabled.
Get-ADUser -Identity Don -Properties Enabled | Select Enabled
The output of the above command about aduser enabled status is below.
PS C:\Windows\system32> Get-ADUser -Identity Don -Properties Enabled | Select Enabled
Enabled
-------
True
Now, after the user leaves and organization, we need to update his active directory status to disabled. Using the Set-AdUser cmdlet we will disable the account as below.
Get-ADUser -Identity Don -Properties Enabled | Set-ADUser -Enabled $False
In the above PowerShell script, the first command gets aduser enabled status for the user object and passes output to the second command.
The second command uses Set-ADUser to disable the user account using Enable $False
.
The output of the above PowerShell Set-ADUser Disabled account command, after we check the user status as below.
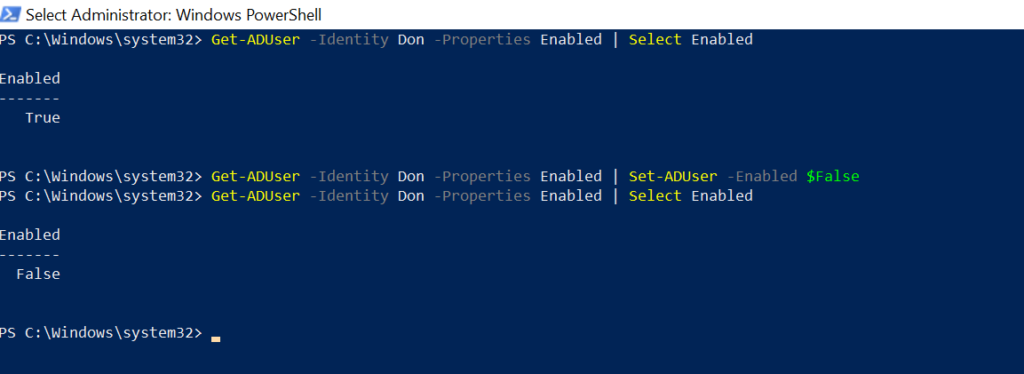
Do you know: How to use the cat command in Windows using PowerShell !
Powershell Set-AdUser FAQ
Set-AdUser cmdlet modifies active directory user attributes. It allows us to modify commonly used user properties using cmdlet parameters.
Using PowerShell Set-Aduser -add,-replace, and -remove parameters, you can set custom attributes in the active directory.
Using PowerShell Set-AdUser properties -MobilePhone, you can easily modify your mobile phone number. Run below command
Set-ADUser -Identity Tom.Smith -MobilePhone 01777896453
Above command, Set-Aduser will modify Tom.Smith user mobile phone.
you can also use the Set-AdUser replace property to modify the phone number as belowSet-ADUser Tom.Smith -replace @{'MobilePhone' = 01777896453 }
Conclusion
In this article, we go through one of the powerful PowerShell Set-ADUser to update active directory user attributes with different examples.
Get-ADUser to get one or more specific user objects and use objects for updating multiple attributes using the PowerShell Set-AdUser cmdlet.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.