Use the Split operator in PowerShell with a regular expression (.{3}) to split the string into fixed lengths in PowerShell. The Split operator breaks the string into multiple substrings based on a delimiter.
PowerShell string contains the sequence of characters. Use the split operator or split function to break the string into multiple substrings.
In this article, we will discuss how to use the Split operator with regex in PowerShell to split a string into fixed lengths.
Use Split Operator in PowerShell to Split String into Fix Length
The Split operator in PowerShell uses the regular expression in delimiter to split the string into fixed lengths. Example, $str -split '(.{3})'|?{$_}
split the string into fix length of 3 characters.
Let’s consider, that the $str
contains the string “ShellGeek PowerShell
“. Use the regular expression in the split operator as given below to break the string into fixed length characters.
$str = "ShellGeek PowerShell" # Break the string into fix length of 3 characters $s1 = $str -split '(.{3})' | ?{$_} $s1
In the above PowerShell script, the split operator uses the regular expression (.{3})
to split the string into the fixed length of 3 characters.
In the regular expression (.{3}),
. matches any character in the string except for the line terminator
{3} matches the previous token exactly 3 times in the string.
The output of the above PowerShell script breaks the string to the fixed lengths of 3 characters.
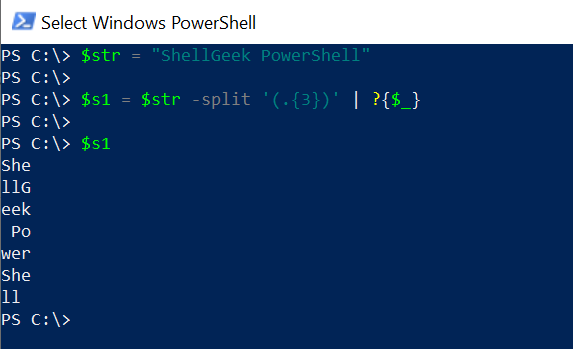
Let’s understand, another way to split a string into fixed lengths with regular expressions in PowerShell.
$str = "ShellGeek PowerShell" # Use the regular expression in Split operator to split the string $s2 = $str -split '(\w{3})' | ?{$_} $s2
In the above PowerShell script, the $str
contains the string data “ShellGeek PowerShell
“.
Use the split operator with a regular expression (\w{3})
to split the string into fixed lengths of characters.
The output of the above PowerShell script is:
PS C:\> $str = "ShellGeek PowerShell"
PS C:\> $s2 = $str -split '(\w{3})' | ?{$_}
PS C:\> $s2
She
llG
eek
Pow
erS
hel
l
PS C:\>
Conclusion
I hope the above article helped you to understand how to split the string into fixed lengths in PowerShell using the Split operator.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.
Recommended Content
PowerShell Substring – How to extract the substring in PowerShell
PowerShell Substring IndexOf – Find the position of the substring.
PowerShell Substring After Character – Extracts the Substring after a character.
PowerShell String Replace – How to replace a string in PowerShell.
PowerShell Split String by Multiple Characters