Using the Indexof() or LastIndexOf() methods in PowerShell, we can find the index position of the substring in PowerShell.
IndexOf() method finds the first occurrence of the specified string in the PowerShell string.IndexOf method counts the index from zero.
LastIndexOf() method finds the last occurrence of the specified string in the PowerShell String.
In this example, we will discuss how to find PowerShell substring Index using the IndexOf and LastIndexOf methods.
Find Substring Index using the IndexOf method in PowerShell
Use the IndexOf method in PowerShell to find the index of a substring in the given PowerShell string. It counts the index from 0.
In the following example, the variable $str stores the string data.
To find the substring index position, it uses IndexOf() method and passes the substring to it.
$str.IndexOf("string")
$str = "PowerShell string IndexOf method finds first occurrence of substring in string" #Print the string $str # Find Substring Index Position $str.IndexOf("string")
In the above PowerShell script, the IndexOf method finds the first occurrence of the substring string and gets the substring index position of the specified substring as 11 which is starting position of a string.
The output of the above script is:
PS C:\> $str = "PowerShell string IndexOf method finds first occurrence of substring in string"
PS C:\> $str
PowerShell string IndexOf method finds first occurrence of substring in string
PS C:\> $str.IndexOf("string")
11
IndexOf method has overload methods where you can specify the start index so that the string or character before the start index is ignored. It finds the first occurrence of a string after the start index.
Let’s consider we have a string as given below
PowerShell string IndexOf method finds first occurrence of substring in string
To find the substring string after index 15, use the following code.
$str = "PowerShell string IndexOf method finds first occurrence of substring in string" $str.IndexOf("string",15)
In the above PowerShell script, the IndexOf method uses the startIndex 15 to ignore any occurrence of string before the start index and start searching for the substring after index position 15.
It returns the position of the substring in a given string as given below.
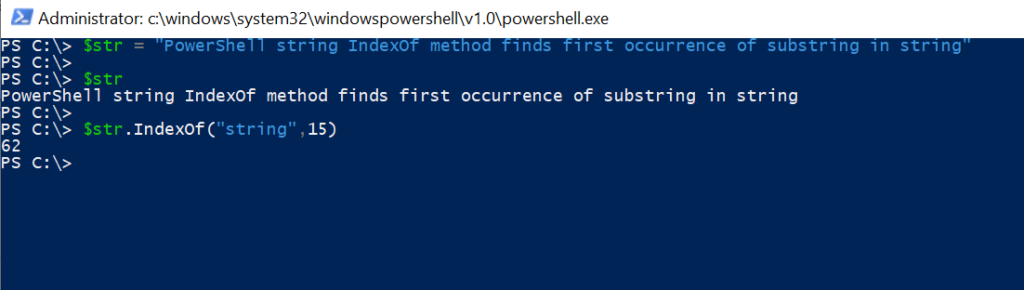
Find Position of Substring using LastIndexOf in PowerShell
Use the LastIndexOf method to find the substring index position in the specified PowerShell string. It finds the last occurrence of a string in the given string.
In the following example, the $str variables contain the string data.
To get the position of the last occurrence of a substring multiple, refer to the below code.
$str = "String Split() breaks the string into multiple parts" $str.LastIndexOf("multiple")
In the above PowerShell script, the LastIndexOf method takes the input parameter to find the last occurrence of a substring in the given string.
The output of the above script is:
PS C:\> $str = "String Split() breaks the string into multiple parts"
PS C:\>
PS C:\> $str
String Split() breaks the string into multiple parts
PS C:\>
PS C:\> $str.LastIndexOf("multiple")
38
PS C:\>
Conclusion
I hope the above article on how to use IndexOf and LastIndexOf methods to find the position of a substring in the given string is helpful to you.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.