JSON (Javascript Object Notation) is a lightweight data-interchange format used to easily store, read and write data. Use the @{}
notation to create JSON variables in PowerShell as objects and arrays.
In PowerShell, the ConvertTo-Json cmdlet converts ps objects to JSON, and the ConvertFrom-Json cmdlet parses JSON data into variables.
In this article, we will discuss how to work with JSON variables in PowerShell, including creating JSON objects, parsing JSON data into variables, and converting PowerShell objects to JSON, manipulating JSON data in PowerShell.
Creating JSON Variables in PowerShell
You can create JSON variables as objects and arrays in PowerShell.
Create JSON Objects
Use the @{}
notation to create a JSON string in PowerShell. Initialize string with property-value pairs.
$jsonString = @" { "Name": "Tom", "Age": 27, "Address": { "Country": "India" } } "@ $jsonObject = ConvertFrom-Json $jsonString # Print the JSON object Write-Host $jsonObject # Print the properties in the JSON object Write-Host $jsonObject.Name
In the above PowerShell script, the JSON string is defined using the here-string syntax (@β and β@) that allows you to create a multi-line string. The variable $jsonString holds a JSON string containing an object with properties and nested objects.
We have used the ConvertFrom-Json cmdlet to convert the $jsonString variable to a PowerShell object and store the converted object in the $jsonObject variable.
$jsonObject
variable is used to access the properties and values in the JSON.
The output of the above PowerShell script after creating the JSON variable and JSON object is to print the value of the βNameβ property in the JSON object to the console.
@{Name=Tom; Age=27; Address=}
Tom
Create JSON Arrays
Use the @{}
notation to create JSON arrays in PowerShell. Initialize string with multi-line string.
$jsonString = @" [ { "Name": "Tom", "Age": 30 }, { "Name": "Harry", "Age": 25 } ] "@ $jsonArray = ConvertFrom-Json $jsonString Write-Host $jsonArray[0].Name
In the above PowerShell script, the here-string ( @β β@) is used to define the JSON string. The JSON string contains an array with two objects with properties like Name and Age and stores string in variable $jsonString
.
Using the ConvertFrom-Json cmdlet, it converted $jsonString
to a PowerShell object. The $jsonArray
variable holds the converted array which can be used to access the objects and properties in the JSON.
The output of the above script when run, print the value of the Name
property in the first object in the JSON array.
Tom
Converting PowerShell Objects to JSON
ConvertTo-Json cmdlet in PowerShell is used to convert objects to JSON:
$process = Get-Process | Select-Object -First 1 $json = $process | ConvertTo-Json
In the above PowerShell script, the Get-Process cmdlet retrieves the list of all running processes on the OS and pipes them to Select-Object to select the first process and store process information in the $process
variable.
The $process
variable that holds the process information pipes the information to the ConvertTo-Json cmdlet which converts the objects to JSON string and stores the result in the $json
variable.
The output of the above PowerShell script converts PowerShell objects to JSON strings.
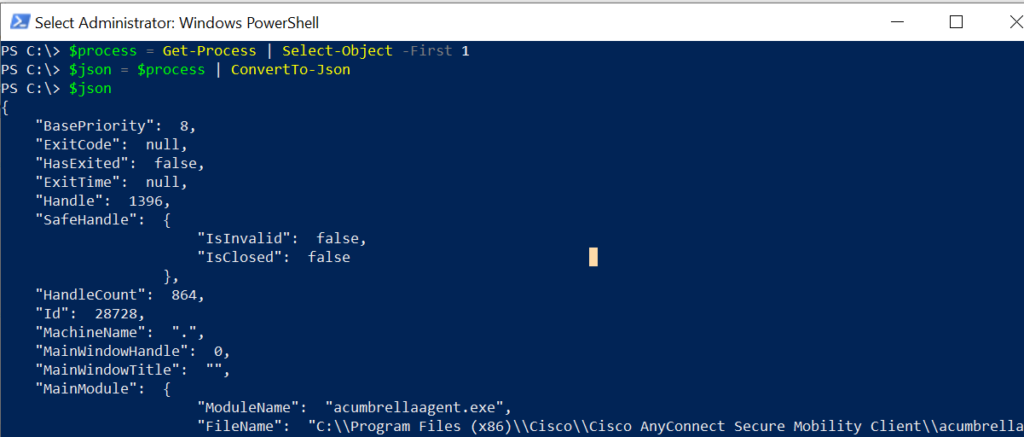
Parsing JSON Object to PowerShell Variables
Use the ConvertFrom-Json cmdlet to parse data into PowerShell variables.
$jsonString = @" { "Name": "Tom", "Age": 27, "Address": { "Country": "India" } } "@ $jsonObject = ConvertFrom-Json $jsonString
In the above PowerShell script, the $jsonString
variable holds the JSON data that contains objects and properties. Using the ConvertFrom-JSON cmdlet, it parsed the JSON data into variable $jsonObject
.
The $jsonObject
can be used to easily access the objects and properties in the JSON data.
Manipulating JSON Data
We can easily manipulate JSON data in PowerShell such as adding, modifying, or removing properties in the JSON object.
Letβs consider the below JSON string and the object that holds the JSON data.
$jsonString = @" { "Name": "Tom", "Age": 30, "Address": { "Street": "Ashoka", "City": "Delhi", "State": "India" } } "@ $jsonObject = ConvertFrom-Json $jsonString
Add New Properties or Key to JSON Objects
Use the Add-Member cmdlet to add a new property or key to the JSON object.
# Add a new property to the JSON object $jsonObject | Add-Member -MemberType NoteProperty -Name "Email" -Value "[email protected]"
In the above PowerShell script, the $jsonObject
variable holds the JSON data. It has been sent to the Add-Member cmdlet to Add a new property named βEmailβ with its value β[email protected]β.
The output of the above PowerShell script adds the key-value pair for the new property βEmailβ to the existing JSON data.
PS C:\> $jsonObject | Add-Member -MemberType NoteProperty -Name "Email" -Value "[email protected]"
PS C:\> $jsonObject
Name Age Address Email
---- --- ------- -----
Tom 30 @{Street=Ashoka; City=Delhi; State=India} [email protected]
Modify Property Value in JSON Object
In PowerShell, we can easily modify the property value of any object in JSON data by accessing it and assigning value to it.
# Modify an existing property value in the JSON object $jsonObject.Age = 32
In the above PowerShell script, the $jsonObject
variable stores the JSON data. It accesses the Age
property of the JSON object and assigns a new value to it. It modifies the existing JSON object.
The output of the above script modifies the existing property value.
PS C:\> $jsonObject.Age = 32
PS C:\> $jsonObject
Name Age Address Email
---- --- ------- -----
Tom 32 @{Street=Ashoka; City=Delhi; State=India} [email protected]
Remove an Existing Property from JSON Object
Use the Remove()
method of the PSObject.Properties
collection to remove the Address
property from the JSON object.
# Remove an existing property from the JSON object $jsonObject.PSObject.Properties.Remove("Address")
The output of the above PowerShell script removes the property βAddressβ from the JSON data.
PS C:\> $jsonObject.PSObject.Properties.Remove("Address")
PS C:\> $jsonObject
Name Age Email
---- --- -----
Tom 32 [email protected]
PS C:\>
Saving JSON Data to a File
Use the Set-Content cmdlet in PowerShell to save JSON data to a file.
Set-Content -Path 'shellusers.json' -value $jsonString
In the above PowerShell script, the $jsonString
variable holds the JSON data. The Set-Content cmdlet uses the -Path
parameter to write the JSON string to a file named shellusers.json
Reading JSON Data from a File
Use the Get-Content cmdlet in PowerShell to read JSON data from a file.
# Read the JSON data from a file $jsonString = Get-Content -Path "D:\PS\shellusers.json" # Read JSON data from a file and convert JSON data to PowerShell object $jsonObject = Get-Content -Path "D:\PS\shellusers.json" | ConvertFrom-JSON
In the above PowerShell script, the Get-Content
cmdlet reads the JSON data from a file and stores the JSON string in the variable $jsonString
.
In the next command, it uses the ConvertFrom-JSON cmdlet to convert JSON data to a PowerShell object and store it in the variable $jsonObject
.
Conclusion
I hope the above article on how to work with JSON data in PowerShell and use the JSON variables is helpful to you.
Working with JSON data in PowerShell allows you to efficiently manage structured data. You can create, modify, remove, and store JSOn variables.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.