The list of users from the organization unit can be retrieved using Get-AdUser with the SearchBase parameter to search for users in specific OU and Get-AdOrganizationalUnit in PowerShell
The OrganizationalUnit in the Active Directory contains the users, groups, computers, and AD objects. Get-AdUser cmdlet in PowerShell helps to get one or more ad users from the active directory.
In this article, we will discuss how to list users from OU in the Active Directory.
Get a list of users from OU
Using the Get-AdUser in PowerShell, you can easily find the users from the specific OU.
Let’s practice with an example to get a list of users from the ad organizational unit.
$OU = 'OU=SALES,DC=SHELLPRO,DC=LOCAL' # Use Get-AdUser to search within organizational unit to get users name Get-ADUser -Filter * -SearchBase $OU | Select-object DistinguishedName,Name,UserPrincipalName
In the above PowerShell script to find the users from OU,
$OU variable in PowerShell contains the OU name.
Using Get-ADUser in PowerShell, it uses Filter and SearchBase parameters to search for users within the specified OU.
It selects the ad user distinguished name, name, and its userprincipalname.
The output of the above PowerShell script to get all users in OU is:
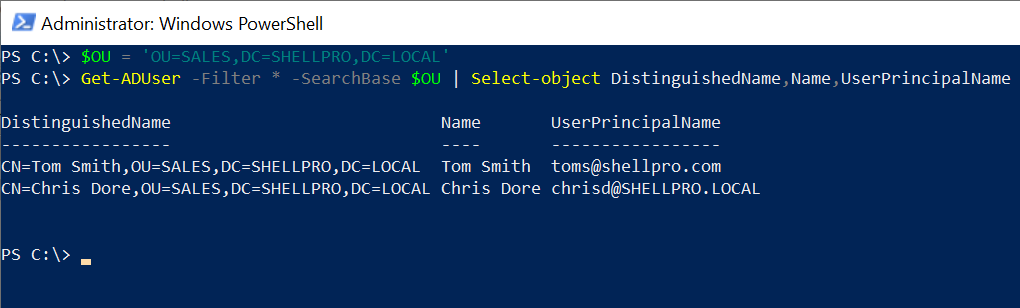
Cool Tip: How to find computers in OU in the Active Directory!
Get all users in ou and sub ou
Using the Get-AdUser cmdlet in PowerShell, you can get all users in ou and sub ou.
It uses the SearchBase parameter to search within the given ou and using the SearchScope subtree parameter, it gets all the sub ou users.
Let’s practice with the example.
$OU = 'OU=SHELLUSERS,DC=SHELLPRO,DC=LOCAL' Get-ADUser -Filter * -SearchBase $OU -SearchScope Subtree | Select-object DistinguishedName,Name,UserPrincipalName
The output of the above PowerShell script to get all users in ou and sub ou is:
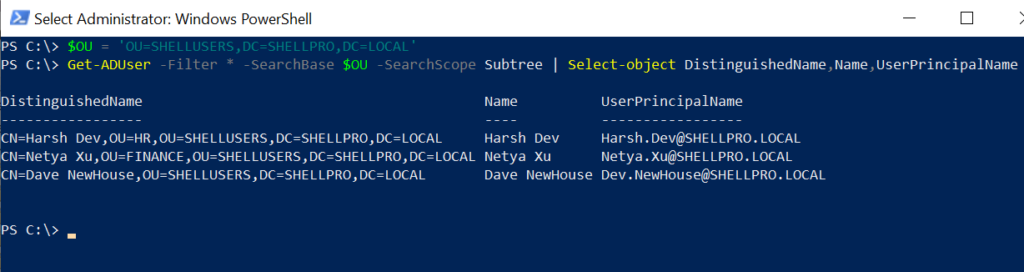
Get AdUsers list from Multiple Organizational Units
Using the Get-AdUser SearchBase parameter, you can get adusers list from multiple ou’s.
# Specify multiple OU $OU = 'OU=SALES,DC=SHELLPRO,DC=LOCAL','OU=SHELLUSERS,DC=SHELLPRO,DC=LOCAL' # Use foreach to iterate over multiple ou to get aduser $OU | ForEach-Object {Get-AdUser -filter {enabled -eq $true} -searchbase $_ -properties canonicalname,userprincipalname } | Select SamAccountName,canonicalname,userprincipalname
In the above command, the $OU variable contains multiple OU’s.
Use the ForEach -Object to iterate over ou and use the Get-AdUser SearchBase parameter to get users from multiple ou’s.
The output of the above command is:
PS C:\> $OU = 'OU=SALES,DC=SHELLPRO,DC=LOCAL','OU=SHELLUSERS,DC=SHELLPRO,DC=LOCAL'
PS C:\> $OU | ForEach-Object {Get-AdUser -filter {enabled -eq $true} -searchbase $_ -properties canonicalname,userprincipalname } | Select SamAccountName,canonicalname,userprincipalname
SamAccountName canonicalname userprincipalname
-------------- ------------- -----------------
toms SHELLPRO.LOCAL/SALES/Tom Smith [email protected]
chrisd SHELLPRO.LOCAL/SALES/Chris Dore [email protected]
Harsh.Dev SHELLPRO.LOCAL/SHELLUSERS/HR/Harsh Dev [email protected]
Netya.Xu SHELLPRO.LOCAL/SHELLUSERS/FINANCE/Netya Xu [email protected]
Dev.NewHouse SHELLPRO.LOCAL/SHELLUSERS/Dave NewHouse [email protected]
Export list of users in ou to csv
In the above example, we have seen you can get a list of users from OU in the Active Directory.
Using the Export-CSV in PowerShell, you can export a list of ad users in OU to a CSV file at the specified location.
Let’s practice!
# Get the OU and sub OU list from the active directory by OU name $OUList = (Get-ADOrganizationalUnit -Filter 'Name -like "SALES"' -SearchScope Subtree) | select DistinguishedName $OUList | foreach { $ADUsersList = Get-ADUser -Filter * -SearchBase $_.DistinguishedName | Select-Object Name,DistinguishedName,UserPrincipalName } $ADUsersList | Export-CSV C:\PowerShell\OU_adusers.csv -NoTypeInformation
In the above PowerShell script to export a list of users from ou and sub ou,
- $OUList variable contains the OU and sub ou name retrived by Get-AdOrganizationalUnit in PowerShell. It contains distinguishedname of OU.
- Used the foreach loop to iterate over ou list
- Used Get-AdUser in PowerShell to search for user in specific OU using the SearchBase parameter.
- It returns ad user name, distinguishedname and userprincipalname of users from ou.
- Used Export-Csv in PowerShell to export list of users in OU to csv file.
The output of the above PowerShell script in CSV file is:
"Name","DistinguishedName","UserPrincipalName"
"Tom Smith","CN=Tom Smith,OU=SALES,DC=SHELLPRO,DC=LOCAL","[email protected]"
"Chris Dore","CN=Chris Dore,OU=SALES,DC=SHELLPRO,DC=LOCAL","[email protected]"
Conclusion
I hope you may find an article on how to get a list of users from OU in the Active Directory using the Get-AdUser and Get-AdOrganizationalUnit cmdlet in PowerShell.
Get-AdUser in PowerShell gets one or more users from the search condition. In the above script, we have specified OU distinguished name in the SearchBase parameter.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.