Very often, PowerShell administrators have to scan servers manually to see the performance issue with a server in the network. It might be possible that one of the servers was not responding or unresponsive and caused performance issues on dependent servers. In this kind of scenario, having information memory usage for servers will help to identify which specific server has higher memory utilization.
Use PowerShell Get-WMIObject
command to get memory usage on the computer. There are multiple ways to get memory utilization on the local computer or remote computer in PowerShell.
In this article, I will explain how to get memory usage for servers using PowerShell.
PowerShell Get Memory Usage
To get memory usage for the top 10 processes on a local computer using PowerShell, use the below command
PS D:\PowerShell> Get-WmiObject WIN32_PROCESS | Sort-Object -Property ws -Descending | Select-Object -first 10 ProcessID,Name,WS ProcessID Name WS --------- ---- -- 2628 Memory Compression 909996032 11984 chrome.exe 470863872 15728 firefox.exe 356192256 14444 chrome.exe 332091392 16680 firefox.exe 315645952 11812 chrome.exe 311554048 22072 Teams.exe 242507776 11256 firefox.exe 221163520 27052 powershell_ise.exe 213434368 25612 chrome.exe 211025920
In the above PowerShell script,
- using Get-WMIObject cmdlet and WIN32_Process class it gets information about all processes on the local computer.
- Sort all processes descending by WS
- Using Select-Object to get the first 10 process id, process names, and WS
- It gets memory usage of the top 10 processes
The output of the above PowerShell script retrieves the process memory usage.
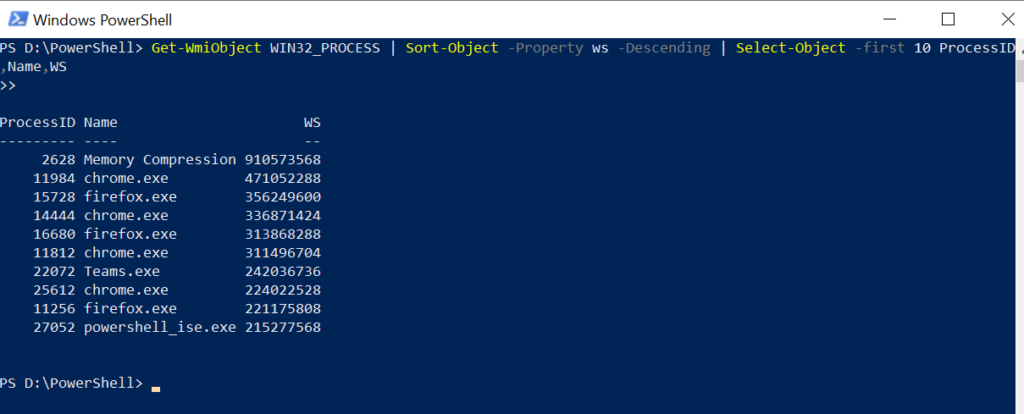
As you can see from the above information we get an idea about which processes are having higher memory usage but it’s very difficult to know how much memory usage in percentage these processes will be utilizing.
Cool Tip: How to create a resource pool for measuring memory usage of VM!
To get total memory usage in percentage on the computer, we need to know how much TotalVisibleMemorySize and FreePhysicalMemory are available on the system.
Run the below command to get process memory usage in MB on the local computer
# Get Computer Object $CompObject = Get-WmiObject -Class WIN32_OperatingSystem $Memory = ((($CompObject.TotalVisibleMemorySize - $CompObject.FreePhysicalMemory)*100)/ $CompObject.TotalVisibleMemorySize) Write-Host "Memory usage in Percentage:" $Memory # Top 5 process Memory Usage (MB) $processMemoryUsage = Get-WmiObject WIN32_PROCESS | Sort-Object -Property ws -Descending | Select-Object -first 5 processname, @{Name="Mem Usage(MB)";Expression={[math]::round($_.ws / 1mb)}} $processMemoryUsage
Output:
Memory usage in Percentage: 78.134547088066
ProcessName Mem Usage(MB)
----------- -------------
Memory Compression 1032
chrome.exe 373
Teams.exe 370
firefox.exe 315
chrome.exe 307
In the above PowerShell script,
- Using Get-WmiObject -Class Win32_OperatingSystem get the local computer object
- Get information about TotalVisibleMemorySize and FreePhysicalMemory
- Calculate and get memory usage in percentage
- Using Win_32_Process gets the process, sorted by ws descending
- Using Select-Object to get top 5 process memory usage
- Easy to read and interpret which processes have higher memory usage.
Cool Tip: How to use multiline command in PowerShell!
PowerShell Get Memory Usage on Remote Computer
To get memory usage for the top 10 processes on a remote computer using PowerShell, use the below command
PS D:\PowerShell> Get-WmiObject WIN32_PROCESS -ComputerName 'corp-in-18' | Sort-Object -Property ws -Descending | Select-Object -first 5 ProcessID,Name,WS >> ProcessID Name WS --------- ---- -- 12628 Memory Compression 913199104 10522 chrome.exe 438820864 17292 Outlook.exe 393461760 26529 firefox.exe 354979840 23133 chrome.exe 325996544
In the above PowerShell script,
- using Get-WMIObject cmdlet and WIN32_Process class it gets information about all processes on remote compute name specified by –
ComputerName
property - Sort all processes descending by WS
- Using
Select-Object
to get the first 5process id, process name,s and WS - It gets memory usage of the top 5 processes
To get total memory usage in percentage on a remote computer, get TotalVisibleMemorySize and FreePhysicalMemory available on the system.
Run the below command to get process memory usage in MB on the remote computer.
$CompName = 'corp-in-18' # Get Computer Object $CompObject = Get-WmiObject -Class WIN32_OperatingSystem -ComputerName $CompName $Memory = ((($CompObject.TotalVisibleMemorySize - $CompObject.FreePhysicalMemory)*100)/ $CompObject.TotalVisibleMemorySize) Write-Host "Memory usage in Percentage:" $Memory # Top 5 process Memory Usage (MB) $processMemoryUsage = Get-WmiObject WIN32_PROCESS -ComputerName $CompName | Sort-Object -Property ws -Descending | Select-Object -first 5 processname, @{Name="Mem Usage(MB)";Expression={[math]::round($_.ws / 1mb)}} $processMemoryUsage
Output
Memory usage in Percentage: 77.2521027641826
ProcessName Mem Usage(MB)
----------- -------------
Memory Compression 1105
chrome.exe 358
firefox.exe 318
chrome.exe 302
firefox.exe 290
In the above PowerShell script,
- Using the Get-WmiObject cmdlet gets a remote computer object specified by
ComputerName
- Get information about TotalVisibleMemorySize and FreePhysicalMemory
- Calculate and get memory usage in percentage
- Using Win_32_Process gets the all processes on the remote computer, sorted by ws descending
- Using Select-Object to get top 5 process memory usage
Cool Tip: How to add a new line to a string or variable in PowerShell!
PowerShell Get RAM Usage
To get RAM size usage in Windows using PowerShell, you can use the Get-Counter command to retrieve the performance counter data related to memory usage.
Get-Counter -Counter "\Memory\Committed Bytes" | Select-Object -ExpandProperty CounterSamples | ForEach-Object { "{0:N2} GB" -f ($_.CookedValue / 1GB) }
In the above PowerShell script, the Get-Counter command retrieves the amount of committed memory in bytes and later converts it to gigabytes (GB).
The above command provides the amount of committed memory, which is a portion of the RAM that is actively being used by running processes.
Conclusion
I hope the above article to get memory usage on the local computer or remote computer using PowerShell script will help you to get memory usage information and take action on it.
Using Win32_Process
class, it returns all processes available on the computer specified by ComputerName.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.