PowerShell has a built-in cmdlet New-Variable to create read-only and constant variables. This command uses the parameter -Option Constant to create a constant variable.
The difference between the read-only and constant variables in Powershell is, read-only variables are those that can be assigned a value only once and their value canβt be modified afterward. Constant variables are similar to read-only variables but they can not be removed or changed, even by using the Remove-Variable cmdlet.
In this article, we will discuss how to create read-only and constant variables in PowerShell.
Create Read-Only Variables in PowerShell
Use the Set-Variable cmdlet in PowerShell with the parameter -Option Readonly
to create a read-only variable.
# Create read-only variable in PowerShell using Set-Variable Set-Variable -Name piValue -Value "3.14" -Option ReadOnly # Print the variable value $piValue
In the above PowerShell script, the Set-Variable cmdlet uses the -Name
parameter to specify the variable `piValue
` and `-Value
` parameter to assign the value to a variable and finally `-Option
` parameter to set to ReadOnly.
The output of the above script creates a read-only variable as given below:
PS D:\> Set-Variable -Name piValue -Value "3.14" -Option ReadOnly
PS D:\> $piValue
3.14
If you try to change or modify the read-only variable value to another value, it will throw an exception.
$piValue = 3.145
By assigning value to read-only variable throw an exception as given below
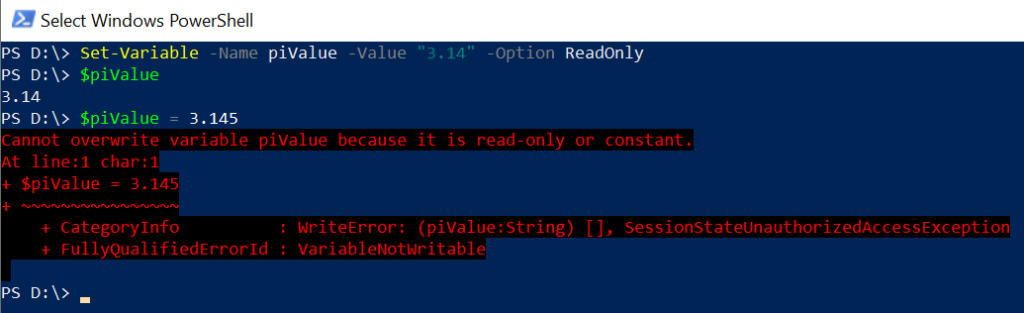
Create Constant Variables in PowerShell
To create a constant variable, use the Set-Variable cmdlet with `-Option
` parameter set to `Constant
`.
# Create PowerShell constant variable Set-Variable -Name dbServerIPAddress -Value "10.1.1.1" -Option Constant # Print the PowerShell constant variable value $dbServerIPAddress
In the above PowerShell script, the Set-Variable cmdlet uses the Name
parameter to specify the variable `dbServerIPAddress
` and `-Value
` parameter to assign the constant value to a variable and finally `-Option
` parameter to set to Constant.
The output of the above script creates PowerShell constant variable $dbServerIPAddress with a value assigned to it.
PS D:\> Set-Variable -Name dbServerIPAddress -Value "10.1.1.1" -Option Constant PS D:\>
PS D:\> $dbServerIPAddress 10.1.1.1
PS D:\>
If you try to modify the PowerShell constant variable value, it will throw an exception.
$dbServerIPAddress = 10.1.1.2
Exception as given below:
PS D:\> Set-Variable -Name dbServerIPAddress -Value "10.1.1.1" -Option Constant PS D:\>
PS D:\> $dbServerIPAddress 10.1.1.1
PS D:\> $dbServerIPAddress = 10.1.1.2 Cannot overwrite variable dbServerIPAddress because it is read-only or constant.
At line:1 char:1
+ $dbServerIPAddress = 10.1.1.2
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : WriteError: (dbServerIPAddress:String) [], SessionStateUnauthorizedAccessException
+ FullyQualifiedErrorId : VariableNotWritable
PS D:\>
Comparing Read-Only and Constant Variables
In PowerShell, both read-only and constant variables can not have their value modified, the main key difference between them is the removal of variables.
The read-only variables can be removed using the Remove-Variable cmdlet, whereas the constant variables cannot be removed.
# Remove read-only variable Remove-Variable -Name "piValue" -Force # Remove PowerShell constant variable Remove-Variable -Name "dbServerIPAddress" -Force
In the above PowerShell script, the Remove-Variable cmdlet uses the -Name
parameter to specify the read-only variable name and -Force
parameter to forcefully delete the parameter. It removes the read-only variable.
While trying to remove the PowerShell constant variable, it throws an exception as given below:
PS D:\> Remove-Variable -Name "piValue" -Force
PS D:\> Remove-Variable -Name "dbServerIPAddress" -Force Remove-Variable : Cannot remove variable dbServerIPAddress because it is constant or read-only. If the variable is
read-only, try the operation again specifying the Force option.
At line:1 char:1
+ Remove-Variable -Name "dbServerIPAddress" -Force
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : WriteError: (dbServerIPAddress:String) [Remove-Variable], SessionStateUnauthorizedAccess
Exception
+ FullyQualifiedErrorId : VariableNotRemovable,Microsoft.PowerShell.Commands.RemoveVariableCommand
PS D:\>
Cool Tip: How to clear variables value in PowerShell!
Conclusion
I hope the above article on how to create PowerShell constants and read-only variables using the Set-Variable cmdlet is helpful to you.
While creating the read-only or constants variables in PowerShell, use the Set-Variable cmdlet with -Option
parameter value set to βReadOnlyβ or βConstantβ respectively.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.