The Get-MgUser cmdlet in PowerShell is used to retrieve information about Microsoft Graph Users. This command allows you to get and extract information about users, or specific users based on criteria such as user name, email address, and manager from Azure Active Directory.
User accounts within your Microsoft 365 tenant are centrally stored in Azure Active Directory
The syntax to retrieve the users in the Azure Active Directory is given below.
Get-MgUser
-UserId <String>
-InputObject <IUsersIdentity>
[-ExpandProperty <String[]>]
[-Property <String[]>]
[-Filter <String>]
[-Search <String>]
[-Sort <String[]>]
[-Top <Int32>]
[-ConsistencyLevel <String>]
[-PageSize <Int32>]
[-All]
[-CountVariable <String>]
[<CommonParameters>]
In this article, we will discuss how to use the Get-MgUser cmdlet in PowerShell to get the list of all the users, get a user by ID, and get the user based on filter criteria.
How to Get the List of All the Users in Azure AD
Firstly, you need to connect to the Microsoft Graph with the correct scope. To retrieve the user data, we can use the User.Read.All
scope.
Connect-MgGraph -Scopes 'User.Read.All'
This command provides the consent to permissions to read the user data.
To get the list of all the users in Microsoft Graph, use the Get-MgUser cmdlet in PowerShell.
Get-MgUser -All
This command returns a list of all users in Azure Active Directory.
The output of the Get-MgUser cmdlet includes the following properties.
- DisplayName: The name of the user.
- Id: The unique ID of the user.
- Mail: The email address of the user.
- UserPrincipalName: The user principal name of the user.
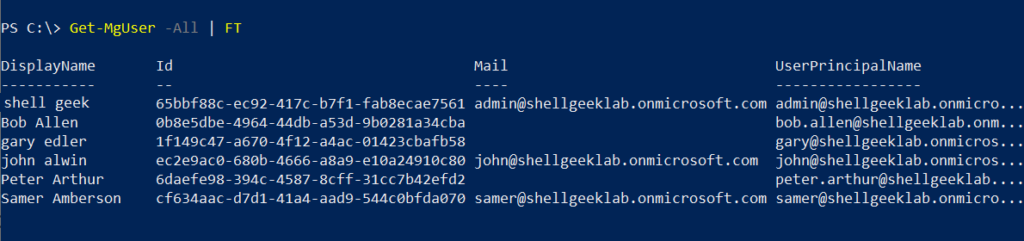
How to Get User by Id and UserPrincipalName
Use the Get-MgUser cmdlet in PowerShell with the -UserId
parameter to get a user by its user principal name. The -UserId
parameter specifies the unique identifier of a user.
# Get the user by the UserPricipalName Get-MgUser -UserId samer@shellgeeklab.onmicrosoft.com
This command gets a user by UserPrincipalName.
You can get the user by user id, by using the following command.
Get-MgUser -UserId cf634aac-d7d1-41a4-aad9-544c0bfda070
The output of the above command provides the user properties such as DisplayName, Id, Mail, UserPrincipalName.
PS C:\> Get-MgUser -UserId samer@shellgeeklab.onmicrosoft.com
DisplayName Id Mail UserPrincipalName
----------- -- ---- -----------------
Samer Amberson cf634aac-d7d1-41a4-aad9-544c0bfda070 samer@shellgeeklab.onmicrosoft.com samer@shellgeeklab.onmicrosof...
PS C:\> Get-MgUser -UserId cf634aac-d7d1-41a4-aad9-544c0bfda070
DisplayName Id Mail UserPrincipalName
----------- -- ---- -----------------
Samer Amberson cf634aac-d7d1-41a4-aad9-544c0bfda070 samer@shellgeeklab.onmicrosoft.com samer@shellgeeklab.onmicrosof...
PS C:\>
The Get-MgUser cmdlet can be used to retrieve a variety of information about Microsoft Graph Users, such as:
- Display Name,
- Id
- Email Address
- Department
- Job Title
- Phone Number
- Created Date Time
- Office Location
- Country
- Manager
- License Assignments
This information can be used to manage user accounts and track user activity.
How to Get a Count of All Users in Microsoft Graph
To get a count of all users in the Azure AD, use the following command.
Get-MgUser -Count userCount -ConsistencyLevel eventual
This command returns the list of all users in the Azure AD and their count. The $userCount
variable contains the count of the objects in the result.
The Get-MgUser command uses the -Count
parameter and the -ConsistencyLevel parameter that indicates the requested consistency level.
How to Use Get-MgUser All Properties
To get all properties of the Microsoft Graph users, use the Get-MgUser cmdlet. This command retrieves the default properties of the user and pipes the output of the user objects to the Format-List
(FL) command to get all the properties for the user object.
Get-MgUser -UserId '65bbf88c-ec92-417c-b7f1-fab8ecae7561' | FL
This command retrieves all the properties for the Microsoft Graph user in Azure AD.
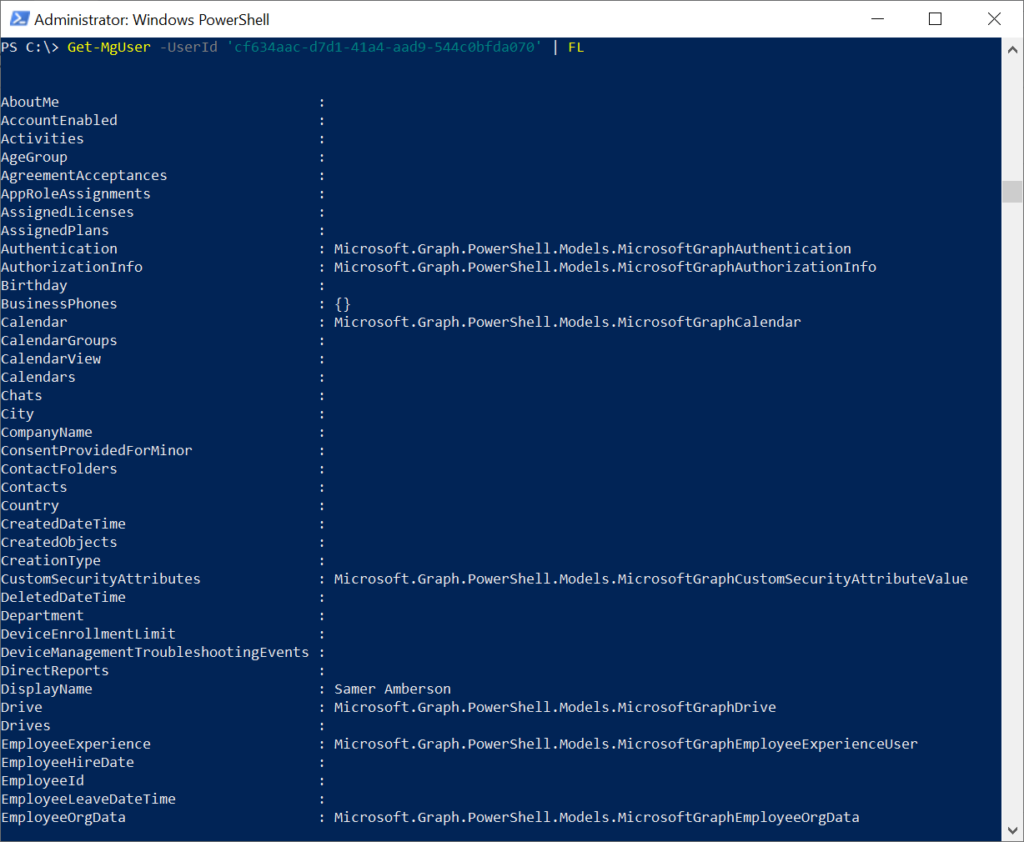
You can select the specific properties of the user by running the following command.
Get-MgUser -UserId 'cf634aac-d7d1-41a4-aad9-544c0bfda070' | Select Id, Email,Mail, JobTitle
This command prints the specific properties of the user.
PS C:\> Get-MgUser -UserId 'cf634aac-d7d1-41a4-aad9-544c0bfda070' | Select Id, Email,Mail, JobTitle
Id Email Mail JobTitle
-- ----- ---- --------
cf634aac-d7d1-41a4-aad9-544c0bfda070 samer@shellgeeklab.onmicrosoft.com Manager
How to Use Filters with Get-MgUser Command
Using the Filter with Get-MgUser cmdlet in PowerShell allows you to retrieve the users based on the filter criteria.
You can use the following operators such as eq, and, or and startswith to filter the users with Get-MgUser cmdlet.
Let’s understand using filters with the Get-MgUser examples.
How to Find the User by the DisplayName
To find the user by the display name, use the following command.
Get-MgUser -Filter "DisplayName eq 'Samer Amberson'"
This command finds the user by their full name.
The output of the Get-MgUser command is given below.
PS C:\> Get-MgUser -Filter "DisplayName eq 'Samer Amberson'"
DisplayName Id Mail UserPrincipalName
----------- -- ---- -----------------
Samer Amberson cf634aac-d7d1-41a4-aad9-544c0bfda070 samer@shellgeeklab.onmicrosoft.com samer@shellgeeklab.onmicrosof...
How to Find Mg Users by Department
To get Microsoft graph users by department name and country, use the following command.
Get-MgUser -Filter "department eq 'Finance' and country eq 'United States'"
This command returns all the users who belong to the “Finance” department in the country “United States“.

How to Get Enabled User Accounts in Azure AD
To get enabled user accounts with the Get-MgUser cmdlet in PowerShell, run the following command.
Get-MgUser -Filter 'accountEnabled eq true' -All
The Get-MgUser command uses the -Filter
parameter to specify the criteria to get user accounts accountEnabled
set to true.
How to Get Users with Display Name that Starts with ‘j’
To get users with a display name that starts with ‘j‘, use the Get-MgUser cmdlet with the -Filter
parameter. The -Filter
parameter is used to specify the criteria to find the user with a display name starting with ‘j’ using the startswith parameter.
Get-MgUser -Filter "startsWith(DisplayName, 'J')"
This command returns the user having displayname starts with ‘J’.
PS C:\> Get-MgUser -Filter "startsWith(DisplayName, 'J')"
DisplayName Id Mail UserPrincipalName
----------- -- ---- -----------------
john alwin ec2e9ac0-680b-4666-a8a9-e10a24910c80 john@shellgeeklab.onmicrosoft.com john@shellgeeklab.onmicrosoft.com
PS C:\>
How to Use Search to Find Users in Azure AD
The Get-MgUser cmdlet has a -Search parameter that can be used to find users in the Azure AD.
Get-MgUser -Search 'DisplayName:joh' -ConsistencyLevel eventual
The Get-MgUser command uses the -Search
parameter to specify the search criteria such as finding the user having ‘joh’ as part of their display name.
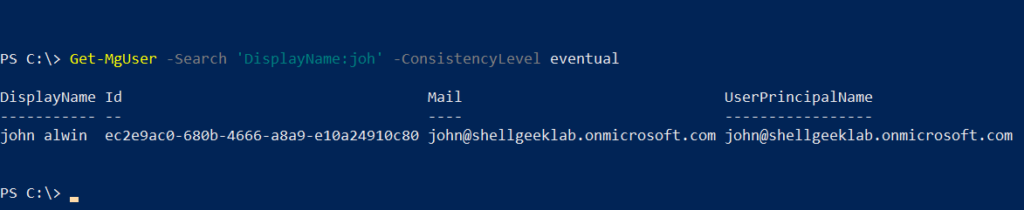
How to Get All Users and Export to CSV
To get all users from the Azure Active Directory, use the Get-MgUser
cmdlet with the -All
parameter. This command retrieves all the uses from the Azure AD and pipes them to the Export-CSV cmdlet to export them to the CSV file.
Get-MgUser -All | Export-Csv -Path D:\PS_delete\mgusers_list.csv -NoTypeInformation
This command gets all users from Azure AD and exports them to the CSV file on the specified location.
Conclusion
I hope the above article on how to use the Get-MgUser cmdlet to get all the users from Azure AD, filter, or search the users with PowerShell is helpful to you.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.