In PowerShell Do Until loop is used when we want to repeatedly execute statements as long as the condition is false.
Like the Do-While loop, Do Until loop always runs at least once before the condition is evaluated in PowerShell.
If the condition specified in Until evaluates to true, Do until loop stop and exist out of the loop.
PowerShell Do Until loop executes script block at least one or more times based on Until condition.
If the condition specified in Until never becomes true, the Do…Until goes into an infinite loop.
In this article, I will explain PowerShell Do Until loop, how to use it with practical examples.
PowerShell Do Until Syntax
Following is the Do…Until loop syntax in PowerShell
Syntax
do { <statement list> } until (<condition>)
In the above syntax, the statement list contains a script block that will be executed each time loop is repeated.
Condition is a statement that resolves to either True or False ( boolean). You can specify do until loop with multiple conditions.
Flow Diagram
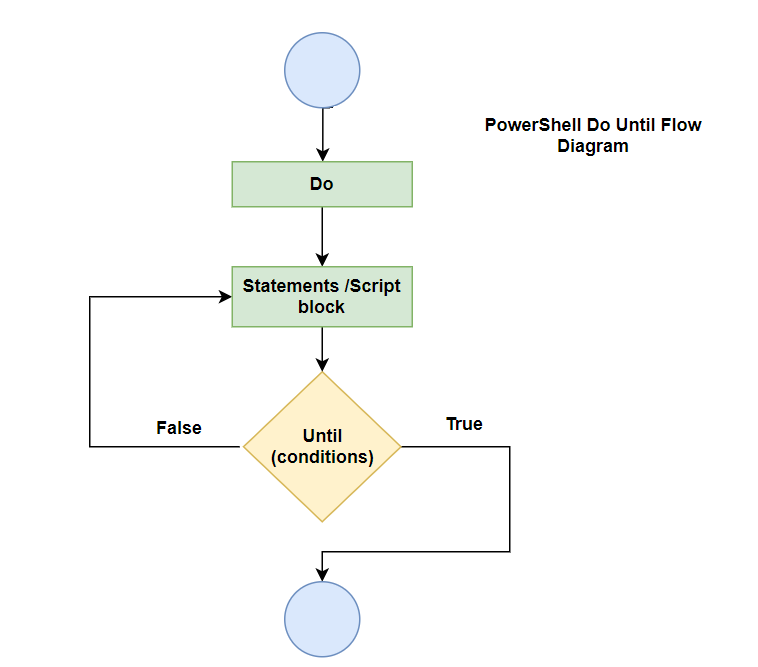
Let’s understand Do…Until loop in PowerShell with examples.
Do Until Loop Counter Example
Let’s consider a simple example to understand Do…Until loop in PowerShell as below
$index = 0 $counter = 0 Do { "Index Value : $index" $counter++ $index++ } Until ($index -eq 10)
In the above PowerShell script,
We have declared two variables as $index and $counter and initialized them to 0.
In Do, we have a statement to display the current index value, the increment loop counter, and the index counter by 1.
Until contains a condition to check if $index
the variable is equal to 0.
As we know, Do…Until loop executes statement at least once and later evaluate Until condition.
In the above case, it will print the Index value till 9 until $index
is equal to 10.
The output of the above script as below
Index Value : 0
Index Value : 1
Index Value : 2
Index Value : 3
Index Value : 4
Index Value : 5
Index Value : 6
Index Value : 7
Index Value : 8
Index Value : 9
When $index = 10, Until condition $index -eq 10 will evaluate to true and stop do until loop.
Note: If Condition specified in Until never becomes true, script block run. It will go to an infinite loop. To stop the Do Until loop, use Ctrl+C on the terminal or use the red square in ISE.
PowerShell Do Until Test-Connection
The practical use of the Do Until loop is to test the connection of the computer if the computer is online or offline.
Let’s understand with an example below
$compName = "incorp-eu-101" Do { Write-Host "$compName is Offline" Start-Sleep 1 } Until (Test-Connection -ComputerName $compName -Quiet -Count 2) Write-Host "$compName is Online"
In the above PowerShell Do Until Example,
$compName
contains the computer name.
In the Do loop, it print computer is Offline using Write-Host and uses Start-Sleep to sleep for 1 sec.
In Until, it uses Test-Connection cmdlet to test the connection to specified computer name quietly using -Quite parameter and retry 2 times using the Count parameter.
Do…Until the loop keeps printing computer is offline until computer connection status resolves to true.
Once the computer connection is successful, the condition evaluates to true.
It breaks do until loop and print computer is Online message on the terminal.
Cool Tip: How to add content to file in PowerShell!
Conclusion
I hope the above article on PowerShell Do Until loop is helpful to you.
There are many practical cases like Do until file exists in the directory, do until end of file, do until end of an array and so many.
Do until loop keeps running until the condition is false and it will stop when the condition evaluates to true.
Do Until at least run one time before the condition is evaluated.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.