PowerShell variable wildcards allow you to write powerful scripts with pattern matching.
Two main types of wildcards *
and ?
are used in PowerShell for pattern matching.
*
– Matches zero or more characters?
– Matches exactly one character
In this tutorial, we will discuss how to use wildcards with PowerShell variables and practical examples to enhance scripts.
Using Wildcards with PowerShell Variables
Using the -Like
operator
To compare a variable with a wildcard pattern, use the Like operator that compares strings using wildcard patterns.
$temp = "ShellGeek" $result = $temp -like "Shell*"
In the above PowerShell script, the $temp
variable stores the string type value. The -like
operator compares string using wildcard patterns * to check if a string matches one or more characters in the given string.
The output of the above PowerShell script is:
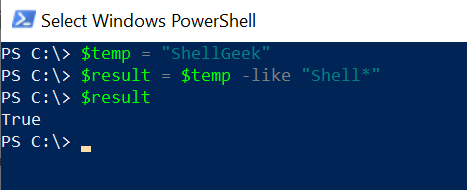
The Where-Object cmdlet with Wildcards
The Where-Object cmdlet is commonly used with wildcards to filter objects based on their properties.
$files = Get-ChildItem -Path 'D:\PS\' $textFiles = $files | Where-Object {$_.name -like "*.txt"} $textFiles
In the above PowerShell script, the Get-ChildItem cmdlet retrieves the items from the specified path and stores them in the variable $files.
The Where-Object
cmdlet is used on the $files
variable to filter the files having their file extension like *.txt
and stores them in the $textFiles
variable.
The output of the above PowerShell script to retrieve text files using the wildcards in the PowerShell cmdlet is:
PS D:\> $files = Get-ChildItem -Path 'D:\PS\' PS D:\> $textFiles = $files | Where-Object {$_.name -like "*.txt"} PS D:\> $textFiles
Directory: D:\PS
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 26-02-2023 16:10 87 comp.txt
-a---- 02-04-2023 19:32 0 emptyFile.txt
PS D:\>
Use ? Wildcard with Variable in PowerShell
?
Wildcard in PowerShell finds the patterns that match exactly one character.
Get-ChildItem -Path 'D:\PS\' ??.* -File
In the above PowerShell script, the Get-ChildItem cmdlet uses the Path
parameter to specify the directory name to find the file name that has only 2 characters in them.
The output of the above PowerShell script is:
PS D:\> Get-ChildItem -Path 'D:\PS\' ??.* -File
Directory: D:\PS
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 07-04-2023 12:10 0 ad.txt
Filter Files based on Name Patterns
In PowerShell wildcards * can be used with -like
operator to filter files in a directory based on a name pattern.
$FilePattern = "powershell*" $imageFiles = Get-ChildItem -Path 'D:\PS\' | Where-Object {$_.Name -like $FilePattern}
In the above PowerShell script, the Get-ChildItem uses the Path
variable to get all the items and pipe them to the Where-Object cmdlet to retrieve the files based on name patterns.
The output of the above PowerShell script is:
PS D:\> $FilePattern = "powershell*" PS D:\> $imageFiles = Get-ChildItem -Path 'D:\PS\' | Where-Object {$_.Name -like $FilePattern} PS D:\> $imageFiles
Directory: D:\PS
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 02-04-2023 20:51 15400 powershell-clear-variable.png
-a---- 07-04-2023 09:47 17358 powershell-convert-variables-to-string.png
-a---- 02-04-2023 19:37 7396 powershell-create-empty-file.png
-a---- 02-04-2023 17:37 10133 powershell-get-temp-folder.png
-a---- 07-04-2023 08:31 12163 powershell-is-null-or-empty-check-for-variable.png
-a---- 02-04-2023 22:04 19830 powershell-read-only-variable.png
-a---- 02-04-2023 18:10 10850 powershell-temporary-file.png
-a---- 07-04-2023 11:58 7162 powershell-variable-wildcards.png
PS D:\>
Cool Tip: How to use a variable in Path in PowerShell!
Find Processes by Name Patterns
Wildcard * can be used in PowerShell to find processes running on a system based on name patterns.
$processPattern = "chrome*" $services = Get-Process | Where-Object {$_.ProcessName -like $processPattern} $services
Conclusion
I hope the above article on how to use PowerShell variables wildcards with * and ? is helpful to you.
Wildcards enable you to create and write flexible search patterns and improve script efficiency.
You can find more topics about PowerShell Active Directory commands and PowerShell basics on the ShellGeek home page.